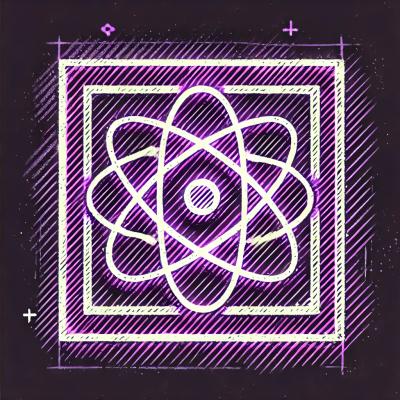
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
choo-lazy-view
Advanced tools
Lazily load views as the router invokes them. Works great with dynamic import or split-require but should work with any software with a promise or callback interface.
var splitRequire = require('split-require')
var LazyView = require('choo-lazy-view')
var html = require('choo/html')
var choo = require('choo')
var app = choo()
app.route('/', main)
// using dynamic import
app.route('/some-page', LazyView.create(() => import('./a')))
// or using split-require
app.route('/another-page', LazyView.create((cb) => splitRequire('./b', cb)))
module.exports = LazyView.mount(app, 'body')
function main () {
return html`
<body class="Home">
<h1>home</h1>
<a href="/a">a</a><br>
<a href="/b">b</a>
</body>
`
}
The module exposes a Nanocomponent class with two static
methods which are used for wrapping your views and choo.mount
.
LazyView.create(callback, loader?)
Accepts a callback and an optional loader view. The callback will be invoked
when the returned function is called upon by the router. The callback, in turn,
should load the required view and relay it's response (or error) back to the
caller. This can be done either with a Promise
or with the supplied callback.
// using promise
app.route('/my-page', LazyView.create(function () {
return fetchViewPromise()
}))
// using the callback
app.route('/another-page', LazyView.create(function (callback) {
fetchViewCallback(callback)
}))
The second argument is optional and should be a function or a DOM node which will be displayed while loading. By default, the node used to mount the application in the DOM is used as loader (meaning the view remains unchanged while loading).
app.route('/a', LazyView.create(
() => import('./my-view'),
(state, emit) => html`<body>Loading view…</body>`
))
LazyView.mount(app, selector)
Wrapper function for app.mount
which stores the selector internally to use as
fallback loader while fetching views.
- module.exports = app.mount('body')
+ module.exports = LazyView.mount(app, 'body')
You may extend on the LazyView component to add a shared framework wrapper, e.g. a header and footer.
// components/view/index.js
var html = require('choo/html')
var LazyView = require('choo-lazy-view')
var Header = require('../header')
var Footer = require('../footer')
module.exports = class View extends LazyView {
createElement (state, emit) {
return html`
<body>
${state.cache(Header, 'header').render()}
${super.createElement(state, emit)}
${state.cache(Footer, 'footer').render()}
</body>
`
}
}
// index.js
var choo = require('choo')
var View = require('./components/view')
var app = choo()
app.route('/', View.create(() => import('./views/home')))
module.exports = View.mount(app, 'body')
Events are namespaced under choo-lazy-view
and emitted when loading views.
choo-lazy-view:fetch
When fetching a view.
choo-lazy-view:done
When the view has been fetched and is about to rerender.
choo-lazy-view:error
When the view fails to load.
FAQs
Lazily fetch view when needed
We found that choo-lazy-view demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.