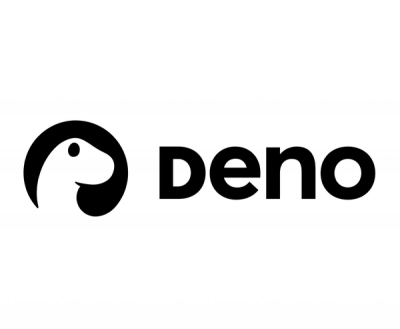
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
A CircleCI client for Node.js.
To install, go to your console/terminal and run:
npm install circleci
In your project, require the package:
var circleci = require("circleci");
Then you can run one of the following methods:
API call: GET /me
Provides information about the signed in user.
circleci.getUser(function(err, user){
if (err) {
console.log(err);
} else {
console.log(user.username); // e.g. jpstevens
}
});
API call: GET /projects
List of all the projects you're following on CircleCI, with build information organized by branch.
circleci.getProjects(function(err, projects){
if (err) {
console.log(err);
} else {
for (var i in projects) {
console.log(projects[i].username + "/" + projects[i].reponame); // e.g. jpstevens/circleci
}
}
});
API call: GET /projects
List of all the projects you're following on CircleCI, with build information organized by branch.
circleci.getProjects(function(err, projects){
if (err) {
console.log(err);
} else {
for (var i in projects) {
console.log(projects[i].username + "/" + projects[i].reponame); // e.g. jpstevens/circleci
}
}
});
TODO:
Documentation:
Testing:
Include methods to wrap:
FAQs
A Node.js client for CircleCI
We found that circleci demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.