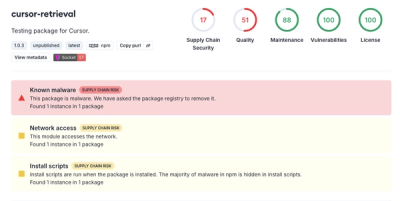
Security News
The Risks of Misguided Research in Supply Chain Security
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
'chatux' is a library that allows you to easily create chat windows on your PC or mobile
'chatux' is a library that allows you to easily create chat windows on your PC or mobile
It is licensed under MIT license.
It is an independent and lightweight chat user interface (chat UI) library for javascript.
https://riversun.github.io/chatux/
How to play demo.
This demo is simple echo chat. But a few command available.If you write "show buttons", you can see action buttons in chat. Or you write "show image", you can see image in chat.
Check this example.
<!DOCTYPE html>
<html lang="en">
<head>
<title>chatux example</title>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, maximum-scale=1">
</head>
<body style="padding:0px;margin:0px;">
<div style="padding:40px">
<h1>chatux example</h1>
<p> Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.</p>
</div>
<script src="https://riversun.github.io/chatux/chatux.min.js"></script>
<script>
const chatux = new ChatUx();
const opt = {
api: {
//URL of chat server
endpoint: 'https://script.google.com/macros/s/AKfycbwpf8iZfGXkJD6K__oCVQYF35HLBQjYxmKP0Ifrpe_piK4By4rh/exec',
//HTTP METHOD
method: 'GET',
//DATA TYPE
dataType: 'jsonp'
},
window: {
title: 'My chat',
infoUrl: 'https://github.com/riversun/chatux'
}
};
//initialize
chatux.init(opt);
chatux.start(true);
</script>
</body>
</html>
Show on PC
Show on mobile
install
npm install chatux --save
code
import {ChatUx} from 'chatux';
const chatux = new ChatUx();
chatux.init({
api: {
endpoint: 'http://localhost:8080/chat',//chat server
method: 'GET',//HTTP METHOD when requesting chat server
dataType: 'json'//json or jsonp is available
}
});
chatux.start();
<script src="https://riversun.github.io/chatux/chatux.min.js"></script>
code
const chatux = new ChatUx();
chatux.init({
api: {
endpoint: 'http://localhost:8080/chat',//chat server
method: 'GET',//HTTP METHOD when requesting chat server
dataType: 'json'//json or jsonp is available
}
});
chatux.start();
The system of chatux is very simple.
Let's look at the execution sequence of chatux.
Suppose you have a chat server for chatux at http://localhost:8080/chat Specify server endpoint like this.
chatux.init({
api: {
endpoint: 'http://localhost:8080/chat',
method: 'GET',
dataType: 'json'
}
});
The following is the sequence.
1. chatux sends user input text to chat server.
GET http://localhost:8080/chat?text=hello
2. The server processes user input text and returns a response as JSON.
{
"output": [
{
"type": "text",
"value": "You say 'hello'"
}
]
}
So, if you create chat server that can do this kind of interaction, you can easily create chatbots etc.
Next, let's see how to render.
Rendering is indicated by simple JSON generated by chat server.
JSON
{
"output": [
{
"type": "text",
"value": "Hello world!"
}
]
}
RESULT
JSON
{
"output": [
{
"type": "image",
"value": "https://avatars1.githubusercontent.com/u/11747460"
}
]
}
RESULT
JSON
{
"output": [
{
"type": "option",
"options": [
{
"label": "label1",
"value": "value1"
},
{
"label": "label2",
"value": "value2"
},
{
"label": "label3",
"value": "value3"
}
]
}
]
}
RESULT
{
"output": [
{
"type": "html",
"value": "Click <a href=\"https://github.com/riversun\" target=\"_blank\" >here</a> to open a page.",
"delayMs": 500
}
]
}
JSON
{
"output": [
{
"type": "youtube",
"value": "TP4lxliMHXY", //youtube video id
"delayMs": 500
}
]
}
RESULT
JSON
{
"output": [
{
"type": "text",
"value": "What is this?",
"delayMs": 500
},
{
"type": "image",
"value": " https://upload.wikimedia.org/wikipedia/commons/a/a3/Aptenodytes_forsteri_-Snow_Hill_Island%2C_Antarctica_-adults_and_juvenile-8.jpg"
},
{
"type": "option",
"options": [
{
"label": "bob",
"value": "value1"
},
{
"label": "riversun",
"value": "value2"
},
{
"label": "john",
"value": "value3"
}
]
}
]
}
RESULT
JSON
{
"output": [
{
"type": "window",
"title": "iframe page",
"url": "https://riversun.github.io/i18nice",
"left": 20,
"top": 20,
"width": 400,
"height": 250,
"addYOffset": true,
}
]
}
RESULT
JSON
{
"output": [
{
"type": "window",
"title": "youtube movie",
"html": "<div style=\"position: relative; width: 100%; padding-top: 56.25%;background:black;overflow: hidden\"><iframe style=\"position: absolute;top: 0;right: 0;width: 100% !important;height: 100% !important;\" width=\"400\" height=\"300\" src=\"https://www.youtube.com/embed/nepdFs-2V1Y\" frameborder=\"0\" allow=\"accelerometer; autoplay; encrypted-media; gyroscope; picture-in-picture\" allowfullscreen></iframe></div>",
"mobileUrl": "https://www.youtube.com/embed/nepdFs-2V1Y",
"left": 60,
"top": 60,
"width": 400,
"height": 250,
"addYOffset": true,
"overflow": "hidden",
"backgroundColor": "black",
"delayMs": 10
}
]
}
RESULT
JSON
{
"output": [
{
"type": "window",
"title": "google drive movie",
"html": "<div style=\"position: relative; width: 100%; padding-top: 56.25%;background:black;overflow: hidden\"><iframe style=\"position: absolute;top: 0;right: 0;width: 100% !important;height: 100% !important;\" src=\"https://drive.google.com/file/d/something_id/preview\" width=\"400\" height=\"300\"></iframe></div>",
"mobileUrl": "https://drive.google.com/file/d/something_id/preview",
"left": 100,
"top": 100,
"width": 400,
"height": 250,
"addYOffset": true,
"overflow": "hidden",
"backgroundColor": "black",
"delayMs": 10
}
]
}
RESULT
FAQs
'chatux' is a library that allows you to easily create chat windows on your PC or mobile
The npm package chatux receives a total of 34 weekly downloads. As such, chatux popularity was classified as not popular.
We found that chatux demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.