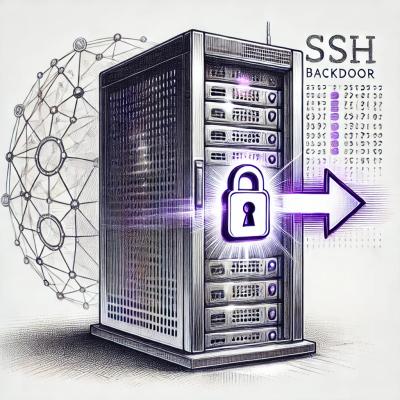
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
circular-linked-list
Advanced tools
Circular singly linked list, heavily based on Singlie by Klaus Sinani.
$ npm install --save circular-linked-list
$ npm run lint
$ npm run test
$ npm run test:coverage
$ npm run dist
$ npm run deploy
const CircularLinkedList = require("circular-linked-list");
const list = new CircularLinkedList();
list.append("B").prepend("A");
console.log(list.node(0));
// => Node { value: 'A', next: Node { value: 'B', next: [CircularLinkedList] } }
console.log(list.node(0).next);
// => Node { value: 'B', next: Node { value: 'A', next: [CircularLinkedList] } }
console.log(list.node(0).next.next);
// => Node { value: 'A', next: Node { value: 'B', next: [CircularLinkedList] } }
console.log(
list
.map(x => `[${x}]`)
.reverse()
.toArray()
);
// => [ '[B]', '[A]' ]
append(value[, value])
Linked List
Appends one of more nodes to the list.
value
any
Can be one or more comma delimited values. Each value corresponds to a single node.
list.append("A", "B", "C", "D");
// => { value: 'A', next: { value: 'B', next: [List] } }
prepend(value[, value])
Linked List
Prepends one of more nodes to the list.
value
any
Can be one or more comma delimited values. Each value corresponds to a single node.
list.append("C", "D");
// => { value: 'C', next: [List] }
list.prepend("B", "A");
// => { value: 'A', next: { value: 'B', next: { value: 'C', next: [List] } } }
head
any
Returns the value of the first node / head on the list.
list.append("A", "B");
console.log(list.head);
// => A
last
any
Returns the value of the last node on the list.
list.append("A", "B");
console.log(list.last);
// => B
length
Integer
Returns the length of the list.
list.append("A", "B");
console.log(list.length);
// => 2
isEmpty()
Boolean
Checks whether or not the list is empty.
list.append("A", "B");
console.log(list.isEmpty());
// => false
insert({value[, value], index})
Linked List
Inserts one or more nodes to the given index.
value
any
Can be one or more comma delimited values. Each value corresponds to a single node.
index
Integer
Can be an integer corresponding to a list index.
list.append("A", "B", "E");
list.insert({ value: ["C", "D"], index: 1 });
// => { value: 'A', next: { value: 'D', next: { value: 'C', next: { value: 'B', next: [List] } } } }
node(index)
Node
Return the node corresponding to the given index.
index
Integer
Can be an integer corresponding to a list index.
list.append("A", "B", "C", "D");
const node = list.node(0);
console.log(node);
// => { value: 'A', next: { value: 'B', next: [List] } }
console.log(node.value);
// => A
console.log(node.next);
// => { value: 'B', next: [List] }
get(index)
any
Return the value of node corresponding to the given index.
index
Integer
Can be an integer corresponding to a list index.
list.append("A", "B");
console.log(list.get(0));
// => A
console.log(list.get(0));
// => B
remove(index)
Linked List
Removes from the list the node located to the given index.
index
Integer
list.length - 1
Can be an integer corresponding to a list index.
If not provided, the last node of the list will be removed.
list.append("A", "B", "C", "D");
// => { value: 'A', next: [List] }
list.remove(0);
// => { value: 'B', next: [List] }
list.remove(0);
// => { value: 'C', next: [List] }
toArray()
Array
Converts the list into an array.
list.append("A", "B", "C");
// => { value: 'A', next: { value: 'B', next: [List] } }
const array = list.toArray();
console.log(array);
// => [ 'A', 'B', 'C' ]
clear()
Empty Linked List
Removes all nodes from the list.
list.append("A", "B", "C");
// => { value: 'A', next: { value: 'B', next: [List] } }
list.clear();
// => null
join([separator])
String
Joins the values of all nodes on the list into a string and returns the string.
separator
String
Comma ','
Specifies a string to separate each pair of adjacent node values of the array.
If omitted, the node values are separated with a comma ','
.
list.append("A", "B", "C");
// => { value: 'A', next: { value: 'B', next: [List] } }
console.log(list.join());
// => 'A,B,C'
console.log(list.join(""));
// => 'ABC'
console.log(list.join(" "));
// => 'A B C'
forEach(function)
undefined
Executes a provided function once for each node value.
function
Function
Function to execute for each node value.
const array = [];
list.append("A", "B", "C");
// => { value: 'A', next: { value: 'B', next: [List] } }
list.forEach(x => array.push(x));
console.log(array);
// => [ 'A', 'B', 'C' ];
map(function)
Linked List
Executes a provided function once for each node value.
function
Function
Function that produces a new node value for the new list.
list.append("A", "B", "C");
// => { value: 'A', next: { value: 'B', next: [List] } }
const mapped = list.map(x => `[${x}]`);
console.log(array.join(" "));
// => '[A] [B] [C]'
My work is released under the MIT licence. One can consider this project to be a fork of Singlie by Klaus Sinani.
FAQs
Circular singly linked list, heavily based on Singlie by Klaus Sinani.
The npm package circular-linked-list receives a total of 3 weekly downloads. As such, circular-linked-list popularity was classified as not popular.
We found that circular-linked-list demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.