js-cid

CID implementation in JavaScript.
Lead Maintainer
Volker Mische
Table of Contents
Install
In Node.js through npm
$ npm install --save cids
Browser: Browserify, Webpack, other bundlers
The code published to npm that gets loaded on require is in fact an ES5 transpiled version with the right shims added. This means that you can require it and use with your favourite bundler without having to adjust asset management process.
const CID = require('cids')
In the Browser through <script>
tag
Loading this module through a script tag will make the Cids
obj available in the global namespace.
<script src="https://unpkg.com/cids/dist/index.min.js"></script>
<!-- OR -->
<script src="https://unpkg.com/cids/dist/index.js"></script>
Usage
You can create an instance from a CID string or CID Uint8Array
const CID = require('cids')
const cid = new CID('bafybeig6xv5nwphfmvcnektpnojts33jqcuam7bmye2pb54adnrtccjlsu')
cid.version
cid.codec
cid.code
cid.multibaseName
cid.toString()
or by specifying the cid version, multicodec name and multihash:
const CID = require('cids')
const multihashing = require('multihashing-async')
const bytes = new TextEncoder('utf8').encode('OMG!')
const hash = await multihashing(bytes, 'sha2-256')
const cid = new CID(1, 'dag-pb', hash)
console.log(cid.toString())
The multicodec integer code can also be used to create a new CID:
const cid = new CID(1, 112, hash)
console.log(cid.toString())
The string form of v1 CIDs defaults to base32
encoding (v0 CIDs are always base58btc
encoded). When creating a new instance you can optionally specify the default multibase to use when calling toBaseEncodedString()
or toString()
const cid = new CID(1, 'raw', hash, 'base64')
console.log(cid.toString())
If you construct an instance from a valid CID string, the base you provided will be preserved as the default.
const cid = new CID('uAXASIHJSUj5lkfuP5VPWf_VahvhARLRqPkF24QxY-lKaSqvV')
cid.toString()
API
CID.isCID(cid)
Returns true if object is a valid CID instance, false if not valid.
It's important to use this method rather than instanceof
checks in
order to handle CID objects from different versions of this module.
CID.validateCID(cid)
Validates the different components (version, codec, multihash, multibaseName) of the CID
instance. Throws an Error
if not valid.
new CID(version, codec, multihash, [multibaseName])
version
must be either 0 or 1.
codec
must be a string of a valid registered codec.
multihash
must be a Uint8Array
instance of a valid multihash.
multibaseName
optional string. Must be a valid multibase name. Default is base58btc
for v0 CIDs or base32
for v1 CIDs.
new CID(baseEncodedString)
Additionally, you can instantiate an instance from a base encoded
string.
new CID(Uint8Array)
Additionally, you can instantiate an instance from a Uint8Array
.
cid.codec
Property containing the string identifier of the codec.
cid.code
Property containing the integer identifier of the codec.
cid.version
Property containing the CID version integer.
cid.multihash
Property containing the multihash Uint8Array
.
cid.multibaseName
Property containing the default base to use when calling .toString
cid.bytes
Property containing the full CID encoded as a Uint8Array
.
cid.prefix
Proprety containing a Uint8Array
of the CID version, codec, and the prefix
section of the multihash.
cid.toV0()
Returns the CID encoded in version 0. Only works for dag-pb
codecs.
Throws if codec is not dag-pb
.
cid.toV1()
Returns the CID encoded in version 1.
cid.toBaseEncodedString(base=this.multibaseName)
Returns a base encoded string of the CID. Defaults to the base encoding in this.multibaseName
.
The value of this.multibaseName
depends on how the instance was constructed:
- If the CID was constructed from an object that already had a multibase (a string or an existing CID) then it retains that base.
- If the CID was constructed from an object that did not have a multibase (a
Uint8Array
, or by passing only version + codec + multihash to the constructor), then multibaseName
will be base58btc
for a v0 CID or base32
for a v1 CID.
cid.toString(base=this.multibaseName)
Shorthand for cid.toBaseEncodedString
described above.
cid.equals(cid)
Compare cid instance. Returns true if CID's are identical, false if
otherwise.
Contribute
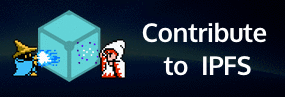
Contributions welcome. Please check out the issues.
Check out our contributing document for more information on how we work, and about contributing in general. Please be aware that all interactions related to multiformats are subject to the IPFS Code of Conduct.
Small note: If editing the Readme, please conform to the standard-readme specification.
License
MIT