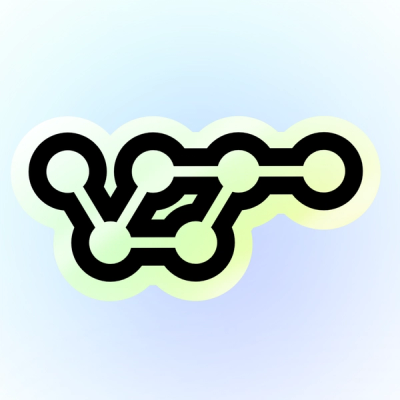
Security News
vlt Debuts New JavaScript Package Manager and Serverless Registry at NodeConf EU
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
command-line-usage
Advanced tools
The command-line-usage npm package is used to generate usage guides for command-line applications. It helps in creating structured and styled help text that can be displayed when users need assistance with the command-line interface (CLI) of your application.
Defining Sections
This feature allows you to define different sections of the usage guide, such as headers and options. The code sample demonstrates how to create a usage guide with a description and options.
const commandLineUsage = require('command-line-usage');
const sections = [
{
header: 'My App',
content: 'This is a description of my app.'
},
{
header: 'Options',
optionList: [
{
name: 'help',
typeLabel: '{underline boolean}',
description: 'Display this usage guide.'
},
{
name: 'src',
typeLabel: '{underline file}',
description: 'The input file to process.'
}
]
}
];
const usage = commandLineUsage(sections);
console.log(usage);
Customizing Option Descriptions
This feature allows you to customize the descriptions of the options in your CLI application. The code sample shows how to define options with aliases and types, along with their descriptions.
const commandLineUsage = require('command-line-usage');
const sections = [
{
header: 'Options',
optionList: [
{
name: 'verbose',
alias: 'v',
type: Boolean,
description: 'Enable verbose mode.'
},
{
name: 'timeout',
alias: 't',
type: Number,
description: 'Set the timeout value in ms.'
}
]
}
];
const usage = commandLineUsage(sections);
console.log(usage);
Adding Examples
This feature allows you to add examples to your usage guide, making it easier for users to understand how to use your CLI application. The code sample demonstrates how to add concise and detailed examples.
const commandLineUsage = require('command-line-usage');
const sections = [
{
header: 'Examples',
content: [
{
desc: '1. A concise example.',
example: '$ app -v'
},
{
desc: '2. A long example.',
example: '$ app --timeout 1000'
}
]
}
];
const usage = commandLineUsage(sections);
console.log(usage);
Yargs is a popular library for building command-line interfaces. It provides extensive functionality for parsing arguments, generating usage information, and handling commands. Compared to command-line-usage, yargs offers more comprehensive features for argument parsing and command handling.
Commander is another widely-used library for building command-line interfaces. It allows you to define commands, options, and usage information. While it provides similar functionality to command-line-usage, it is more focused on command and option parsing rather than generating usage guides.
Oclif is a framework for building command-line tools. It provides a robust set of features for creating complex CLI applications, including argument parsing, command handling, and generating help text. Oclif is more comprehensive and suited for larger CLI projects compared to command-line-usage.
A simple template to create a usage guide. It was extracted from command-line-args to faciliate arbitrary use.
var getUsage = require("command-line-usage");
var usage = getUsage(definitions, options)
Inline ansi formatting can be used anywhere within the usage template using the formatting syntax described here.
A description
field is added to each option definition. A title
, description
and simple footer
are set in the getUsage options. Code.
Demonstrates breaking the options up into groups. This example also sets a typeLabel
on each option definition (e.g. a typeLabel
value of files
is more meaningful than the default string[]
). Code.
Here, the title
is replaced with a header
banner. This example also adds a synopsis
list. Code.
The footer is displayed at the end of the template. Code.
A list of examples
is added. In this case the example list is defined as an array of objects (each with consistently named properties) so will be formatted by column-layout. Code.
Demonstrates usage of custom column layout in the description. In this case the second column (containing the hammer and sickle) has nowrap
enabled, as the input is already formatted as desired. Code.
Demonstrates a custom template. The getUsage.optionList()
method exists for users that want the option list and nothing else. Code.
string
⏏
Array.<string>
string
⏏Kind: Exported function
Param | Type | Description |
---|---|---|
definitions | Array.<optionDefinition> | an array of option definition objects. In addition to the regular definition properties, command-line-usage will look for:
|
options | usage-options | see UsageOptions. |
Array.<string>
A helper for getting a column-format list of options and descriptions. Useful for inserting into a custom usage template.
Kind: static method of getUsage
Param | Type | Description |
---|---|---|
definitions | Array.<optionDefinition> | the definitions to Display |
[group] | string | if specified, will output the options in this group. The special group |
The class describes all valid options for the getUsage
function. Inline formatting can be used within any text string supplied using valid ansi-escape-sequences formatting syntax.
Kind: Exported class
textBlock
Use this field to display a banner or header above the main body.
Kind: instance property of UsageOptions
string
The title line at the top of the usage, typically the name of the app. By default it is underlined but this formatting can be overridden by passing a module:usage-options~textObject.
Kind: instance property of UsageOptions
Example
{ title: "my-app" }
textBlock
A description to go underneath the title. For example, some words about what the app is for.
Kind: instance property of UsageOptions
textBlock
An array of strings highlighting the main usage forms of the app.
Kind: instance property of UsageOptions
object
Specify which groups to display in the output by supplying an object of key/value pairs, where the key is the name of the group to include and the value is a string or textObject. If the value is a string it is used as the group title. Alternatively supply an object containing a title
and description
string.
Kind: instance property of UsageOptions
Example
{
main: {
title: "Main options",
description: "This group contains the most important options."
},
misc: "Miscellaneous"
}
textBlock
Examples
Kind: instance property of UsageOptions
textBlock
Displayed at the foot of the usage output.
Kind: instance property of UsageOptions
Example
{
footer: "Project home: [underline]{https://github.com/me/my-app}"
}
string
| Array.<string>
If you want to hide certain options from the output, specify their names here. This is sometimes used to hide the defaultOption
.
Kind: instance property of UsageOptions
Example
{
hide: "files"
}
string
| Array.<string>
| Array.<object>
| Object
A text block can be a string:
{
description: 'This is a single-line description.'
}
.. or multiple strings:
{
description: [
'This is a multi-line description.',
'A new string in the array represents a new line.'
]
}
.. or an array of objects. In which case, it will be formatted by column-layout:
{
description: {
column1: 'This will go in column 1.',
column2: 'Second column text.'
}
}
If you want set specific column-layout options, pass an object with two properties: options
and data
.
{
description: {
options: {
columns: [
{ name: 'two', width: 40, nowrap: true }
]
},
data: {
column1: 'This will go in column 1.',
column2: 'Second column text.'
}
}
}
Kind: inner typedef of UsageOptions
© 2015 Lloyd Brookes <75pound@gmail.com>. Documented by jsdoc-to-markdown.
FAQs
Generates command-line usage information
The npm package command-line-usage receives a total of 1,083,425 weekly downloads. As such, command-line-usage popularity was classified as popular.
We found that command-line-usage demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
Security News
Research
The Socket Research Team uncovered a malicious Python package typosquatting the popular 'fabric' SSH library, silently exfiltrating AWS credentials from unsuspecting developers.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.