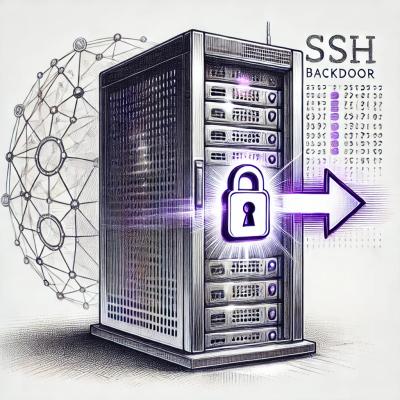
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
The cfonts npm package is used to generate ASCII art text with various fonts and styles in the terminal. It allows for customization of font, color, background, and alignment, making it useful for creating visually appealing text outputs in command-line applications.
Basic Text Rendering
This feature allows you to render basic text in the terminal using a specified font and alignment. The example code renders 'Hello, World!' using the 'block' font, left-aligned, and with system colors.
const cfonts = require('cfonts');
cfonts.say('Hello, World!', { font: 'block', align: 'left', colors: ['system'] });
Custom Colors
This feature allows you to customize the colors of the text. The example code renders 'Colorful Text' using the 'console' font, center-aligned, and with a gradient of red, yellow, and blue colors.
const cfonts = require('cfonts');
cfonts.say('Colorful Text', { font: 'console', align: 'center', colors: ['red', 'yellow', 'blue'] });
Background Colors
This feature allows you to set a background color for the text. The example code renders 'Background Color' using the 'chrome' font, right-aligned, with a black background and white text color.
const cfonts = require('cfonts');
cfonts.say('Background Color', { font: 'chrome', align: 'right', background: 'black', colors: ['white'] });
Multiple Lines
This feature allows you to render multiple lines of text. The example code renders three lines of text using the 'simple' font, center-aligned, and with cyan color.
const cfonts = require('cfonts');
cfonts.say('Line 1\nLine 2\nLine 3', { font: 'simple', align: 'center', colors: ['cyan'] });
Figlet is a package that generates text banners in various fonts. It is similar to cfonts in that it allows for ASCII art text generation, but it has fewer customization options for colors and backgrounds.
Ascii-art is a package that provides a wide range of ASCII art functionalities, including text, images, and tables. It offers more comprehensive ASCII art features compared to cfonts but may be more complex to use for simple text rendering.
Chalk is a package that allows you to style terminal strings with colors and other attributes. While it does not generate ASCII art text, it can be used in conjunction with other packages like figlet to add color and style to text outputs.
██████╗ ███████╗ ██████╗ ███╗ ██╗ ████████╗ ███████╗
██╔════╝ ██╔════╝ ██╔═══██╗ ████╗ ██║ ╚══██╔══╝ ██╔════╝
██║ █████╗ ██║ ██║ ██╔██╗ ██║ ██║ ███████╗
██║ ██╔══╝ ██║ ██║ ██║╚██╗██║ ██║ ╚════██║ ╔╗╔ ╔═╗ ╔╦╗ ╔═╗ ╦ ╔═╗
╚██████╗ ██║ ╚██████╔╝ ██║ ╚████║ ██║ ███████║ ║║║ ║ ║ ║║ ║╣ ║ ╚═╗
╚═════╝ ╚═╝ ╚═════╝ ╚═╝ ╚═══╝ ╚═╝ ╚══════╝ ╝╚╝ ╚═╝ ═╩╝ ╚═╝ ╚╝ ╚═╝
This is a silly little command line tool for sexy ANSI fonts in the console. Give your cli some love.
npm i cfonts -g
yarn global add cfonts
To use it in your shell:
$ cfonts "Hello|World\!"
💡 Remember to escape the !
character with \
in the shell
Or use it in your project:
const cfonts = require('cfonts');
cfonts.say('Hello|world!', {
font: 'block', // define the font face
align: 'left', // define text alignment
colors: ['system'], // define all colors
background: 'transparent', // define the background color, you can also use `backgroundColor` here as key
letterSpacing: 1, // define letter spacing
lineHeight: 1, // define the line height
space: true, // define if the output text should have empty lines on top and on the bottom
maxLength: '0', // define how many character can be on one line
gradient: false, // define your two gradient colors
independentGradient: false, // define if you want to recalculate the gradient for each new line
transitionGradient: false, // define if this is a transition between colors directly
rawMode: false, // define if the line breaks should be CRLF (`\r\n`) over the default LF (`\n`)
env: 'node' // define the environment cfonts is being executed in
});
All settings are optional and shown here with their default
You can use cfonts
in your project without the direct output to the console:
const cfonts = require('cfonts');
const prettyFont = cfonts.render('Hello|world!', {/* same settings object as above */});
prettyFont.string // the ansi string for sexy console font
prettyFont.array // returns the array for the output
prettyFont.lines // returns the lines used
prettyFont.options // returns the options used
Read more in the root repo.
This package is tested on the below platform and node combinations as part of our CI.
Platform | Node |
---|---|
Linux | v12 |
Linux | v14 |
Linux | v16 |
Linux | v18 |
Linux | v20 |
Linux | v22 |
MacOS | v16 |
MacOS | v18 |
MacOS | v20 |
MacOS | v22 |
Windows | v12 |
Windows | v14 |
Windows | v16 |
Windows | v18 |
Windows | v20 |
Windows | v22 |
The package comes with a bunch of unit tests that aim to cover 100% of the code base. For more details about the code coverage check out coveralls.
npm run test:unit
Since the code base uses JSDocs we use typescript to test the inferred types from those comments. Typescript supports JSDocs and we use it in our test.
npm run test:types
There is also a test suite for font files.
npm run test:fonts
This tool checks:
Run all tests via:
npm run test
To build the repo install dependencies via:
(Since we ship a yarn.lock
file please use yarn
for development.)
yarn
and run the watch to continuously transpile the code.
yarn watch
Please look at the coding style and work with it, not against it ;)
gray
to gradient colorstop
and bottom
align optionstiny
slick
, grid
and pallet
, added double quote to all fontsmaxLength
, gradient
and lineHeight
, added more end-to-end testsansi-styles
from direct dependencieschange-case
dependency, added UpperCaseFirst
with testsshade
, added hex color supportcfonts
with pure functions, made colors case-insensitivetransparent
and system
as default background and color option, added backgroundColor
as alias for background
, upgraded depsbabel-polyfill
to babel-plugin-transform-runtime
, added files to package.json, added images to docs, fixed dependenciesconsole
font, added comma, added font huge
, added render method, added candy colorchrome
font, fonttestsimpleBlock
simple3d
simple3d
and added to grunt testsimple3d
fontconsole
font3d
fontsimple
fontsimple
fontCopyright (c) 2022 Dominik Wilkowski. Licensed under the GNU GPL-3.0-or-later.
FAQs
Sexy ANSI fonts for the console
We found that cfonts demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.