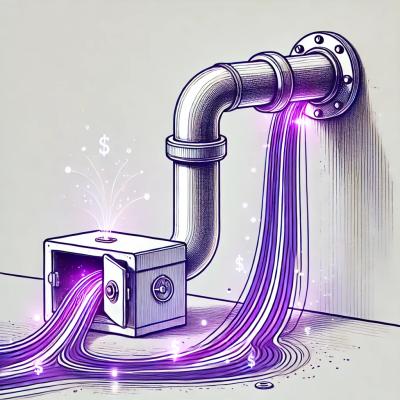
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
A Node.js Active Record with a charming declaration and simple usage. Supports Redis as backend but can be easily extended
version 1.2.0
Clay is a lightweight active record for Node.js applications. It leverages the effort of declaring models and its relationships, and store them in any backend.
Clay comes with builtin support for Redis but has a very simple interface with storage mechanisms, so that you can write your own backend.
npm install clay
Let's go by example:
var redis = require('redis').createConnection();
var models = require('clay');
var User = models.declare("User", function(it, kind){
it.has.field("name", kind.string);
it.has.field("email", kind.email);
it.has.field("password", kind.hashOf(["name", "email"]));
it.has.method('greet', function() {
return [
"Hello, my name is ", this.name, ", it's nice to meet you"
].join('');
});
});
var Build = models.declare("Build", function(it, kind){
it.has.field("status", kind.numeric);
it.has.field("error", kind.string);
it.has.field("output", kind.string);
it.has.one("author", User, "builds");
});
var BuildInstruction = models.declare("BuildInstruction", function(it, kind){
it.has.field("name", kind.string);
it.has.field("repository_address", kind.string);
it.has.field("build_command", kind.string);
it.validates.uniquenessOf("name");
it.has.index("repository_address");
it.has.many("builds", Build, "instruction");
it.has.one("owner", User, "created_instructions");
});
Clay provides syntactic sugar function calls that will help you declare models in a very classy, fashion and expressive way.
It is possible through the callback passed to the models.declare
call, and it has the arguments it
and kind
. These two will help
you out to declare your model.
Clay's field kinds are no more than just functions responsible to transform and validate data.
You can implement your own field kind, or use the builtin kinds. They come with valitation out of the box:
shorthand for the regexp /^[a-zA-z-0-9]+$/
USAGE:
var Foo = models.declare('Foo', function(it, kind){
it.has.field('example', kind.alphanumeric);
});
shorthand for the regexp /^[0-9]+$/
also returns an integer through parseInt
USAGE:
var Foo = models.declare('Foo', function(it, kind){
it.has.field('example', kind.numeric);
});
returns a new Date(value)
, be aware that clay is date-utils powered
USAGE:
var Foo = models.declare('Foo', function(it, kind){
it.has.field('birth_day', kind.datetime);
});
auto-assigns a new Date()
to the given field whenever a new Model
is issued
USAGE:
var Foo = models.declare('Foo', function(it, kind){
it.has.field('created_at', kind.auto);
});
var x = new Foo();
x.created_at.toFormat("DD/MM/YYYY") /* should be "today" */
shorthand for the regexp /^\w+[@]\w+[.]\w{2,}$/
USAGE:
var Foo = models.declare('Foo', function(it, kind){
it.has.field('example', kind.email);
});
any string of any size, although it's trimmed
USAGE:
var Foo = models.declare('Foo', function(it, kind){
it.has.field('example', kind.string);
});
any string of any size, will me returned as a slug,
for example the input Hello World
turns into hello-world
USAGE:
var Foo = models.declare('Foo', function(it, kind){
it.has.field('example', kind.slug);
});
Now this is cool :)
Let's say you want to have a password kind of field.
One of the ways to implement such thing is by concatenating with other field value(s) and then hashed with md5 or sha1, right?
That is what the field kind hashOf
does for you. Just declare on it
which fields must be ued in the concatenation, that will be done
automatically for you.
You can take a look on its unit tests to see how it works precisely, but here is an example:
var u1 = new User({
name: "John Doe",
email: "example@email.com",
password: '123'
});
assert.equal(u1.password, "f8543ecd4084527d7bc443f272a38c6390bbb7d6")
the password was already converted from 123
to
f8543ecd4084527d7bc443f272a38c6390bbb7d6
, which is the sha1
sum of
the string:
John Doe|sha1-clay|example@email.com|sha1-clay|123
var assert = require('assert');
var lettuce_instructions = new BuildInstruction({
name: 'Lettuce Unit Tests',
repository_address: 'git://github.com/gabrielfalcao/lettuce.git',
build_command: 'make unit'
});
lettuce_instructions.save(function(err, pk, model_instance, storage, redis_connection){
assert.equal(pk, 'clay:BuildInstruction:id:1');
});
Clay "kind of supports
one-to-many and many-to-one "relationships", in order to declare them
you can just use either: it.has.one()
or it.has.many()
declaration.
Nevertheless there are two important things you must know about how Clay leverages the relationship feature:
In my opinion, a snippet is worth than words:
Supposing you have this declaration
var Person = models.declare("Person", function(it, kind){
it.has.field("name", kind.string);
});
var Belonging = models.declare("Belonging", function(it, kind){
it.has.field("description", kind.string);
it.has.one("owner", Person, "belongings");
});
This is telling Clay that a Belonging has an owner, as well as that a Person has many belongings
Technically speaking, it means that internally Clay will make the declaration above idempodent to the example below:
var Belonging = models.declare("Belonging", function(it, kind){
it.has.field("description", kind.string);
});
var Person = models.declare("Person", function(it, kind){
it.has.field("name", kind.string);
it.has.many("belonging", Person, "owner");
});
Now, ain't that so cool?
