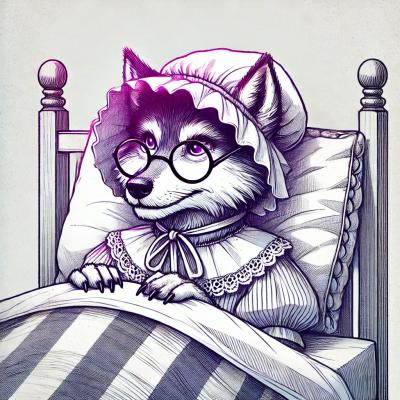
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
The coa npm package is a powerful command-line option parser that helps you build command-line applications by defining commands, options, and arguments in a declarative way. It provides a fluent API to make the definition of command-line interfaces easy and readable.
Command definition and execution
This code sample demonstrates how to define a command-line application with a version option and a subcommand. The application will display the version when the '--version' flag is used and will execute a subcommand when 'subcommand' is invoked.
const coa = require('coa');
coa.Cmd()
.name('app')
.title('My awesome command line app')
.helpful()
.opt()
.name('version')
.title('Show version')
.short('v')
.long('version')
.flag()
.act((opts) => console.log('v1.0.0'))
.end()
.cmd()
.name('subcommand')
.title('A subcommand example')
.helpful()
.act((opts, args) => console.log('Subcommand executed'))
.end()
.run(process.argv.slice(2));
Argument parsing
This code sample shows how to define a required argument for a command-line application. The application expects an input file as an argument and will print the name of the input file when the application is run.
const coa = require('coa');
coa.Cmd()
.name('app')
.title('My awesome command line app')
.helpful()
.arg()
.name('input')
.title('Input file')
.req()
.end()
.act((opts, args) => console.log(`Input file: ${args.input}`))
.run(process.argv.slice(2));
Option parsing
This code sample illustrates how to define an option for a command-line application. The application allows the user to specify an output file using either '-o' or '--output' flags and will print the name of the output file when the application is run.
const coa = require('coa');
coa.Cmd()
.name('app')
.title('My awesome command line app')
.helpful()
.opt()
.name('output')
.title('Output file')
.short('o')
.long('output')
.end()
.act((opts, args) => console.log(`Output file: ${opts.output}`))
.run(process.argv.slice(2));
Commander is a widely-used npm package for building command-line interfaces. It offers a similar declarative API for defining options and commands, and it is known for its simplicity and ease of use. Compared to coa, commander has a larger user base and more extensive documentation.
Yargs is another popular npm package for parsing command-line arguments. It provides a rich set of features for building interactive command-line tools, including advanced parsing, validation, and generating help messages. Yargs is often preferred for its fluent API and its support for subcommands, which is similar to coa but with more customization options.
Meow is a lighter alternative to coa, designed to be simple and minimalistic. It provides a straightforward way to parse command-line arguments and generate help text. While it lacks some of the advanced features of coa, such as the detailed command and option definitions, it is suitable for simpler command-line applications that do not require complex structures.
COA is a yet another parser for command line options. You can choose one of the existing modules, or write your own like me.
require('coa').Cmd() // main (top level) command declaration
.name(process.argv[1]) // set top level command name from program name
.title('My awesome command line util') // title for use in text messages
.helpful() // make command "helpful", i.e. options -h --help with usage message
.opt() // add some option
.name('version') // name for use in API
.title('Version') // title for use in text messages
.short('v') // short key: -v
.long('version') // long key: --version
.type(Boolean) // type Boolean for options without value
.act(function(opts) { // add action for option
this.exit( // exit program with code 0 and text message
JSON.parse(require('fs').readFileSync(__dirname + '/package.json'))
.version);
})
.end() // end option chain and return to main command
.cmd().name('subcommand').apply(require('./subcommand').COA).end() // load subcommand from module
.cmd() // inplace subcommand declaration
.name('othercommand').title('Awesome other subcommand').helpful()
.opt()
.name('input').title('input file, required')
.short('i').long('input')
.validate(function(v) { // validator function, also for translate simple values
return require('fs').createReadStream(v) })
.required() // make option required
.end() // end option chain and return to command
.end() // end subcommand chain and return to parent command
.parse(process.argv.slice(2)); // parse and run on process.argv
// subcommand.js
exports.COA = function() {
this
.title('Awesome subcommand').helpful()
.opt()
.name('output').title('output file')
.short('o').long('output')
.output() // use default preset for "output" option declaration
.end()
};
Command is a top level entity. Commands may have options and arguments.
