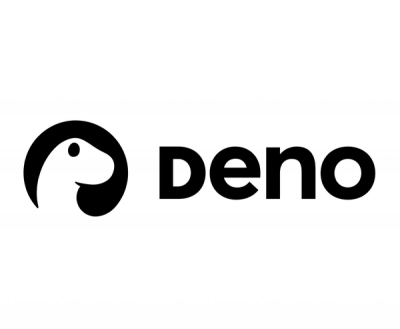
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
cf-service-metrics-logger
Advanced tools
Install library by using npm
npm install cf-service-metrics-logger
or by using yarn
yarn add cf-service-metrics-logger
Create a new instance of CfServiceMetricsLogger and pass any kind of logger implementing following interface:
{} | [] | string | number
){} | [] | string | number
){} | [] | string | number
){} | [] | string | number
)/* import library by using CommonJS module loader */
const CfServiceMetricsLogger = require('cf-service-metrics-logger');
/* or import library by using ES6 module loader */
import { CfServiceMetricsLogger } from 'cf-service-metrics-logger';
/* create logger instance or use a logger library */
class Logger {
debug(message) {
console.debug(message);
}
info(message) {
console.info(message);
}
warn(message) {
console.warn(message);
}
error(message) {
console.error(message);
}
}
const logger = new Logger();
/* you can add some options to CfServiceMetricsLogger */
const options = {
mongoDB: {
serverStatusInterval: 10000, //optional
bStatsInterval: 20000, //optional
},
redis: {
infoInterval: 100000 //optional
},
vcap: {}; //optional
vcapFile: ''; //optional
};
/* create new instance of CfServiceMetricsLogger and pass logger instance and optional options */
const cfServiceMetricsLogger = new CfServiceMetricsLogger(logger, options);
/* start service metrics logging */
cfServiceMetricsLogger.start();
/* stop service metrics logging */
cfServiceMetricsLogger.stop();
You need to set up your development environment before you can do anything.
Install Node.js and NPM
brew install node
choco install nodejs
Install yarn globally
yarn install yarn -g
Copy the vcap.example.json
file and rename it to vcap.json
. This file provides VCAP_SERVICES
and/or VCAP_APPLICATION
for local development.
More information is provided here.
Install all dependencies with yarn.
yarn install
yarn install
yarn run lint
. This runs tslint.yarn run test
.yarn run build
to generate commonJS and ES6 modules as well as declaration from the TypeScript source.dist
folder.Just set a breakpoint in source or unit test and hit F5 in your Visual Studio Code to execute and debug all unit tests.
Name | Description |
---|---|
.vscode/ | VSCode tasks, launch configuration and some other settings |
dist/ | Compiled and bundled source files will be placed here |
src/ | Source files |
src/types/ *.d.ts | Custom type definitions and files that aren't on DefinitelyTyped |
test/ | Tests |
test/unit/ *.test.ts | Unit tests |
vcap.example.json | Provides VCAP_SERVICES and/or VCAP_APPLICATION for local development |
vcap.test.json | Provides VCAP_SERVICES and/or VCAP_APPLICATION for unit tests |
rollup.config.js | Config for Rollup module bundler |
[0.1.0] - 2019-01-29
FAQs
Log Cloud Foundry service metrics
The npm package cf-service-metrics-logger receives a total of 1 weekly downloads. As such, cf-service-metrics-logger popularity was classified as not popular.
We found that cf-service-metrics-logger demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.