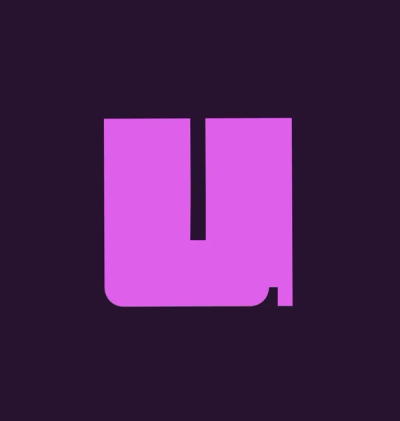
Product
Socket Now Supports uv.lock Files
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
compact-encoding
Advanced tools
A series of compact encoding schemes for building small and fast parsers and serializers
A series of compact encoding schemes for building small and fast parsers and serializers
npm install compact-encoding
const cenc = require('compact-encoding')
const state = { start: 0, end: 0, buffer: null }
// use preencode to figure out how big a buffer is needed
cenc.uint.preencode(state, 42)
cenc.string.preencode(state, 'hi')
console.log(state) // { start: 0, end: 4, buffer: null }
state.buffer = Buffer.allocUnsafe(state.end)
// then use encode to actually encode it to the buffer
cenc.uint.encode(state, 42)
cenc.string.encode(state, 'hi')
// to decode it simply use decode instead
state.start = 0
cenc.uint.decode(state) // 42
cenc.string.decode(state) // 'hi'
state
Should be an object that looks like this { start, end, buffer }
.
You can also get a blank state object using cenc.state()
.
enc.preencode(state, val)
Does a fast preencode dry-run that only sets state.end. Use this to figure out how big of a buffer you need.
enc.encode(state, val)
Encodes val
into state.buffer
at position state.start
.
Updates state.start
to point after the encoded value when done.
val = enc.decode(state)
Decodes a value from state.buffer
as position state.start
.
Updates state.start
to point after the decoded value when done in the buffer.
If you are just encoding to a buffer or decoding from one you can use the encode
and decode
helpers
to reduce your boilerplate
const buf = cenc.encode(cenc.bool, true)
const bool = cenc.decode(cenc.bool, buf)
The following encodings are bundled as they are primitives that can be used to build others on top. Feel free to PR more that are missing.
cenc.uint
- Encodes a uint using compact-uintcenc.int
- Encodes an int using compact-uint as a signed int using ZigZag encoding.cenc.uint16
- Encodes a fixed size uint16 (useful for things like ports)cenc.uint24
- Encodes a fixed size uint24 (useful for message framing)cenc.uint32
- Encodes a fixes size uint32 (useful for very large message framing)cenc.buffer
- Encodes a buffer with it's length uint prefixed. When decoding an empty buf, null is returned.cenc.raw
- Pass through encodes a buffer - ie a basic copy.cenc.uint32array
- Encodes a uint32array with it's element length uint32 prefixed.cenc.bool
- Encodes a boolean as 1 or 0.cenc.string
- Encodes a utf-8 string, similar to buffer.cenc.fixed32
- Encodes a fixed 32 byte buffer.cenc.fixed64
- Encodes a fixed 64 byte buffer.cenc.fixed(n)
- Makes a fixed sized encoder.cenc.array(enc)
- Makes an array encoder from another encoder. Arrays are uint prefixed with their length.cenc.from(enc)
- Makes a compact encoder from a codec or abstract-encodingMIT
FAQs
A series of compact encoding schemes for building small and fast parsers and serializers
The npm package compact-encoding receives a total of 1,599 weekly downloads. As such, compact-encoding popularity was classified as popular.
We found that compact-encoding demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.