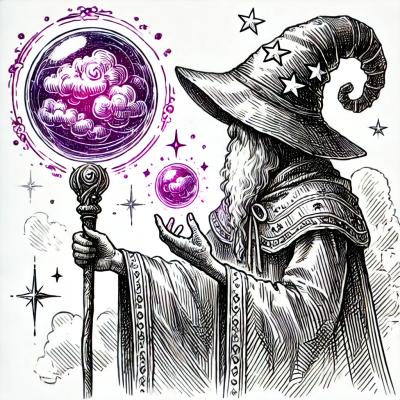
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
class-transformer-validator
Advanced tools
A simple wrapper around class-transformer and class-validator which provides nice and programmer-friendly API.
A simple plugin for class-transformer and class-validator which combines them in a nice and programmer-friendly API.
npm install class-transformer-validator --save
This package is only a simple plugin/wrapper, so you have to install the required modules too because it can't work without them. See detailed installation instruction for the modules installation:
The usage of this module is very simple.
import { IsEmail } from 'class-validator';
import { transformAndValidate } from "class-transformer-validator";
// declare the class using class-validator decorators
class User {
@IsEmail()
public email: string;
public hello(): string {
return "World!";
}
}
// then load the JSON string from any part of your app
const userJson: string = loadJsonFromSomething();
// transform the JSON to class instance and validate it correctness
transformAndValidate(User, userJson)
.then((userObject: User) => {
// now you can access all your class prototype method
console.log(`Hello ${userObject.hello()}`); // prints "Hello World!" on console
})
.catch(error => {
// here you can handle error on transformation (invalid JSON)
// or validation error (e.g. invalid email property)
console.err(error);
});
You can also transform and validate plain JS object (e.g. from express req.body). Using ES7 async/await syntax:
async (req, res) => {
try {
// transform and validate request body
const userObject = await transformAndValidate(User, req.body);
// intered type of userObject is User, you can access all class prototype properties and methods
} catch (error) {
// your error handling
console.err(error);
}
}
There is available one function with two overloads:
function transformAndValidate<T extends PlainObject>(classType: ClassType<T>, jsonString: string, options?: TransformValdiationOptions): Promise<T>;
function transformAndValidate<T extends PlainObject>(classType: ClassType<T>, object: PlainObject, options?: TransformValdiationOptions): Promise<T>;
classType
- an class symbol, a constructor function which can be called with new
type ClassType<T> = {
new (...args: any[]): T;
}
jsonString
- a normal string containing JSON
object
- plain JS object with some properties (not empty - {}
). PlainObject
is a defined as normal JS object type but with some properties defined, to provide compile-time error when e.g. number is passed as parameter:
type PlainObject = {
[property: string]: any;
}
options
- optional options object, it has two optional propertiesinterface TransformValdiationOptions {
validator?: ValidatorOptions;
transformer?: ClassTransformOptions;
}
You can use it to pass options for class-validator
(more info) and for class-transformer
(more info).
The class-transformer and class-validator are more powerfull than it was showed in the simple usage sample, so go to their github page and check out they capabilities!
FAQs
A simple wrapper around class-transformer and class-validator which provides nice and programmer-friendly API.
The npm package class-transformer-validator receives a total of 25,912 weekly downloads. As such, class-transformer-validator popularity was classified as popular.
We found that class-transformer-validator demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.