Now whenever you persist your data, as long as the dynamically assigned objects were already persisted, their references will be kept tracked by its related objects.
Clay provides an object-oriented-friendly object declaration.
So as expected, you can define class-level methods, instance-level methods, getters and setters.
Once again, using code to show the magic:
var Animal = models.declare("Animal", function(it, kind){
it.has.field("name", kind.string);
it.has.field("sex", kind.string);
it.has.class_method("create_male", function(name){
return new this({sex: "male", name: name});
});
});
// now you can do:
var leo = Animal.create_male("Lion");
assert.equal(leo.name, "Lion");
assert.equal(leo.sex, "male");
var Person = models.declare("Person", function(it, kind){
it.has.field("name", kind.string);
it.has.method("say_hello", function(){
// yes, "this" is bound to the actual instance
console.log("Hello, I am " + this.name);
});
});
var john = new Person({name: "John Doe"});
john.say_hello();
would produce the output
Hello, I am John Doe
var BankAccount = models.declare("BankAccount", function(it, kind){
it.has.field("balance", kind.numeric);
it.has.getter("is_positive", function(){
return this.balance > 0;
});
it.has.getter("is_negative", function(){
return this.balance < 0;
});
});
var red = new BankAccount({balance: -5000});
red.is_negative() // true
var green = new BankAccount({balance: 99});
green.is_positive() // true
would produce the output
Hello, I am John Doe
var Person = models.declare("Person", function(it, kind){
it.has.field("first_name", kind.string);
it.has.field("last_name", kind.string);
it.has.setter("name", function(name){
var parts = name.trim().split(/\s+/);
if (parts.length == 2) {
this.first_name = parts[0];
this.last_name = parts[1];
} else {
this.first_name = name;
this.last_name = "";
}
});
it.has.getter("name", function(){
return [this.first_name, this.last_name].join(' ');
});
});
var john = new Person();
john.name = "John Doe";
assert.equal(john.first_name, "John");
assert.equal(john.last_name, "John");
var assert = require('assert');
var gabrielfalcao = new Build({
name: 'Gabriel Falcão',
email: 'gabriel@yipit.com',
password: '123'
});
var b1 = new Build({
status: 0,
error: '',
output: 'Worked!',
author: gabrielfalcao
});
var b2 = new Build({
status: 32,
error: 'Failed!',
output: 'OOps',
author: gabrielfalcao
});
var lettuce_unit = new BuildInstruction({
name: "Lettuce Unit Tests",
repository_address: 'git://github.com/gabrielfalcao/lettuce.git',
build_command: 'make unit',
owner: gabrielfalcao,
builds: [b1, b2]
});
gabrielfalcao.save(function(e, gabrielfalcao_pk){
b1.save(function(e, b1_pk){
b2.save(function(e, b2_pk){
lettuce_unit.save(function(e4, lettuce_unit_pk){
// from now on, whenever you fetch the
// BuildInstruction 'Lettuce Unit Tests', the related objects
// will be automatically fetched from the database
});
});
});
});
BuildInstruction.find_by_id(1, function(e, found){
assert.equal(found.name, 'Lettuce Unit Tests');
assert.equal(found.repository_address, 'git://github.com/gabrielfalcao/lettuce.git');
assert.equal(
"Will now build: {name}".render(found),
"Will now build: Lettuce Unit Tests"
);
});
Clay attempts to be really simple to use, and for the sake of this fact there is a lot of magic here.
When you declare any model with Clay, you have special class-methods available right away.
In order to search by any declared field, all you need to do is call
YourModel.find_by_fieldname
, where YourModel
is the return of
models.declare()
and fieldname
is the name of any fields you have
declared. All of them will be available.
It takes just 2 parameters: the RegExp
that will be used to match
against values and a callback.
The callback, takes 2 parameters: an error and an array with instances of models.
var adam = new User({
name: "Adam Nelson",
email: "adam@yipit.com",
password: '123'
});
adam.save(function(e, pk, instance){
User.find_by_email(/yipit.com$/, function(e, found){
assert.equal(found.length, 1);
assert.equal(found.first.name, 'Adam Nelson');
assert.equal(found.first.email, 'adam@yipit.com');
});
});
cd clay.js
npm install
npm install -g jake
jake unit
jake functional
PS.: you need to have redis running in order to make the functional tests running
<clay - active record for node.js with redis backend>
Copyright (C) <2011> Gabriel Falcão <gabriel@yipit.com>
Permission is hereby granted, free of charge, to any person
obtaining a copy of this software and associated documentation
files (the "Software"), to deal in the Software without
restriction, including without limitation the rights to use,
copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the
Software is furnished to do so, subject to the following
conditions:
The above copyright notice and this permission notice shall be
included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES
OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT
HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY,
WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR
OTHER DEALINGS IN THE SOFTWARE.
FAQs
Clay
The npm package clay receives a total of 592 weekly downloads. As such, clay popularity was classified as not popular.
We found that clay demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 16 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.