colorful-chalk-logger
Advanced tools
Comparing version 0.1.0 to 0.2.0
@@ -26,3 +26,3 @@ "use strict"; | ||
const negate_pattern = /^(no-)?(\S+)$/; | ||
const valid_flag = /^date|colorful$/; | ||
const valid_flag = /^date|colorful|inline$/; | ||
let flags = program.logFlag; | ||
@@ -29,0 +29,0 @@ for (let f of flags) { |
@@ -8,1 +8,2 @@ import { Chalk } from 'chalk'; | ||
} | ||
export declare function colorToChalk(color: Color, fg: boolean): any; |
@@ -49,1 +49,2 @@ "use strict"; | ||
} | ||
exports.colorToChalk = colorToChalk; |
@@ -0,1 +1,3 @@ | ||
import { Chalk } from 'chalk'; | ||
import { Color } from './colors'; | ||
import { Level } from './level'; | ||
@@ -5,11 +7,19 @@ export interface Options { | ||
date?: boolean; | ||
inline?: boolean; | ||
colorful?: boolean; | ||
dateChalk?: Chalk | Color; | ||
nameChalk?: Chalk | Color; | ||
} | ||
export declare class Logger { | ||
private static readonly defaultLevel; | ||
private static readonly defaultDateChalk; | ||
private static readonly defaultNameChalk; | ||
private readonly write; | ||
readonly name: string; | ||
readonly level: Level; | ||
readonly dateChalk: any; | ||
readonly nameChalk: any; | ||
readonly flags: { | ||
date: boolean; | ||
inline: boolean; | ||
colorful: boolean; | ||
@@ -16,0 +26,0 @@ }; |
"use strict"; | ||
Object.defineProperty(exports, "__esModule", { value: true }); | ||
const util = require("util"); | ||
const chalk_1 = require("chalk"); | ||
const colors_1 = require("./colors"); | ||
const level_1 = require("./level"); | ||
const chalk_1 = require("chalk"); | ||
class Logger { | ||
@@ -10,4 +11,7 @@ constructor(name, options) { | ||
this.level = Logger.defaultLevel; | ||
this.dateChalk = Logger.defaultDateChalk; | ||
this.nameChalk = Logger.defaultNameChalk; | ||
this.flags = { | ||
date: false, | ||
inline: false, | ||
colorful: true, | ||
@@ -18,7 +22,21 @@ }; | ||
return; | ||
let { level, date, colorful, } = options; | ||
let { level, dateChalk, nameChalk, date, inline, colorful, } = options; | ||
if (level) | ||
this.level = level; | ||
if (dateChalk) { | ||
if (typeof dateChalk === 'function') | ||
this.dateChalk = dateChalk; | ||
else | ||
this.dateChalk = colors_1.colorToChalk(dateChalk, true); | ||
} | ||
if (nameChalk) { | ||
if (typeof nameChalk === 'function') | ||
this.nameChalk = nameChalk; | ||
else | ||
this.nameChalk = colors_1.colorToChalk(nameChalk, true); | ||
} | ||
if (date != null) | ||
this.flags.date = date; | ||
if (inline != null) | ||
this.flags.inline = inline; | ||
if (colorful != null) | ||
@@ -28,2 +46,4 @@ this.flags.colorful = colorful; | ||
static get defaultLevel() { return level_1.WARN; } | ||
static get defaultDateChalk() { return chalk_1.default.gray.bind(chalk_1.default); } | ||
static get defaultNameChalk() { return chalk_1.default.gray.bind(chalk_1.default); } | ||
format(level, header, message) { | ||
@@ -38,7 +58,7 @@ if (this.flags.colorful) { | ||
