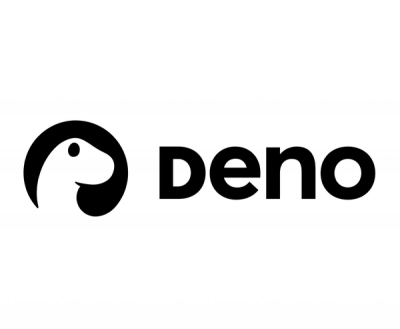
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
clarifai-nodejs
Advanced tools
This library is currently in developer preview, any improvements & feedback welcome!
This is the official Node.js client for interacting with our powerful API. The Clarifai Node.js SDK offers a comprehensive set of tools to integrate Clarifai's AI platform to leverage computer vision capabilities like classification, detection, segmentation and natural language capabilities like classification, summarisation, generation, Q&A, etc into your applications. With just a few lines of code, you can leverage cutting-edge artificial intelligence to unlock valuable insights from visual and textual content.
Website | Schedule Demo | Signup for a Free Account | API Docs | Clarifai Community | Node.js SDK Docs | Examples | Discord
Give the repo a star ⭐
npm install clarifai-nodejs
To use Clarifai Node.js in Next.js App Directory with server components, you will need to add clarifai-nodejs-grpc package (which is one of the primary dependency of Clarifai Node.js SDK) to the serverComponentsExternalPackages config of next.config.js
/** @type {import('next').NextConfig} */
const nextConfig = {
experimental: {
serverComponentsExternalPackages: ['clarifai-nodejs-grpc'],
},
}
module.exports = nextConfig
Clarifai uses Personal Access Tokens(PATs) to validate requests. You can create and manage PATs under your Clarifai account security settings.
Export your PAT as an environment variable. Then, import and initialize the API Client.
Set PAT as environment variable through terminal:
export CLARIFAI_PAT={your personal access token}
or use dotenv to load environment variables via a .env
file
Using the celebrity face recognition model to predict the celebrity in a given picture. For list of all available models visit clarifai models page.
import { Input, Model } from "clarifai-nodejs";
const input = Input.getInputFromUrl({
inputId: "test-image",
imageUrl:
"https://samples.clarifai.com/celebrity.jpeg",
});
const model = new Model({
authConfig: {
pat: process.env.CLARIFAI_PAT!,
userId: process.env.CLARIFAI_USER_ID!,
appId: process.env.CLARIFAI_APP_ID!
},
modelId: "celebrity-face-recognition",
});
model
.predict({
inputs: [input],
})
.then((response) => {
const result = response?.[0].data?.conceptsList[0].name ?? "unrecognized";
console.log(result);
})
.catch(console.error);
Using a custom workflow built on clarifai.com to analyze sentiment of a given image. For list of all available workflows visit clarifai workflows page
import { Input, Workflow } from "clarifai-nodejs";
const input = Input.getInputFromUrl({
inputId: "test-image",
imageUrl:
"https://samples.clarifai.com/celebrity.jpeg",
});
const workflow = new Workflow({
authConfig: {
pat: process.env.CLARIFAI_PAT!,
userId: process.env.CLARIFAI_USER_ID!,
appId: process.env.CLARIFAI_APP_ID!
},
workflowId: "workflow-238a93",
});
workflow
.predict({
inputs: [input],
})
.then((response) => {
const result =
response.resultsList[0].outputsList[0].data?.regionsList[0].data
?.conceptsList[0].name ?? "unrecognized";
console.log(result);
})
.catch(console.error);
On Clarifai, apps act as a central repository for models, datasets, inputs and other resources and information. Checkout how to create apps on clarifai portal.
import { User } from "clarifai-nodejs";
export const user = new User({
pat: process.env.CLARIFAI_PAT!,
userId: process.env.CLARIFAI_USER_ID!,
appId: process.env.CLARIFAI_APP_ID!,
});
const list = await user
.listApps({
pageNo: 1,
perPage: 20,
params: {
sortAscending: true,
},
})
.next();
const apps = list.value;
console.log(apps);
For full usage details, checkout our API Reference docs
FAQs
The official Clarifai Node.js SDK
The npm package clarifai-nodejs receives a total of 33 weekly downloads. As such, clarifai-nodejs popularity was classified as not popular.
We found that clarifai-nodejs demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.