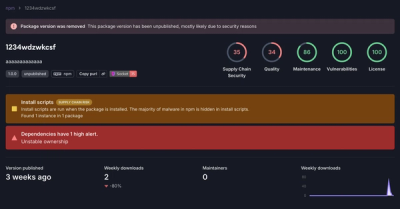
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
cloudevents
Advanced tools
The 'cloudevents' npm package is a library for working with CloudEvents, which are a specification for describing event data in a common way. This package allows you to create, validate, and serialize/deserialize CloudEvents, making it easier to work with event-driven architectures.
Create CloudEvent
This feature allows you to create a new CloudEvent instance with specified attributes such as type, source, and data.
const { CloudEvent } = require('cloudevents');
const myEvent = new CloudEvent({
type: 'com.example.someevent',
source: '/mycontext',
data: {
foo: 'bar'
}
});
console.log(myEvent);
Validate CloudEvent
This feature allows you to validate a CloudEvent instance to ensure it conforms to the CloudEvents specification.
const { CloudEvent, ValidationError } = require('cloudevents');
const myEvent = new CloudEvent({
type: 'com.example.someevent',
source: '/mycontext',
data: {
foo: 'bar'
}
});
try {
myEvent.validate();
console.log('Event is valid');
} catch (e) {
if (e instanceof ValidationError) {
console.error('Event is invalid:', e.errors);
}
}
Serialize CloudEvent
This feature allows you to serialize a CloudEvent instance to a JSON string, which can be useful for transmitting the event over a network.
const { CloudEvent } = require('cloudevents');
const myEvent = new CloudEvent({
type: 'com.example.someevent',
source: '/mycontext',
data: {
foo: 'bar'
}
});
const serializedEvent = myEvent.toString();
console.log(serializedEvent);
Deserialize CloudEvent
This feature allows you to deserialize a JSON string back into a CloudEvent instance, making it easier to work with received events.
const { CloudEvent } = require('cloudevents');
const serializedEvent = '{"type":"com.example.someevent","source":"/mycontext","data":{"foo":"bar"}}';
const myEvent = CloudEvent.parse(serializedEvent);
console.log(myEvent);
EventEmitter3 is a high-performance event emitter for Node.js and the browser. While it does not specifically adhere to the CloudEvents specification, it provides a robust way to handle events in an application.
Node EventStore Client is a client for EventStore, a database optimized for event sourcing. It provides more advanced features for event storage and retrieval but is more complex and specific to EventStore.
The AWS SDK for JavaScript provides a way to interact with AWS services, including event-driven services like AWS Lambda and Amazon EventBridge. It offers broader functionality but is specific to the AWS ecosystem.
The CloudEvents SDK for JavaScript.
Note: Supports CloudEvent versions 0.3, 1.0
The CloudEvents SDK requires a current LTS version of Node.js. At the moment those are Node.js 12.x, Node.js 14.x and Node.js 16.x. To install in your Node.js project:
npm install cloudevents
You can choose any popular web framework for port binding. A CloudEvent
object can be created by simply providing the HTTP
protocol binding
the incoming headers and request body.
const app = require("express")();
const { HTTP } = require("cloudevents");
app.post("/", (req, res) => {
// body and headers come from an incoming HTTP request, e.g. express.js
const receivedEvent = HTTP.toEvent({ headers: req.headers, body: req.body });
console.log(receivedEvent);
});
You can send events over HTTP in either binary or structured format
using the HTTP
binding to create a Message
which has properties
for headers
and body
.
const axios = require("axios").default;
const { HTTP, CloudEvent } = require("cloudevents");
const ce = new CloudEvent({ type, source, data });
const message = HTTP.binary(ce); // Or HTTP.structured(ce)
axios({
method: "post",
url: "...",
data: message.body,
headers: message.headers,
});
You may also use the emitterFor()
function as a convenience.
