What is chance?
The Chance npm package is a minimalist generator of random strings, numbers, and other utilities. It is designed to help developers create random data for testing, simulations, and more. The package offers a wide range of functionalities, from generating random names, addresses, and phone numbers to creating random numbers, characters, and even custom random generators.
What are chance's main functionalities?
Basic Random Data Generation
This feature demonstrates how to generate basic random data such as names and integers. The 'name' method generates a random full name, while the 'integer' method generates a random integer within a specified range.
const Chance = require('chance');
const chance = new Chance();
// Generate a random name
console.log(chance.name());
// Generate a random integer
console.log(chance.integer({min: 0, max: 100}));
Advanced Random Data Generation
This feature showcases the generation of more complex data types like addresses and geo-coordinates. The 'address' method returns a random address, and the 'coordinates' method returns a random latitude and longitude pair.
const Chance = require('chance');
const chance = new Chance();
// Generate a random address
console.log(chance.address());
// Generate a random geo-coordinate
console.log(chance.coordinates());
Custom Random Data Generation
This feature illustrates how to create custom random data generators using Chance. In this example, a simple dice roll function is created that returns a random integer between 1 and 6.
const Chance = require('chance');
const chance = new Chance();
// Custom random generator for a dice roll
function diceRoll() {
return chance.integer({min: 1, max: 6});
}
console.log(diceRoll());
Other packages similar to chance
faker
Faker is a popular alternative to Chance that focuses on generating massive amounts of fake data, especially for testing purposes. It supports a wide range of data types similar to Chance but also includes locales for generating data in specific languages and formats.
random-js
Random-js is a mathematically correct random number generator library for JavaScript. It offers a lower-level approach compared to Chance, providing tools to generate random values using various distributions. It's more suited for simulations and games that require specific random behavior.
Chance
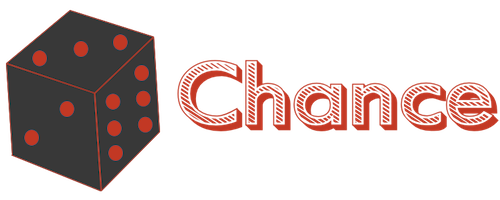

Chance - Random generator helper for JavaScript
Homepage: http://chancejs.com
Many more details on http://chancejs.com but this single
library can generate random numbers, characters, strings, names, addresses,
dice, and pretty much anything else.
It includes the basic building blocks for all these items and is built on top
of a Mersenne Twister so it can generate these things with repeatability, if
desired.
Usage
See the full docs for details on installation and usage.
Dependent tools
Or view all of the dependents on npm
Know a library that uses Chance that isn't here? Update the README and submit a PR!
Author
Victor Quinn
https://www.victorquinn.com
@victorquinn
Please feel free to reach out to me if you have any questions or suggestions.
Contributors
THANK YOU!
Contribute!
Be a part of this project! You can run the test using the following.
- Install dependencies from package.json by running
npm install
- Run the test via
npm test
- Make some fun new modules!
This project is licensed under the MIT License so feel free to hack away :)
Proudly written in Washington, D.C.