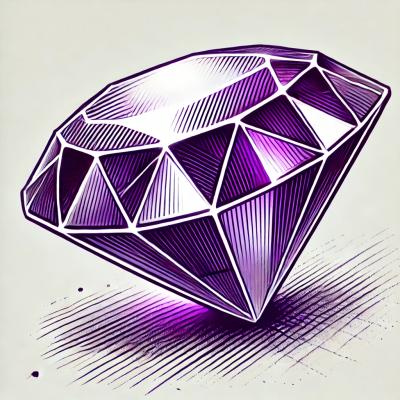
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
code-block-writer
Advanced tools
A simple code writer that assists with formatting and visualizing blocks of code.
The code-block-writer npm package is a utility for generating code with proper formatting, such as indentation and line breaks. It is particularly useful when writing code generators or transpilers that need to output human-readable code.
Writing code with automatic indentation and new lines
This feature allows you to write code blocks with automatic indentation management. The code sample demonstrates how to create a class with a constructor and a property.
const CodeBlockWriter = require('code-block-writer');
const writer = new CodeBlockWriter();
writer.writeLine('class MyClass {').indent(() => {
writer.writeLine('constructor() {').indent(() => {
writer.writeLine('this.myProperty = 1;');
}).writeLine('}');
}).writeLine('}');
console.log(writer.toString());
Conditional writing
This feature enables writing parts of the code conditionally. In the code sample, 'someCondition' is only written if the 'condition' is true.
const writer = new CodeBlockWriter();
const condition = true;
writer.write('if (').conditionalWrite(condition, 'someCondition').writeLine(') {').indent(() => {
writer.writeLine('doSomething();');
}).writeLine('}');
console.log(writer.toString());
Block indentation
This feature allows for easy indentation of entire blocks of code. The code sample shows how to write a function with multiple lines inside its body, all properly indented.
const writer = new CodeBlockWriter();
writer.writeLine('function myFunction() {').indentBlock(() => {
writer.writeLine('let x = 10;').writeLine('return x;');
}).writeLine('}');
console.log(writer.toString());
Prettier is an opinionated code formatter that supports many languages and integrates with most editors. Unlike code-block-writer, which is used for generating code, Prettier formats existing code according to its style rules.
js-beautify is a package that can format HTML, CSS, and JavaScript. It is similar to Prettier in that it formats existing code. It is not specifically designed for code generation like code-block-writer.
Recast is a JavaScript AST tool that allows you to parse your code, modify it, and then generate new code. It is more complex than code-block-writer as it deals with abstract syntax trees, but it can be used for similar code generation tasks.
A simple code writer that assists with formatting and visualizing blocks of JavaScript or TypeScript code.
npm install --save code-block-writer
import CodeBlockWriter from "code-block-writer";
const writer = new CodeBlockWriter({
// optional options
newLine: "\r\n", // default: "\n"
indentNumberOfSpaces: 2, // default: 4
useTabs: false // default: false
});
const className = "MyClass";
writer.write(`class ${className} extends OtherClass`).block(() => {
writer.writeLine(`@MyDecorator("myArgument1", "myArgument2")`);
writer.write(`myMethod(myParam: any)`).block(() => {
writer.write(`return this.post("myArgument");`);
});
});
console.log(writer.toString());
Outputs:
class MyClass extends OtherClass {
@MyDecorator("myArgument1", "myArgument2")
myMethod(myParam: any) {
return this.post("myArgument");
}
}
block(block: () => void)
- Indents all the code written within and surrounds it in braces.inlineBlock(block: () => void)
- Same as block, but doesn't add a space before the first brace and doesn't add a newline at the end.getLength()
- Get the current number of characters.writeLine(str: string)
- Writes some text and adds a newline.newLineIfLastNotNewLine()
- Writes a newline if what was written last wasn't a newline.newLine()
- Writes a newline.blankLine()
- Writes a blank line. Does not allow consecutive blank lines.indent()
- Indents the current line.spaceIfLastNotSpace()
- Writes a space if the last was not a space.write(str: string)
- Writes some text.conditionalNewLine(condition: boolean)
- Writes a newline if the condition is matched.conditionalWrite(condition: boolean, str: string)
- Writes if the condition is matched.conditionalWriteLine(condition: boolean, str: string)
- Writes some text and adds a newline if the condition is matched.setIndentationLevel(indentationLevel: number)
- Sets the current indentation level.isInComment()
- Gets if the writer is currently in a comment.isInString()
- Gets if the writer is currently in a string.toString()
- Gets the string.FAQs
A simple code writer that assists with formatting and visualizing blocks of code.
The npm package code-block-writer receives a total of 622,935 weekly downloads. As such, code-block-writer popularity was classified as popular.
We found that code-block-writer demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.