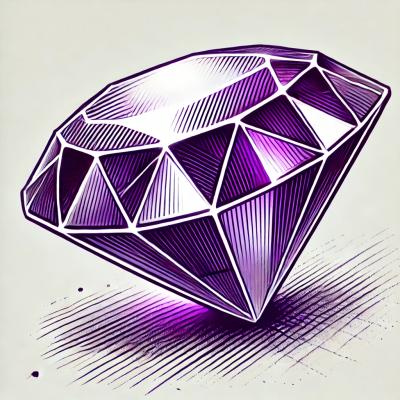
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Use Node JS to scan files on your server with ClamAV's clamscan binary. This is especially useful for scanning uploaded files provided by un-trusted sources.
The clamscan npm package is a Node.js wrapper for the ClamAV antivirus software. It allows you to scan files, directories, and streams for viruses and malware using ClamAV's powerful scanning capabilities.
Scan a file
This feature allows you to scan a specific file for viruses. The code initializes the clamscan instance and scans the specified file, returning whether the file is infected and the names of any detected viruses.
const NodeClam = require('clamscan');
const clamscan = new NodeClam().init();
clamscan.then(async clamscan => {
const { isInfected, file, viruses } = await clamscan.scanFile('/path/to/file');
if (isInfected) {
console.log(`The file ${file} is infected with ${viruses}`);
} else {
console.log(`The file ${file} is clean.`);
}
}).catch(err => {
console.error(`Error initializing ClamScan: ${err}`);
});
Scan a directory
This feature allows you to scan an entire directory for viruses. The code initializes the clamscan instance and scans the specified directory, returning whether any files are infected and listing the infected files and detected viruses.
const NodeClam = require('clamscan');
const clamscan = new NodeClam().init();
clamscan.then(async clamscan => {
const { isInfected, goodFiles, badFiles, viruses } = await clamscan.scanDir('/path/to/directory');
if (isInfected) {
console.log(`The directory contains infected files: ${badFiles}`);
} else {
console.log(`All files in the directory are clean.`);
}
}).catch(err => {
console.error(`Error initializing ClamScan: ${err}`);
});
Scan a stream
This feature allows you to scan a stream for viruses. The code initializes the clamscan instance and scans the provided stream, returning whether the stream is infected and the names of any detected viruses.
const NodeClam = require('clamscan');
const fs = require('fs');
const clamscan = new NodeClam().init();
clamscan.then(async clamscan => {
const stream = fs.createReadStream('/path/to/file');
const { isInfected, viruses } = await clamscan.scanStream(stream);
if (isInfected) {
console.log(`The stream is infected with ${viruses}`);
} else {
console.log(`The stream is clean.`);
}
}).catch(err => {
console.error(`Error initializing ClamScan: ${err}`);
});
The node-virustotal package is a Node.js client for the VirusTotal API, which allows you to scan files, URLs, and IP addresses for malware using multiple antivirus engines. Compared to clamscan, node-virustotal provides access to a broader range of antivirus engines but requires an API key and internet access.
Use Node JS to scan files on your server with ClamAV's clamscan binary or clamdscan daemon. This is especially useful for scanning uploaded files provided by un-trusted sources.
You will need to install ClamAV's clamscan binary and/or have clamdscan daemon running on your server. On linux, it's quite simple.
Fedora-based distros:
sudo yum install clamav
Debian-based distros:
sudo apt-get install clamav
As for OSX, I've not tried it, but, here's a promising looking site: http://www.clamxav.com/index.php . I would stick with linux varieties, though...
This module is not intended to work on a Windows server. This would be a welcome addition if someone wants to add that feature (I may get around to it one day but have no urgent need for this).
npm install clamscan
Licensed under the MIT License:
All of the values listed in the example below represent the default values for their respective configuration item.
You can simply do this:
var clam = require('clamscan')();
And, you'll be good to go.
BUT: If you want more control, you can specify all sorts of options.
var clam = require('clamscan')({
remove_infected: false, // If true, removes infected files
quarantine_infected: false, // False: Don't quarantine, Path: Moves files to this place.
scan_log: null, // Path to a writeable log file to write scan results into
debug_mode: false // Whether or not to log info/debug/error msgs to the console
file_list: null, // path to file containing list of files to scan
scan_recursively: true, // If true, deep scan folders recursively
clamscan: {
path: '/usr/bin/clamscan', // Path to clamscan binary on your server
db: null, // Path to a custom virus definition database
scan_archives: true, // If true, scan archives (ex. zip, rar, tar, dmg, iso, etc...)
active: true // If true, this module will consider using the clamscan binary
},
clamdscan: {
path: '/usr/bin/clamdscan', // Path to the clamdscan binary on your server
config_file: null, // Specify config file if it's in an unusual place
multiscan: true, // Scan using all available cores! Yay!
reload_db: false, // If true, will re-load the DB on every call (slow)
active: true // If true, this module will consider using the clamdscan binary
},
preference: 'clamdscan' // If clamdscan is found and active, it will be used by default
});
Here is a non-default values example (to help you get an idea of what the proper-looking values should be):
var clam = require('clamscan')({
remove_infected: true, // Removes files if they are infected
quarantine_infected: '~/infected/', // Move file here. remove_infected must be FALSE, though.
scan_recursively: true, // Choosing false here will save some CPU cycles
scan_log: '/var/log/node-clam', // You're a detail-oriented security professional.
