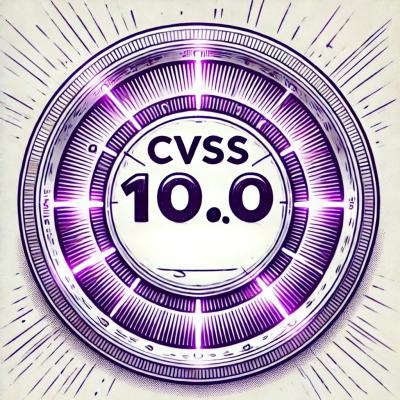
Security News
cURL Project and Go Security Teams Reject CVSS as Broken
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
cloud-task-wrapper
Advanced tools
This package helps with utils that are not offered by @google-cloud/tasks
Cloud Tasks Wrapper API client for Node.js
This module offers additional wrapper on top of @google-cloud/tasks (https://www.npmjs.com/package/@google-cloud/task). There are some of the features that are missing as part of the current @google-cloud/task module. This cloud task wrapper aims at addressing the missing utils by using @google-cloud/task under the hood.
npm i cloud-task-wrapper
// Imports the Google Cloud Tasks Wrapper library.
const { CloudTaskWrapper } = require("cloud-task-wrapper");
async function quickstart() {
// TODO(developer): Uncomment these lines and replace with your values.
// const project = 'my-project-id';
// const queue = 'my-appengine-queue';
// const location = 'us-central1';
const config = {
project,
location,
};
const wrapper = new CloudTaskWrapper(config);
const cloudTasks = await wrapper.getCloudTasksByRegex(regex, queue); //regex that matches name of your cloud task
console.log(`List of matching cloud tasks ${cloudTasks}`);
}
quickstart();
// Imports the Google Cloud Tasks Wrapper library.
const { CloudTaskWrapper } = require("cloud-task-wrapper");
async function quickstart() {
// TODO(developer): Uncomment these lines and replace with your values.
// const project = 'my-project-id';
// const queue = 'my-appengine-queue';
// const location = 'us-central1';
const config = {
project,
location,
};
const wrapper = new CloudTaskWrapper(config);
const cloudTasks = await wrapper.getCloudTasksByRegex(regex, queue); //regex that matches name of your cloud task
for (const cloudTask of cloudTasks) {
const taskName = cloudTask.name.split("/").pop();
await wrapper.deleteCloudTaskByName(taskName, queue);
}
console.log(`List of deleted cloud tasks ${cloudTasks}`);
}
quickstart();
// Imports the Google Cloud Tasks Wrapper library.
const { CloudTaskWrapper } = require("cloud-task-wrapper");
async function quickstart() {
// TODO(developer): Uncomment these lines and replace with your values.
// const project = 'my-project-id';
// const queue = 'my-appengine-queue';
// const location = 'us-central1';
const config = {
project,
location,
};
const wrapper = new CloudTaskWrapper(config);
//TODO: Uncomment these lines and replace with values name of the cloud task to be updated, time in minutes to be triggered (UTC), new Name is optional
//const name = 'cloud task name';
//const inMinutes = 20
//const newName = 'This is optional';
const updatedCloudTask = await wrapper.updateCloudTaskScheduledTime(
name,
20,
queue,
newName
);
console.log(`Updated the cloud task and its new name - ${updatedCloudTask}`);
}
quickstart();
// Imports the Google Cloud Tasks Wrapper library.
const { CloudTaskWrapper } = require("cloud-task-wrapper");
async function quickstart() {
// TODO(developer): Uncomment these lines and replace with your values.
// const project = 'my-project-id';
// const queue = 'my-appengine-queue';
// const location = 'us-central1';
const config = {
project,
location,
};
const wrapper = new CloudTaskWrapper(config);
const cloudTask = await wrapper.getCloudTaskByName(name, queue); //just the name of cloud task eg: 133454565656767
console.log(`The cloud task is - ${cloudTask}`);
}
quickstart();
// Imports the Google Cloud Tasks Wrapper library.
const { CloudTaskWrapper } = require("cloud-task-wrapper");
async function quickstart() {
// TODO(developer): Uncomment these lines and replace with your values.
// const project = 'my-project-id';
// const queue = 'my-appengine-queue';
// const location = 'us-central1';
const config = {
project,
location,
};
const wrapper = new CloudTaskWrapper(config);
await wrapper.deleteCloudTaskByName(name, queue); //just the name of cloud task eg: 133454565656767
console.log(`Deleted the cloud task`);
}
quickstart();
const { CloudTaskWrapper } = require("cloud-task-wrapper");
async function quickstart() {
// TODO(developer): Uncomment these lines and replace with your values.
// const project = 'my-project-id';
// const queue = 'my-appengine-queue';
// const location = 'us-central1';
// const payload = 'hello';
const config = {
project,
queue,
location,
};
const wrapper = new CloudTaskWrapper(config);
const task = {
appEngineHttpRequest: {
httpMethod: "POST",
relativeUri: "/log_payload",
body: Buffer.from(payload).toString("base64")
},
scheduleTime = {
seconds: inSeconds + Date.now() / 1000, //inSeconds is the time for the task to be executed
};
};
// Send create task request.
const cloudTask = await wrapper.createCloudTask(task, queue, name); //name of cloud task is optional
console.log(`Created task ${cloudTask}`);
}
quickstart();
const { CloudTaskWrapper } = require("cloud-task-wrapper");
async function quickstart() {
// TODO(developer): Uncomment these lines and replace with your values.
// const project = 'my-project-id';
// const queue = 'my-appengine-queue';
// const location = 'us-central1';
// const payload = 'hello';
const config = {
project,
location,
};
const wrapper = new CloudTaskWrapper(config);
const task = {
httpRequest: {
httpMethod: "POST",
url: "https://ram-sankhavaram.com",
body: Buffer.from(payload).toString("base64"),
},
scheduleTime = {
seconds: inSeconds + Date.now() / 1000, //inSeconds is the time for the task to be executed
};
};
// Send create task request.
const cloudTask = await wrapper.createCloudTask(task, queue, name); //name of cloud task is optional
console.log(`Created task ${cloudTask}`);
}
quickstart();
FAQs
This package helps with utils that are not offered by @google-cloud/tasks
We found that cloud-task-wrapper demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.
Security News
Biden's executive order pushes for AI-driven cybersecurity, software supply chain transparency, and stronger protections for federal and open source systems.