let { desc } = level; | ||
let { name } = this; | ||
let { name, dateChalk, nameChalk } = this; | ||
if (this.flags.colorful) { | ||
desc = level.headerChalk.fg(desc); | ||
desc = level.headerChalk.bg(desc); | ||
name = chalk_1.default.gray(name); | ||
name = nameChalk(name); | ||
} | ||
@@ -50,3 +70,3 @@ let header = `${desc} ${name}`; | ||
if (this.flags.colorful) | ||
dateString = chalk_1.default.gray(dateString); | ||
dateString = dateChalk(dateString); | ||
return `${dateString} [${header}]`; | ||
@@ -56,2 +76,3 @@ } | ||
let text; | ||
let { inline } = this.flags; | ||
switch (typeof message) { | ||
@@ -63,5 +84,11 @@ case 'boolean': | ||
break; | ||
case 'function': | ||
text = message.toString(); | ||
break; | ||
default: | ||
try { | ||
text = JSON.stringify(message, null, 2); | ||
if (inline) | ||
text = util.inspect(message, false, null); | ||
else | ||
text = JSON.stringify(message, null, 2); | ||
} | ||
@@ -72,3 +99,5 @@ catch (error) { | ||
} | ||
if (text.indexOf('\n') > -1) | ||
if (inline) | ||
text = text.replace(/\n\s*/g, ' '); | ||
if (!inline && text.indexOf('\n') > -1) | ||
text += '\n'; | ||
@@ -75,0 +104,0 @@ else |
{ | ||
"name": "colorful-chalk-logger", | ||
"version": "0.1.0", | ||
"version": "0.2.0", | ||
"description": "a colorful logger, support control output(format | level) through command-line options.", | ||
@@ -5,0 +5,0 @@ "main": "dist/index.js", |
@@ -12,2 +12,10 @@ `colorful-chalk-logger` is a colorful logger tool based on ***[chalk](https://github.com/chalk/chalk)***(so you can use a lot of colors) and ***[commander](https://github.com/tj/commander.js)***(so you can use command line parameters to customized the logger's behavior). | ||
# cli-options | ||
* `--log-level <debug|verbose|info|warn|error|fatal>`: index global logger level. | ||
* `--log-flag <[no-](date|inline|colorful)>`: the prefix `no-` represent negation. | ||
> - `date`: whether to print date. | ||
> - `inline`: each log record output in one line. | ||
> - `colorful`: whether to print with colors. | ||
# Example | ||
@@ -74,1 +82,26 @@ ```typescript | ||
***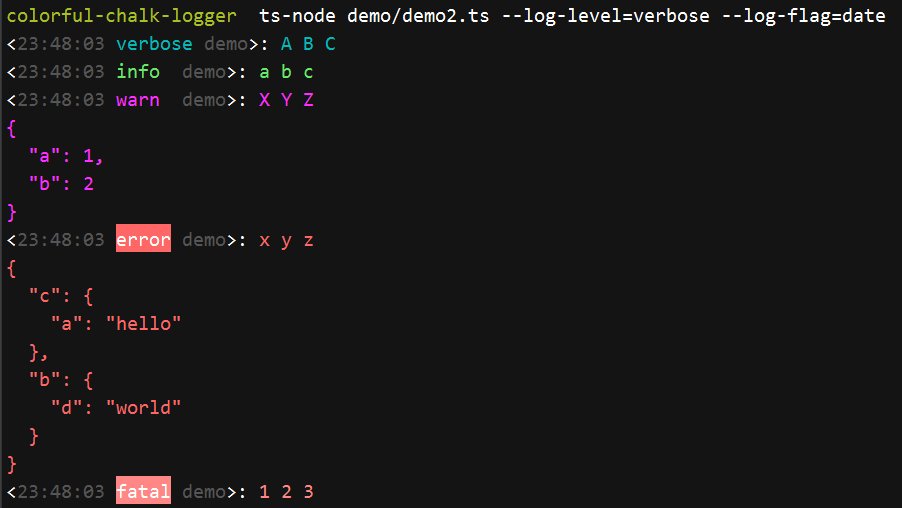*** | ||
```typescript | ||
// demo/demo3.ts | ||
import { ColorfulChalkLogger, ERROR } from '../index' | ||
import { Level } from '../src/level' | ||
import chalk from 'chalk' | ||
let logger = new ColorfulChalkLogger('demo', { | ||
level: ERROR, // the default value is DEBUG | ||
date: false, // the default value is false. | ||
colorful: true, // the default value is true. | ||
dateChalk: 'green', | ||
nameChalk: chalk.cyan.bind(chalk), | ||
}) | ||
logger.debug('A', 'B', 'C') | ||
logger.verbose('A', 'B', 'C') | ||
logger.info('a', 'b', 'c') | ||
logger.warn('X', 'Y', 'Z', { a: 1, b: 2}) | ||
logger.error('x', 'y', 'z', { c: { a: 'hello' }, b: { d: 'world' } }) | ||
logger.fatal('1', '2', '3') | ||
``` | ||
***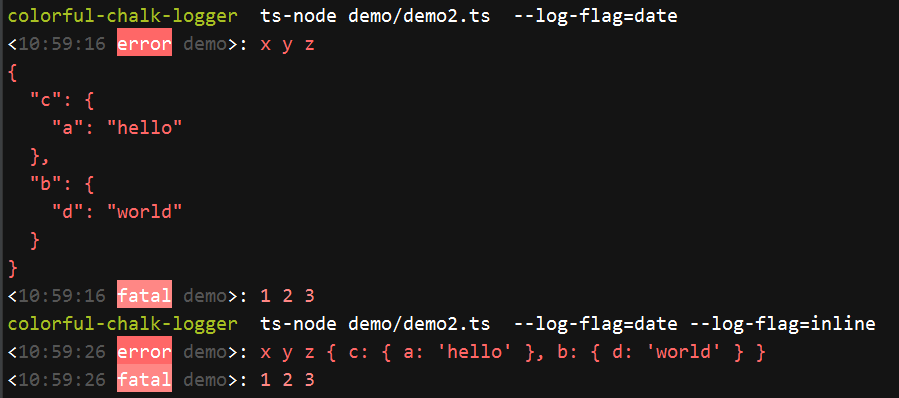*** |
104930
15
321
106