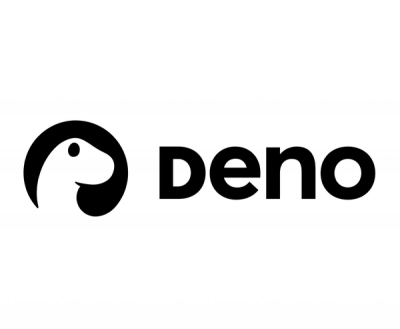
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
classy-router
Advanced tools
Class based HTTP router
Lightweight, has only debug and path-to-regexp dependencies. It is compatible with Express 4, Hapi or simple HTTP server.
This module is a follow-up of ES6 Class based Express routing blog post.
npm install classy-router -S
Instead of writing app.get('/', function() {});
or server.router({ method: 'GET', path: '/', handler: function() {}})
to define request handlers, you use
a Class to hold methods registered as route handlers to the underlying
framework, Express or Hapi.
Additionnaly, it implements a simple routing mechanism with raw HTTP server.
import ClassyRouter from 'classy-router'
class Router extends ClassyRouter {
get routes() {
'/': 'index'
}
index(req, res) {
console.log('Incoming request:', req.url);
return res.send('Response from server');
}
}
var app = require('express')();
var router = new Router(app);
app.listen(3000);
Or
var app = require('express')();
app.use(Router.middleware(app));
app.listen(3000);
var router = new Router();
router.listen(3000);
Or
var http = require('http');
http.createServer(Router.middleware()).listen(3000);
Or
Router.createServer().listen(3000);
Or
Router.listen(3000);
Router.createServer().
Router.createServer().listen(PORT, done);
Router.listen().
Router.listen(PORT, done);
Router.create().
var router = Router.create();
assert.ok(router instanceof Router);
Router.dispatch() - express middleware.
app.use(Router.middleware(app));
Router.dispatch() - HTTP server.
http.createServer(Router.middleware());
App extends Router.
class App extends Router {
get routes() {
return {
'/': 'index'
};
}
index(req, res, next) {
return res.end('OK');
}
}
var app = new App();
app.listen(PORT, done);
GET /.
request(this.app)
.get('/')
.expect('OK')
.expect(200, done);
var server = TestApp.createServer();
request(this.app)
.get('/aoizheuaziouh')
.expect(404, done);
FAQs
Class based HTTP routing
The npm package classy-router receives a total of 3 weekly downloads. As such, classy-router popularity was classified as not popular.
We found that classy-router demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.