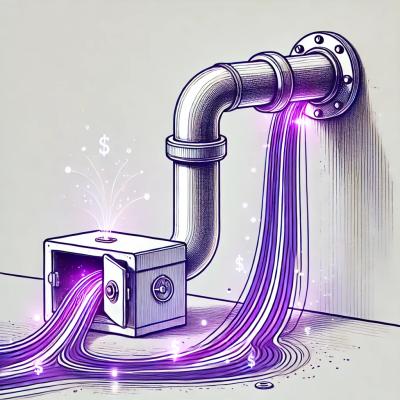
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
cdk-internal-gateway
Advanced tools
CDK construct to create to create internal serverless applications.
Use this CDK construct to create internal serverless applications.
Useful for larger companies to create internal serverless applications that are not exposed to the internet and only accessible from the internal network.
Using Typescript for aws cdk
npm i cdk-internal-gateway
Using Python for aws cdk
pip install pharindoko.cdk-internal-gateway
Modularized approach with separate constructs
Internal Service Construct (mandatory construct):
Internal Api Gateway Construct:
Internal Website Construct:
Let`s assume we create a simple internal api for our company and start with a single lambda function...
Create a file called /lib/my-new-stack.ts
import { aws_apigateway as apigateway, aws_ec2 as ec2, aws_lambda as lambda, aws_route53 as route53, Stack, StackProps } from 'aws-cdk-lib';
import { HttpMethod } from 'aws-cdk-lib/aws-events';
import { InternalApiGateway, InternalApiGatewayProps, InternalService } from 'cdk-internal-gateway';
import { Construct } from 'constructs';
import * as path from 'path';
// Create a new stack that inherits from the InternalApiGateway Construct
export class ServerlessStack extends InternalApiGateway {
constructor(scope: Construct, id: string, props: InternalApiGatewayProps) {
super(scope, id, props);
// The internal api gateway is available as member variable
// Attach your lambda function to the this.apiGateway
const defaultLambdaJavascript = this.apiGateway.root.resourceForPath("hey-js");
const defaultHandlerJavascript = new lambda.Function(
this,
`backendLambdaJavascript`,
{
functionName: `js-lambda`,
runtime: lambda.Runtime.NODEJS_14_X,
handler: "index.handler",
code: lambda.Code.fromAsset(path.join(__dirname, "../src")),
}
);
defaultLambdaJavascript.addMethod(
HttpMethod.GET,
new apigateway.LambdaIntegration(defaultHandlerJavascript)
);
}
}
// Create a new stack that contains the whole service with all nested stacks
export class ServiceStack extends Stack {
constructor(scope: Construct, id: string, props: StackProps) {
super(scope, id, props);
// get all parameters to create the internal service stack
const vpc = ec2.Vpc.fromLookup(this, 'vpcLookup', { vpcId: 'vpc-1234567890' });
const subnetSelection = {
subnets: ['subnet-0b1e1c6c7d8e9f0a2', 'subnet-0b1e1c6c7d8e9f0a3'].map((ip, index) =>
ec2.Subnet.fromSubnetId(this, `Subnet${index}`, ip),
),
};
const hostedZone = route53.HostedZone.fromLookup(this, 'hostedzone', {
domainName: 'test.aws1234.com',
privateZone: true,
vpcId: vpc.vpcId,
});
const vpcEndpoint =
ec2.InterfaceVpcEndpoint.fromInterfaceVpcEndpointAttributes(
this,
'vpcEndpoint',
{
port: 443,
vpcEndpointId: 'vpce-1234567890',
},
);
// create the internal service stack
const serviceStack = new InternalService(this, 'InternalServiceStack', {
hostedZone: hostedZone,
subnetSelection: subnetSelection,
vpcEndpointIPAddresses: ['192.168.2.1', '192.168.2.2'],
vpc: vpc,
subjectAlternativeNames: ['internal.example.com'],
subDomain: "internal-service"
})
// create your stack that inherits from the InternalApiGateway
new ServerlessStack(this, 'MyProjectStack', {
domains: serviceStack.domains,
stage: "dev",
vpcEndpoint: vpcEndpoint,
})
// create another stack that inherits from the InternalApiGateway
...
...
}
}
Reference the newly created ServiceStack
in the default /bin/{project}.ts
file e.g. like this
new ServiceStack(app, 'MyProjectStack', {
env:
{
account: process.env.CDK_DEPLOY_ACCOUNT || process.env.CDK_DEFAULT_ACCOUNT,
region: process.env.CDK_DEPLOY_REGION || process.env.CDK_DEFAULT_REGION
}
You have to expect basic infra costs for 2 components in this setup:
Count | Type | Estimated Costs |
---|---|---|
1 x | application load balancer | 20 $ |
2 x | network interfaces for the vpc endpoint | 16 $ |
A shared vpc can lower the costs as vpc endpoint and their network interfaces can be used together...
FAQs
CDK construct to create to create internal serverless applications.
The npm package cdk-internal-gateway receives a total of 132 weekly downloads. As such, cdk-internal-gateway popularity was classified as not popular.
We found that cdk-internal-gateway demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.