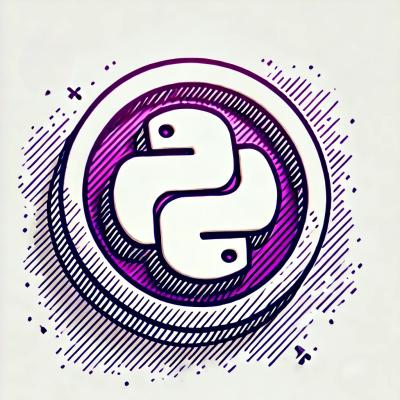
Security News
PyPI Now Supports iOS and Android Wheels for Mobile Python Development
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
civicrm-api
Advanced tools
JavaScript (and TypeScript) client for the [CiviCRM API](https://docs.civicrm.org/dev/en/latest/api/v4/usage/).
JavaScript (and TypeScript) client for the CiviCRM API.
npm install civicrm-api
import { createClient } from "civicrm-api";
const client = createClient({
baseUrl: "https://example.com/civicrm",
auth: { apiKey: "<your-api-key>" },
entities: { contact: "Contact" },
});
const contactRequest = client.contact.get({ where: [["id", "=", "1"]] }).one();
You can optionally create an API v3 client by providing the relevant configuration:
const client = createClient({
// ...
api3: {
enabled: true,
entities: {
contact: {
name: "Contact",
actions: {
getList: "getlist",
},
},
},
});
const contactsRequest = client.contact.getList();
createClient(options: ClientOptions): Client
Configure a CiviCRM API client.
The base URL of the CiviCRM installation.
The API key, JWT, or username and password that will be used to authenticate requests. Refer to the CiviCRM authentication documentation.
const client = createClient({
// ...
auth: { apiKey: "api-key" },
//=> X-Civi-Auth: Bearer api-key
auth: { jwt: "jwt" },
//=> X-Civi-Auth: Bearer jwt
auth: { username: "user", password: "pass" },
//=> X-Civi-Auth: Basic dXNlcjpwYXNz
});
An object containing entities the client will be used to make requests for. Keys will be used to reference the entity within the client, and values should match the entity in CiviCRM:
const client = createClient({
// ...
entities: {
contact: "Contact",
activity: "Activity",
},
});
Set default request options for all requests.
Accepts
the same options as fetch
.
Headers will be merged with the default headers.
Enable logging request and response details to the console.
Enable API v3 client.
const client = createClient({
// ...
api3: {
enabled: true,
},
});
An object containing entities and actions the API v3 client will be used to make requests for. Keys will be used to reference the entity within the client. The value contains the name of the entity in API v3, and an object of actions, where the key is used to reference the action within the client, and the value is the action in API v3.
const client = createClient({
// ...
api3: {
enabled: true,
entities: {
contact: {
name: "Contact",
actions: {
getList: "getlist",
},
},
},
},
});
client.<entity>: Api4.RequestBuilder
Create a request builder for a configured entity.
Request builders are used to build and execute requests.
Methods can be chained, and the request is executed by
calling .then()
or starting a chain with await
.
// Using .then()
client.contact
.get({ where: [["id", "=", "1"]] })
.one()
.then((contact) => {
//
});
// Using await
const contact = await client.contact.get({ where: [["id", "=", "1"]] }).one();
get(params?: Api4.Params): Api4.RequestBuilder
create(params?: Api4.Params): Api4.RequestBuilder
update(params?: Api4.Params): Api4.RequestBuilder
save(params?: Api4.Params): Api4.RequestBuilder
delete(params?: Api4.Params): Api4.RequestBuilder
getChecksum(params?: Api4.Params): Api4.RequestBuilder
Set the action for the request to the method name, and optionally set request parameters.
An object accepting parameters accepted by CiviCRM
including select
, where
, having
, join
,
groupBy
, orderBy
, limit
, offset
and values
.
Alternatively accepts a key-value object for methods like getChecksum
.
one(): Api4.RequestBuilder
Return a single record (i.e. set the index of the request to 0).
chain(label: string, requestBuilder: Api4.RequestBuilder): Api4.RequestBuilder
Chain a request for another entity within the current API call.
const contact = await client.contact
.get({ where: [["id", "=", "1"]] })
.chain(
"activity",
client.activity.get({ where: { target_contact_id: "$id" } }),
)
.one();
console.log(contact);
// => { id: 1, activity: [{ target_contact_id: 1, ... }], ... }
The label for the chained request, which will be used access the result of the chained request within the response.
A request builder for the chained request.
options(requestOptions: RequestInit): Api4.RequestBuilder
Set request options.
Accepts
the same options as fetch
.
Headers will be merged with the default headers.
client.contact.get().requestOptions({
headers: {
"X-Custom-Header": "value",
},
cache: "no-cache",
});
client.api3.<entity>: Api3.RequestBuilder
Create an API v3 request builder for a configured entity.
Request builders are used to build and execute requests.
Methods can be chained, and the request is executed by
calling .then()
or starting a chain with await
.
// Using .then()
client.api3.contact.getList({ input: "example" }).then((contacts) => {
//
});
// Using await
const contacts = await client.getList({ input: "example" });
<action>(params?: Api3.Params): Api3.RequestBuilder
Set the action for the request, and optionally set request parameters.
option(option: string, value: Api3.Value): Api3.RequestBuilder
Set API options.
client.api3.contact.getList().option("limit", 10);
options(requestOptions: RequestInit): Api3.RequestBuilder
Set request options.
Accepts
the same options as fetch
.
Headers will be merged with the default headers.
client.api3.contact.getList().requestOptions({
headers: {
"X-Custom-Header": "value",
},
cache: "no-cache",
});
npm install
npm test
npm run build
Increment the version number and create a tag:
npm version <major|minor|patch|prerelease>
Push the tag to GitHub:
git push --tags
Create a release on GitHub. The package will be built and published to npm automatically by GitHub Actions.
FAQs
TypeScript client for the CiviCRM API, supporting both API v4 and v3.
The npm package civicrm-api receives a total of 15 weekly downloads. As such, civicrm-api popularity was classified as not popular.
We found that civicrm-api demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.