Set a canonical command identifier to be used anywhere in the API.
@param String _name
command name
@returns COA.Cmd this
instance (for chainability)
Set a long description for command to be used anywhere in text messages.
@param String _title
command title
@returns COA.Cmd this
instance (for chainability)
Create new subcommand for current command.
@returns COA.Cmd new
subcommand instance
Create option for current command.
@returns COA.Opt new
option instance
Create argument for current command.
@returns COA.Opt new
argument instance
Add (or set) action for current command.
@param Function act
action function,
invoked in the context of command instance
and has the parameters:
- Object opts
parsed options
- Array args
parsed arguments
@param {Boolean} [force=false] flag for set action instead add to existings
@returns COA.Cmd this
instance (for chainability)
Apply function with arguments in context of command instance.
@param Function fn
@param Array args
@returns COA.Cmd this
instance (for chainability)
Make command "helpful", i.e. add -h --help flags for print usage.
@returns COA.Cmd this
instance (for chainability)
Terminate program with error code 1.
@param String msg
message for print to STDERR
@param {Object} [o] optional object for print with message
Terminate program with error code 0.
@param String msg
message for print to STDERR
Build full usage text for current command instance.
@returns String usage
text
Parse arguments from simple format like NodeJS process.argv.
@param Array argv
@returns COA.Cmd this
instance (for chainability)
Finish chain for current subcommand and return parent command instance.
@returns COA.Cmd parent
command
Option is a named entity. Options may have short and long keys for use from command line.
@namespace
@class Presents option
Set a canonical option identifier to be used anywhere in the API.
@param String _name
option name
@returns COA.Opt this
instance (for chainability)
Set a long description for option to be used anywhere in text messages.
@param String _title
option title
@returns COA.Opt this
instance (for chainability)
Set a short key for option to be used with one hyphen from command line.
@param String _short
@returns COA.Opt this
instance (for chainability)
Set a short key for option to be used with double hyphens from command line.
@param String _long
@returns COA.Opt this
instance (for chainability)
Set a type of option. Mainly using with Boolean for options without value.
@param Object _type
@returns COA.Opt this
instance (for chainability)
Makes an option accepts multiple values.
Otherwise, the value will be used by the latter passed.
@returns COA.Opt this
instance (for chainability)
Makes an option required.
@returns COA.Opt this
instance (for chainability)
Set a validation function for option.
Value from command line passes through before becoming available from API.
@param Function _validate
validating function,
invoked in the context of option instance
and has one parameter with value from command line
@returns COA.Opt this
instance (for chainability)
Set a default value for option.
Default value passed through validation function as ordinary value.
@param Object _def
@returns COA.Opt this
instance (for chainability)
Make option value outputing stream.
It's add useful validation and shortcut for STDOUT.
@returns COA.Opt this
instance (for chainability)
Add action for current option command.
This action is performed if the current option
is present in parsed options (with any value).
@param Function act
action function,
invoked in the context of command instance
and has the parameters:
- Object opts
parsed options
- Array args
parsed arguments
@returns COA.Opt this
instance (for chainability)
Finish chain for current option and return parent command instance.
@returns COA.Cmd parent
command
Argument is a unnamed entity.
From command line arguments passed as list of unnamed values.
Set a canonical argument identifier to be used anywhere in text messages.
@param String _name
argument name
@returns COA.Arg this
instance (for chainability)
Set a long description for argument to be used anywhere in text messages.
@param String _title
argument title
@returns COA.Arg this
instance (for chainability)
Makes an argument accepts multiple values.
Otherwise, the value will be used by the latter passed.
@returns COA.Arg this
instance (for chainability)
Makes an argument required.
@returns COA.Arg this
instance (for chainability)
Set a validation function for argument.
Value from command line passes through before becoming available from API.
@param Function _validate
validating function,
invoked in the context of argument instance
and has one parameter with value from command line
@returns COA.Arg this
instance (for chainability)
Set a default value for argument.
Default value passed through validation function as ordinary value.
@param Object _def
@returns COA.Arg this
instance (for chainability)
Make argument value outputing stream.
It's add useful validation and shortcut for STDOUT.
@returns COA.Arg this
instance (for chainability)
Finish chain for current option and return parent command instance.
@returns COA.Cmd parent
command
FAQs
Command-Option-Argument: Yet another parser for command line options.
The npm package coa receives a total of 5,295,392 weekly downloads. As such, coa popularity was classified as popular.
We found that coa demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.