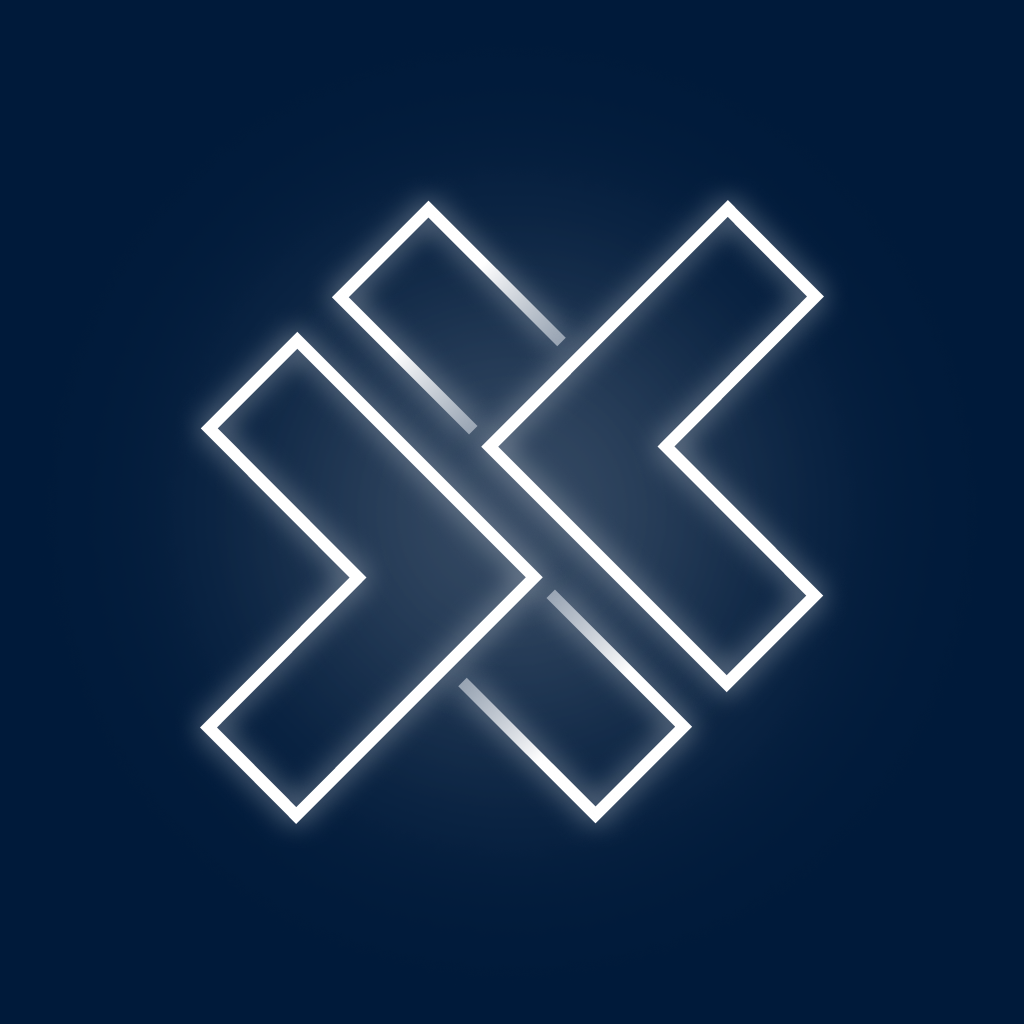
Native Audio
@capacitor-community/native-audio
Capacitor community plugin for playing sounds.
Capacitor Native Audio Plugin
Capacitor plugin for native audio engine.
Capacitor v3 - ✅ Support!
Click on video to see example 💥
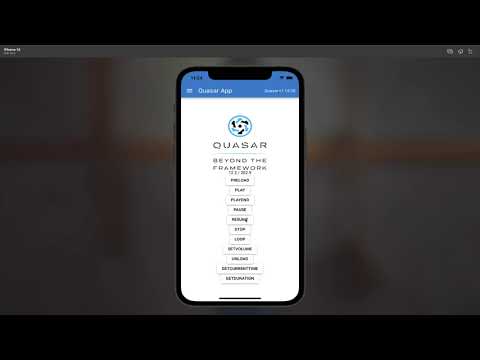
Maintainers
Mainteinance Status: Actively Maintained
Preparation
All audio place in specific platform folder
Andoid: android/app/src/assets
iOS: ios/App/App/sounds
Installation
To use npm
npm install @capacitor-community/native-audio
To use yarn
yarn add @capacitor-community/native-audio
Sync native files
npx cap sync
On iOS and Android, no further steps are needed.
Configuration
No configuration required for this plugin.
Supported methods
Name | Android | iOS | Web |
---|
configure | ✅ | ✅ | ❌ |
preloadSimple | ✅ | ✅ | ❌ |
preloadComplex | ✅ | ✅ | ❌ |
play | ✅ | ✅ | ❌ |
loop | ✅ | ✅ | ❌ |
stop | ✅ | ✅ | ❌ |
unload | ✅ | ✅ | ❌ |
setVolume | ✅ | ✅ | ❌ |
getDuration | ✅ | ✅ | ❌ |
getCurrentTime | ✅ | ✅ | ❌ |
Usage
Example repository
import {NativeAudio} from '@capacitor-community/native-audio'
NativeAudio.preload({
assetId: "fire",
assetPath: "fire.mp3",
audioChannelNum: 1,
isUrl: false
});
NativeAudio.play({
assetId: 'fire',
});
NativeAudio.loop({
assetId: 'fire',
});
NativeAudio.stop({
assetId: 'fire',
});
NativeAudio.unload({
assetId: 'fire',
});
NativeAudio.setVolume({
assetId: 'fire',
volume: 0.4,
});
NativeAudio.getDuration({
assetId: 'fire'
})
.then(result => {
console.log(result.duration);
})
NativeAudio.getCurrentTime({
assetId: 'fire'
});
.then(result => {
console.log(result.currentTime);
})
API
configure(...)
configure(options: ConfigureOptions) => Promise<void>
preload(...)
preload(options: PreloadOptions) => Promise<void>
play(...)
play(options: { assetId: string; time: number; }) => Promise<void>
Param | Type |
---|
options | { assetId: string; time: number; } |
resume(...)
resume(options: { assetId: string; }) => Promise<void>
Param | Type |
---|
options | { assetId: string; } |
loop(...)
loop(options: { assetId: string; }) => Promise<void>
Param | Type |
---|
options | { assetId: string; } |
stop(...)
stop(options: { assetId: string; }) => Promise<void>
Param | Type |
---|
options | { assetId: string; } |
unload(...)
unload(options: { assetId: string; }) => Promise<void>
Param | Type |
---|
options | { assetId: string; } |
setVolume(...)
setVolume(options: { assetId: string; volume: number; }) => Promise<void>
Param | Type |
---|
options | { assetId: string; volume: number; } |
getCurrentTime(...)
getCurrentTime(options: { assetId: string; }) => Promise<{ currentTime: number; }>
Param | Type |
---|
options | { assetId: string; } |
Returns: Promise<{ currentTime: number; }>
getDuration(...)
getDuration(options: { assetId: string; }) => Promise<{ duration: number; }>
Param | Type |
---|
options | { assetId: string; } |
Returns: Promise<{ duration: number; }>
Interfaces
ConfigureOptions
Prop | Type |
---|
fade | boolean |
focus | boolean |
PreloadOptions
Prop | Type |
---|
assetPath | string |
assetId | string |
volume | number |
audioChannelNum | number |
isUrl | boolean |