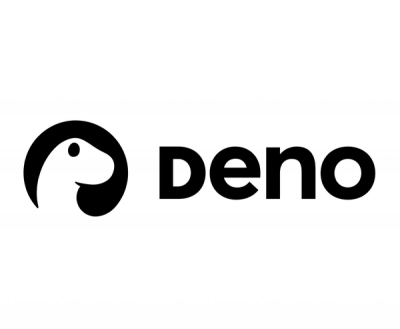
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
chat
🍹yarn add chat
Build production-ready, full-stack chat in minutes. Drop in our pre-made UI components, connect them to your backend our pre-made logic, and you're good to go.
Stop worrying about:
✅ Auth
✅ Customizable UI
✅ Transitions & animations
✅ Websockets, data & fallbacks
✅ Offline support (beta)
✅ 1-on-1 and group chats
import * as React
import { useMessages, Messages } from 'usechat'
const ChatScreen = () => {
const { data, send } = useMessages({ id: 'my-first-chat-room' })
return <Messages onSend={send} messages={data} />
}
If you only want to use this library for the UI components, there is no further setup required.
However, if you want to connect your firebase/firestore backend, there is are two added steps.
1. Install dependencies
yarn add firebase @nandorojo/fuego
2. Add the provider to the root of your app
Initialize fuego
's db first. Then, wrap your app with the ChatProvider
.
// App.tsx
import * as React from 'react'
import { ChatProvider } from 'chat'
import { Fuego } from '@nandorojo/fuego'
const fuego = new Fuego({
//...firebase config here
})
export default () => {
return <ChatProvider fuego={fuego}>{/* Your app goes here*/}</ChatProvider>
}
How to find your firebase config
Note: Make sure you've enabled firebase firestore to use this. See this tutorial for more.
This is all it takes to build a full-stack live chat with chat
in React/React Native (with some abstraction).
import * as React
import { useNavigation, useNavigationParam } from 'react-navigation'
import { useInbox, Inbox, useMessages, Messages } from 'usechat'
const InboxScreen = () => {
const { data } = useInbox()
const navigation = useNavigation()
return <Inbox rooms={data} onPressItem={id => navigation.navigate('Chat', { id })} >
}
const ChatScreen = () => {
const id = useNavigationParam('id')
const { data, send } = useMessages({ id })
return <Messages onSend={send} messages={data} />
}
😎 Works with react
and react native
(including Expo!)
🚨 Integrates with Firebase auth (and can also work with your custom auth solutions)
📣 Integrates with expo-notifications
🥐 Fully customizable UI components
🍇 Production-level animations, transitions, and swipe gestures.
👾 Written in TypeScript
Setup is quick and easy. Jump to the setup guide. Then, check out the hello world and example.
✏️ Typing indicators
💯 Unread count
😂 Meme search (powered by Memezy)
👻 Image & video, including uploading logic & content storage
🍪 Online status
🕺 Realtime inbox
🤯 Realtime chat rooms
😇 Room-level user permissions
🔥 Group chats
👴 Customizable system messages (ex: William Buzzelbees changed the group name to "Frankenstein's Friday Squad")
👀 Send a message to multiple people, even if a room with those people doesn't exist yet (like an instagram feed-to-DM flow.)
🥇 Room names (coming soon)
🧤 <AuthGate />
wraps your app and gives it customizable auth, powered by firebase.
🧢 <Inbox />
gives you a real-time list of chat rooms.
🎩 <InboxItem />
List item for <Inbox />
, including customizable swipe gestures.
⛑ <TypingIndicator />
Show typing, with animations, as it happens
🍪 <UnreadIndicator />
Show an iMessage-like unread indicator, with or without the number of unread messages.
⚡️ <Messages />
Like GiftedChat, to the max.
Functions are pre-made for the following:
🐻 Create chat room
🎃 Leave chat room
🤘 Remove users from chat room
👮♂️ Customizable avatars for chat rooms
🏄🏾♂️ Add people to a chat room
Chat will automatically integrate into your Firebase auth if you're using it once you've completed the setup.
Yes, there are. The motivation for this library comes down to only one concept: production-ready chat.
If you google react native chat tutorial
, you'll come up with many smart people writing good articles about building your first chat app. Suggestions range from using firebase's highly-customizable serverless database to more opinionated startups that build SDKs for chat only.
The thing is, none of these options created a production-ready chat that "thinks in React."
With a custom option like Firebase, you're having to deal with tons of different socket subscriptions, chat schema changes, etc, and building in features like unread count, online status, or typing indicators becomes non-trivial quickly.
Meanwhile, existing JavaScript chat SDKs aren't made with React's stateful nature in mind. Companies like Pusher, PubNub, and Stream clearly have something good going for them, but they aren't made for React the way a library like Apollo
is.
You should be able to build a customizable, full-stack chat with only a few lines of code. If Stripe can do that for accepting payments, we can do it for sending words.
Tutorials for existing chat SDKs have you typing setState
dozens of times, and you wonder, would this actually look as good as iMessage in production? What if I want to move my component files around? What if I forget to unmount a listener on a certain screen? And if I want to reorganize my component files, do I have to write all that bulky setState
logic over? What happens if I want to use a state manager like redux?
With all those questions in mind, I decided I would go feature-by-feature, headache-by-headache, and make my own chat SDK that was made specifically for react
/react-native
(and expo
in particluar).
Thanks to the introduction of react hooks
in React 16.8.3 (and in expo
after SDK v33 🔥), making a react-first chat SDK went from a massive headache to an elegant, scalable experience.
This chat library relies on two main APIs: firebase firestore
for the database, react hooks
for stateful component logic.
That means that you can build a serverless chat app that is actually ready for production. And when you need extra customizations like unread counts and typing indicators, you just click that you want them in a GUI.
A final point: most chat SDKs own your messages and data. With us, we just link into your firebase, so you own all the data.
FAQs
Unknown package
The npm package chat receives a total of 109 weekly downloads. As such, chat popularity was classified as not popular.
We found that chat demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.