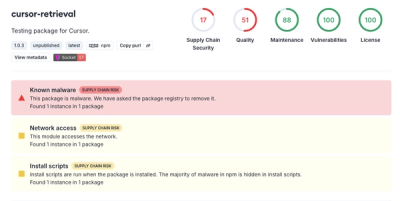
Security News
The Risks of Misguided Research in Supply Chain Security
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
claudia-api-builder
Advanced tools
#Claudia API Builder
This utility simplifies Node.js Lambda - API Gateway handling. It helps you:
The API builder is designed to work with Claudia, and add minimal overhead to client projects.
An instance of the Claudia API Builder should be used as the module export from your API module. You can create a new API simply
by instantiating a new ApiBuilder
, then defining HTTP handlers for paths by calling .get
, .put
, and .post
. For example, the following
snippet creates a single handler for a GET
call to /greet
, responding with a parameterised message:
var ApiBuilder = require('claudia-api-builder'),
api = new ApiBuilder(),
superb = require('superb');
module.exports = api;
api.get('/greet', function (request) {
return request.queryString.name + ' is ' + superb();
});
For a more detailed example, see the Web API Example project.
Claudia will automatically bundle all the parameters and pass it to your handler, so you do not have to define request and response models. The request
object passed to your handler contains the following properties:
queryString
: a key-value map of query string argumentsenv
: a key-value map of the API Gateway stage variables (useful for storing resource identifiers and access keys)headers
: a key-value map of all the HTTP headers posted by the clientpost
: in case of a FORM post (application/x-form-www-urlencoded
), a key-value map of the values postedbody
: in case of an application/json
POST or PUT, the body of the request, parsed as a JSON objectpathParams
: arguments from dynamic path parameter mappings (such as '/people/{name}')You can either respond synchronously (just return a value, as above), or respond with a Promise
. In that case, the lambda function will wait until the
Promise
resolves or rejects before responding. Please note that AWS currently uses Node.js 0.10.36, which does not include the standard Promise
library,
so you need to include a third party one. API Builder just checks for the .then
method, so it should work with any A+ Promise library.
By default, Claudia.js uses 500 as the HTTP response code for all errors, and 200 for successful operations. The application/json
content type is default for both successes and failures. You can change all that by using the optional third argument to handler definition methods. All keys are optional, and the structure is:
error
: a number or a key-value map. If a number is specified, it will be used as the HTTP response code. If a key-value map is specified, it should have the following keys:
code
: HTTP response codecontentType
: the content type of the responseheaders
: a key-value map of hard-coded header values, or an array enumerating custom header names. See Custom headers below for more informationsuccess
: a number or a key-value map. If a number is specified, it will be used as the HTTP response code. If a key-value map is specified, it should have the following keys:
code
: HTTP response codecontentType
: the content type of the responseheaders
: a key-value map of hard-coded header values, or an array enumerating custom header names. See Custom headers below for more informationapiKeyRequired
: boolean, determines if a valid API key is required to call this method. See Requiring Api Keys below for more informationFor example:
api.get('/greet', function (request) {
return request.queryString.name + ' is ' + superb();
}, {
success: { contentType: 'text/plain' },
error: {code: 403}
});
These special rules apply to content types and codes:
text/plain
or text/html
, only the error message is sent back in the body, not the entire error structure.application/json
, the entire error structure is sent back with the response.application/json
, the response is JSON-encoded. So if you just send back a string, it will have quotes around it.text/plain
, text/xml
, text/html
or application/xml
, the response is sent back without JSON encoding (so no extra quotes).Location
header, so you can easily create HTTP redirects.To see these options in action, see the Serving HTML Example project.
You can force a method to require an API key by using an optional third argument to handler definition methods, and setting the apiKeyRequired
property on it. For example:
api.get('/echo', function (request) { ... }, {apiKeyRequired: true});
See How to Use an API Key in API Gateway for more information on creating and using API keys.
Claudia API Builder provides limited support for custom HTTP headers. AWS API Gateway requires all custom headers to be enumerated upfront, and you can use the success.headers
and error.headers
keys of your handler configuration for that. There are two options for enumerating headers:
api.get('/hard-coded-headers', function () {
return 'OK';
}, {success: {headers: {'X-Version': '101', 'Content-Type': 'text/plain'}}});
ApiResponse(contents, headers)
from your handler method. For example:api.get('/programmatic-headers', function () {
return new api.ApiResponse('OK', {'X-Version': '202', 'Content-Type': 'text/plain'});
}, {success: {headers: ['X-Version', 'Content-Type']}});
Due to the limitations with Lambda error processing, the error.handlers
key can only be hard-coded. Dynamic values for error handlers are not supported.
To see custom headers in action, see the Custom Headers Example Project.
Claudia API builder automatically sets up the API to allow cross-origin resource sharing (CORS). The most common usage scenario for API Gateway projects is to provide dynamic functions to Web sites served on a different domain, so CORS is necessary to support that use case. To simplify things, by default, APIs allow calls from any domain.
If you plan to proxy both the main web site and the APIs through a CDN and put them under a single domain, or if you want to restrict access to your APIs, you can override the default behaviour for CORS handling.
To completely prevent CORS access, use:
api.corsOrigin(false)
To hard-code the CORS origin to a particular domain, call the corsOrigin
function with a string, representing the target origin:
api.corsOrigin('https://www.claudiajs.com')
To dynamically choose an origin (for example to support different configurations for development and production use, or to allow multiple sub-domains to access your API), call pass a JavaScript function into corsOrigin
. Your function will receive the request object (filled with stage variables and the requesting headers) and should return a string with the contents of the origin header. This has to be a synchronous function (promises are not supported).
api.corsOrigin(function (request) {
if (/claudiajs.com$/.test(request.headers.Origin)) {
return request.headers.Origin;
}
return '';
});
If your API endpoints use HTTP headers as parameters, you may need to allow additional headers in Access-Control-Allow-Headers
. To do so, just call the corsHeaders
method on the API, and pass a string with the Allow-Header
value.
api.corsHeaders('Content-Type,X-Amz-Date,Authorization,X-Api-Key,X-Api-Version');
To see this in action, see the Custom CORS Example Project. For more information on CORS, see the MDN CORS page.
FAQs
Simplify AWS ApiGateway handling
The npm package claudia-api-builder receives a total of 3,910 weekly downloads. As such, claudia-api-builder popularity was classified as popular.
We found that claudia-api-builder demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.