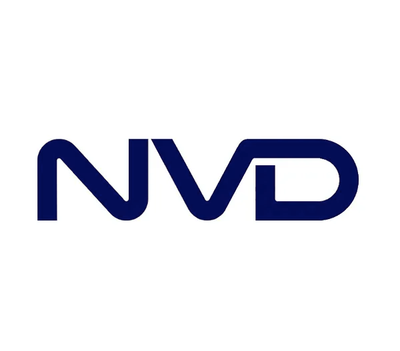
Security News
NIST Misses 2024 Deadline to Clear NVD Backlog
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.
child-process-promise
Advanced tools
Simple wrapper around the "child_process" module that makes use of promises
The 'child-process-promise' npm package is a utility that provides a promise-based interface for Node.js's child_process module. It allows you to execute shell commands and spawn new processes with the added benefit of using promises for better asynchronous control.
exec
The 'exec' function allows you to run a shell command and returns a promise that resolves with the command's output. This is useful for running simple shell commands and capturing their output.
const { exec } = require('child-process-promise');
exec('ls -la')
.then(result => {
console.log('stdout:', result.stdout);
console.log('stderr:', result.stderr);
})
.catch(err => {
console.error('ERROR:', err);
});
spawn
The 'spawn' function allows you to start a new process using a given command and arguments. It returns a promise that resolves when the process completes. This is useful for more complex scenarios where you need to interact with the process's input/output streams.
const { spawn } = require('child-process-promise');
const child = spawn('ls', ['-la']);
child.childProcess.stdout.on('data', data => {
console.log('stdout:', data.toString());
});
child.childProcess.stderr.on('data', data => {
console.error('stderr:', data.toString());
});
child.then(() => {
console.log('Process completed');
}).catch(err => {
console.error('ERROR:', err);
});
Execa is a modern alternative to child_process that provides a promise-based interface for executing shell commands. It offers more features and better defaults compared to child-process-promise, such as improved error handling and support for streaming output.
Promisify-child-process is another package that wraps Node.js's child_process module with promises. It provides a similar interface to child-process-promise but focuses on being a lightweight and minimalistic solution.
Node-cmd is a simple package for executing shell commands in Node.js. It provides a callback-based interface but can be easily used with promises using utilities like util.promisify. It is less feature-rich compared to child-process-promise but can be a good choice for simpler use cases.
Simple wrapper around the child_process
module that makes use of promises
npm install child-process-promise --save
var exec = require('child-process-promise').exec;
exec('echo hello')
.then(function (result) {
var stdout = result.stdout;
var stderr = result.stderr;
console.log('stdout: ', stdout);
console.log('stderr: ', stderr);
})
.catch(function (err) {
console.error('ERROR: ', err);
});
var spawn = require('child-process-promise').spawn;
var promise = spawn('echo', ['hello']);
var childProcess = promise.childProcess;
console.log('[spawn] childProcess.pid: ', childProcess.pid);
childProcess.stdout.on('data', function (data) {
console.log('[spawn] stdout: ', data.toString());
});
childProcess.stderr.on('data', function (data) {
console.log('[spawn] stderr: ', data.toString());
});
promise.then(function () {
console.log('[spawn] done!');
})
.catch(function (err) {
console.error('[spawn] ERROR: ', err);
});
Type: Array
Default: []
Pass an additional capture
option to buffer the result of stdout
and/or stderr
var spawn = require('child-process-promise').spawn;
spawn('echo', ['hello'], { capture: [ 'stdout', 'stderr' ]})
.then(function (result) {
console.log('[spawn] stdout: ', result.stdout.toString());
})
.catch(function (err) {
console.error('[spawn] stderr: ', err.stderr);
});
FAQs
Simple wrapper around the "child_process" module that makes use of promises
The npm package child-process-promise receives a total of 357,021 weekly downloads. As such, child-process-promise popularity was classified as popular.
We found that child-process-promise demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.