debug_mode: true // This will put some debug info in your js console
file_list: '/home/webuser/scan_files.txt', // path to file containing list of files to scan
clamscan: {
path: '/usr/bin/clam', // I dunno, maybe your clamscan is just call "clam"
db: '/usr/bin/better_clam_db', // Path to a custom virus definition database
scan_archives: false, // Choosing false here will save some CPU cycles
active: false // you don't want to use this at all because it's evil
},
clamdscan: {
path: '/bin/clamdscan', // Special path to the clamdscan binary on your server
config_file: __dirname + '/logs/clamscan-log', // logs file in your app directory
multiscan: false, // You hate speed and multi-threaded awesome-sauce
reload_db: true, // You want your scans to run slow like with clamscan
active: false // you don't want to use this at all because it's evil
},
preference: 'clamscan' // If clamscan is found and active, it will be used by default
});
This method allows you to scan a single file.
file_path
(string) Represents a path to the file to be scanned.callback
(function) Will be called when the scan is complete. It takes 3 parameters:err
(string or null) A standard error message string (null if no error)file
(string) The original file_path
passed into the is_infected
method.is_infected
(boolean) True: File is infected; False: File is clean.clam.is_infected('/a/picture/for_example.jpg', function(err, file, is_infected) {
if(err) {
console.log(err);
return false;
}
if(is_infected) {
res.send({msg: "File is infected!"});
} else {
res.send({msg: "File is clean!"});
}
});
Allows you to scan an entire directory for infected files. This obeys your recursive
option even for clamdscan
which does not have a native way to turn this feature off. If you have multiple paths, send them in an array to scan_files
.
TLDR: For maximum speed, don't supply a file_callback
.
If you choose to supply a file_callback
, the scan will run a little bit slower (depending on number of files to be scanned) for clamdscan
. If you are using clamscan
, while it will work, I'd highly advise you to NOT pass a file_callback
... it will run incredibly slow.
dir_path
(string) Full path to the directory to scan.end_callback
(function) Will be called when the entire directory has been completely scanned. This callback takes 3 parameters:err
(string or null) A standard error message string (null if no error)good_files
(array) List of the full paths to all files that are clean.bad_files
(array) List of the full paths to all files that are infected.file_callback
(function) Will be called after each file in the directory has been scanned. This is useful for keeping track of the progress of the scan. This callback takes 3 parameters:err
(string or null) A standard error message string (null if no error)file
(string) Path to the file that just got scanned.is_infected
(boolean) True: File is infected; False: File is clean.clam.scan_dir('/some/path/to/scan', function(err, good_files, bad_files) {
if(!err) {
if(bad_files.length > 0) {
res.send({msg: "Your directory was infected. The offending files have been quarantined."});
} else {
res.send({msg: "Everything looks good! No problems here!."});
}
} else {
// Do some error handling
}
});
This allows you to scan many files that might be in different directories or maybe only certain files of a single directory. This is essentially a wrapper for is_infected
that simplifies the process of scanning many files or directories.
files
(array) A list of strings representing full paths to files you want scanned.end_callback
(function) Will be called when the entire directory has been completely scanned. This callback takes 3 parameters:err
A standard error message string (null if no error)good_files
(array) List of the full paths to all files that are clean.bad_files
(array) List of the full paths to all files that are infected.file_callback
(function) Will be called after each file in the directory has been scanned. This is useful for keeping track of the progress of the scan. This callback takes 3 parameters:err
(string or null) A standard error message string (null if no error)file
(string) Path to the file that just got scanned.is_infected
(boolean) True: File is infected; False: File is clean.var scan_status = {
good: 0,
bad: 0
};
var files = [
'/path/to/file/1.jpg',
'/path/to/file/2.mov',
'/path/to/file/3.rb'
];
clam.scan_files(files, function(err, good_files, bad_files) {
if(!err) {
if(bad_files.length > 0) {
res.send({
msg: good_files.length + ' files were OK. ' + bad_files.length + ' were infected!',
bad: bad_files,
good: good_files
});
} else {
res.send({msg: "Everything looks good! No problems here!."});
}
} else {
// Do some error handling
}
}, function(err, file, is_infected) {
if(is_infected) {
scan_status.bad++;
} else {
scan_status.good++;
}
console.log("Scan Status: " + (scan_status.bad + scan_status.good) + "/" + files.length);
});
Got a missing feature you'd like to use? Found a bug? Go ahead and fork this repo, build the feature and issue a pull request.
0.6.0 (2015-01-02)
NOTE: There are some breaking changes on this release. Since this is still a pre-version 1 release, I decided to only do a minor bump to 0.4.0
clamscan
has been removed because of irregularities with different systems--namely if you had max_forks
set to 3, it would sometimes only scan the first or last file in the group... not good.clamdscan
. This ultimately negates the downside of removing the forking capability mentioned in item one. This is a really big improvement (many orders of magnitude) if your system has access to the clamdscan
daemon.file_list
option allowing one to specify a text file that lists (one per line) paths to files to be scanned. This is great if you need to scan hundreds or thousands of random files.clam_path
option has been moved to clam.path
db
option has been moved to clam.db
scan_archives
option has been moved to clam.scan_archives
scan_files
now supports directories as well and will obey your scan_recursively
option.FAQs
Use Node JS to scan files on your server with ClamAV's clamscan/clamdscan binary or via TCP to a remote server or local UNIX Domain socket. This is especially useful for scanning uploaded files provided by un-trusted sources.
The npm package clamscan receives a total of 57,585 weekly downloads. As such, clamscan popularity was classified as popular.
We found that clamscan demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.