const axios = require("axios").default;
const { emitterFor, Mode, CloudEvent } = require("cloudevents");
function sendWithAxios(message) {
// Do what you need with the message headers
// and body in this function, then send the
// event
axios({
method: "post",
url: "...",
data: message.body,
headers: message.headers,
});
}
const emit = emitterFor(sendWithAxios, { mode: Mode.BINARY });
emit(new CloudEvent({ type, source, data }));
You may also use the Emitter
singleton
const axios = require("axios").default;
const { emitterFor, Mode, CloudEvent, Emitter } = require("cloudevents");
function sendWithAxios(message) {
// Do what you need with the message headers
// and body in this function, then send the
// event
axios({
method: "post",
url: "...",
data: message.body,
headers: message.headers,
});
}
const emit = emitterFor(sendWithAxios, { mode: Mode.BINARY });
// Set the emit
Emitter.on("cloudevent", emit);
...
// In any part of the code will send the event
new CloudEvent({ type, source, data }).emit();
// You can also have several listeners to send the event to several endpoints
All created CloudEvent
objects are read-only. If you need to update a property or add a new extension to an existing cloud event object, you can use the cloneWith
method. This will return a new CloudEvent
with any update or new properties. For example:
const {
CloudEvent,
} = require("cloudevents");
// Create a new CloudEvent
const ce = new CloudEvent({...});
// Add a new extension to an existing CloudEvent
const ce2 = ce.cloneWith({extension: "Value"});
You can create a CloudEvent
object in many ways, for example, in TypeScript:
import { CloudEvent, CloudEventV1, CloudEventV1Attributes } from "cloudevents";
const ce: CloudEventV1<string> = {
specversion: "1.0",
source: "/some/source",
type: "example",
id: "1234"
};
const event = new CloudEvent(ce);
const ce2: CloudEventV1Attributes<string> = {
specversion: "1.0",
source: "/some/source",
type: "example",
};
const event2 = new CloudEvent(ce2);
const event3 = new CloudEvent({
source: "/some/source",
type: "example",
});
There are a few trivial example applications in the examples folder. There you will find Express.js, TypeScript and Websocket examples.
Core Specification | v0.3 | v1.0 |
---|---|---|
CloudEvents Core | :heavy_check_mark: | :heavy_check_mark: |
Event Formats | v0.3 | v1.0 |
---|---|---|
AVRO Event Format | :x: | :x: |
JSON Event Format | :heavy_check_mark: | :heavy_check_mark: |
Protocol Bindings | v0.3 | v1.0 |
---|---|---|
AMQP Protocol Binding | :x: | :x: |
HTTP Protocol Binding | :heavy_check_mark: | :heavy_check_mark: |
Kafka Protocol Binding | :x: | :heavy_check_mark: |
MQTT Protocol Binding | :heavy_check_mark: | :x: |
NATS Protocol Binding | :x: | :x: |
Content Modes | v0.3 | v1.0 |
---|---|---|
HTTP Binary | :heavy_check_mark: | :heavy_check_mark: |
HTTP Structured | :heavy_check_mark: | :heavy_check_mark: |
HTTP Batch | :heavy_check_mark: | :heavy_check_mark: |
Kafka Binary | :heavy_check_mark: | :heavy_check_mark: |
Kafka Structured | :heavy_check_mark: | :heavy_check_mark: |
Kafka Batch | :heavy_check_mark: | :heavy_check_mark: |
MQTT Binary | :heavy_check_mark: | :heavy_check_mark: |
MQTT Structured | :heavy_check_mark: | :heavy_check_mark: |
We love contributions from the community! Please check the Contributor's Guide for information on how to get involved.
Each SDK may have its own unique processes, tooling and guidelines, common
governance related material can be found in the
CloudEvents community
directory. In particular, in there you will find information concerning
how SDK projects are
managed,
guidelines
for how PR reviews and approval, and our
Code of Conduct
information.
FAQs
CloudEvents SDK for JavaScript
The npm package cloudevents receives a total of 752,743 weekly downloads. As such, cloudevents popularity was classified as popular.
We found that cloudevents demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.