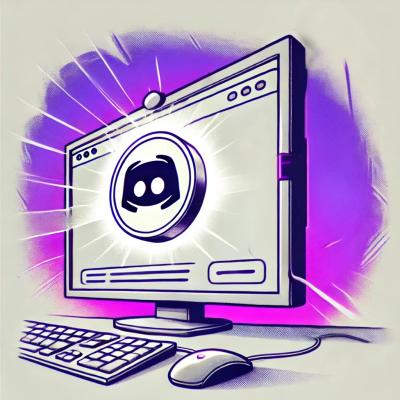
Research
Security News
Malicious PyPI Package ‘pycord-self’ Targets Discord Developers with Token Theft and Backdoor Exploit
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
codemod-missing-await-act
Advanced tools
Adds missing `await` to `act` calls or methods that contain an `act` call using [jscodeshift](https://github.com/facebook/jscodeshift). The codemod propagates these changes throughout the file. For example, given
Adds missing await
to act
calls or methods that contain an act
call using jscodeshift.
The codemod propagates these changes throughout the file.
For example, given
import { act } from "react";
function focus(element) {
act(() => {
element.focus();
});
}
test("focusing", () => {
const { container } = render("<button />");
focus(container);
});
will add an await
to act
and also add await
to focus
since focus
is now an async method.
The end result will be
import { act } from "react";
async function focus(element) {
await act(() => {
element.focus();
});
}
test("focusing", async () => {
const { container } = await render("<button />");
await focus(container);
});
Right now we assume that any call to rerender
and unmount
should be awaited.
$ npx codemod-missing-await-act ./src
Processing 4 files...
All done.
Results:
0 errors
3 unmodified
0 skipped
1 ok
Time elapsed: 0.428seconds
$ npx codemod-missing-await-act
codemod-missing-await-act <paths...>
Positionals:
paths [string] [required]
Options:
--version Show version number [boolean]
--help Show help [boolean]
--dry [boolean] [default: false]
--ignore-pattern [string] [default: "**/node_modules/**"]
--import-config A path to a JS file importing all methods whose calls should
be awaited. [string]
--verbose [boolean] [default: false]
Examples:
codemod-missing-await-act ./ Ignores `node_modules` and `build`
--ignore-pattern folders
"**/{node_modules,build}/**"
codemod-missing-await-act ./ Adds await to to all calls of
--import-confg methods imported in that file.
./missing-await-import-config.js
When a newly async function is exported, the codemod will not automatically update all references. However, the codemod summarizes at the end which files are impacted and will generate an import config that can be used to update the remaining references if these are imported via relative imports.
// codemod-missing-await/default-import-config.js
// Import aliases have no effect on the codemod.
// They're only used to not cause JS Syntax errors in this file.
// The codemod will only consider the imported name.
import {
act,
cleanup,
/**
* @includeMemberCalls
* e.g. fireEvent.click()
*/
fireEvent,
render,
renderHook,
} from "@testing-library/react";
import {
act as act2,
cleanup as cleanup2,
/**
* @includeMemberCalls
* e.g. fireEvent.click()
*/
fireEvent as fireEvent2,
render as render2,
renderHook as renderHook2,
} from "@testing-library/react/pure";
import {
act as act3,
cleanup as cleanup3,
/**
* @includeMemberCalls
* e.g. fireEvent.click()
*/
fireEvent as fireEvent3,
render as render3,
renderHook as renderHook3,
} from "@testing-library/react-native";
import {
act as act4,
cleanup as cleanup4,
/**
* @includeMemberCalls
* e.g. fireEvent.click()
*/
fireEvent as fireEvent4,
render as render4,
renderHook as renderHook4,
} from "@testing-library/react-native/pure";
import { unstable_act } from "react";
import { act as ReactTestUtilsAct } from "react-dom/test-utils";
import { act as ReactTestRendererAct } from "react-test-renderer";
For example, when we transform this ~/src/utils.js
file
export function hoverAndClick(element) {
fireEvent.mouseEnter(element);
fireEvent.click(element);
}
hoverAndClick
will now be async.
The codemod generates an import config that will look something like this
import { hoverAndClick as hoverAndClick1 } from "file:///Users/you/repo/src/utils.js";
You can then run the codemod again with the --import-config
option.
The codemod will now also await hoverAndClick
if utils.js
is imported via a relative path.
import { render } from "@testing-library/react";
import { hoverAndClick } from "./utils";
test("hover and click", () => {
const { container } = render("<button />");
hoverAndClick(container);
});
will be transformed into
import { render } from "@testing-library/react";
import { hoverAndClick } from "./utils";
test("hover and click", async () => {
const { container } = await render("<button />");
await hoverAndClick(container);
});
You need to repeat this process until the codemod no longer prompts you at the end to update the import config.
If you use path aliases or modules with newly async exports are imported via package specifiers, you need to manually adjust the import config e.g.
import { hoverAndClick as hoverAndClick1 } from "file:///Users/you/repo/src/utils.js";
import { hoverAndClick as hoverAndClick2 } from "@my/module";
const acting = act(scope);
await anotherPromise;
await acting;
will add await
to the act
call.
This codemod is targetted at act calls.
act()
calls are not allowed to overlap anyway so the original code was already problematic since acting
could also contain an act
call.
We don't track references to class methods. Only references to (arrow-) function declarations or expressions are tracked.
class MyTestUtils {
render() {
act(scope);
}
}
const utils = new MyTestUtils();
utils.render();
will be transformed to
class MyTestUtils {
async render() {
await act(scope);
}
}
const utils = new MyTestUtils();
// no `await` added
utils.render();
The following list contains officially supported runtimes. Please file an issue for runtimes that are not included in this list.
18.x || 20.x || 22.x || 23.x
0.3.0
#48 5898881
Thanks @eps1lon! - Add support for propagating through relative paths
The import config (from --import-config-path
) can now contain imports to absolute
paths prefixed as file URLs.
File URLs will be matched against relative imports.
Given this import config
import { render as render1 } from "file:///root/relative-paths/utils.js";
the import import { render } from './utils'
in /root/index.test.js
will be
considered as newly async and each call of render
will be awaited.
#49 00f35d5
Thanks @eps1lon! - Summarize follow-up items when codemod finished
When newly async functions are exported, the codemod will not automatically update all references. However, we now summarize at the end which files are impacted and generate an import config that can be used to update the remaining references if these are imported via relative imports. However, if these exports are imported via package specifiers or other path aliases, users need to manually adjust the import sources which is explained in the README.
#26 c74e8af
Thanks @eps1lon! - Ensure different import configs can be used during module lifetime
#32 eaefca3
Thanks @eps1lon! - Update Node.js support matrix
Codemod officially supports all currently Node.js versions listed in https://nodejs.org/en/about/previous-releases#release-schedule.
#30 25492dd
Thanks @eps1lon! - Add support for files using import assertions
E.g. import manifest from './package.json' assert { type: 'json' }
FAQs
Adds missing `await` to `act` calls or methods that contain an `act` call using [jscodeshift](https://github.com/facebook/jscodeshift). The codemod propagates these changes throughout the file. For example, given
The npm package codemod-missing-await-act receives a total of 3 weekly downloads. As such, codemod-missing-await-act popularity was classified as not popular.
We found that codemod-missing-await-act demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.
Security News
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.