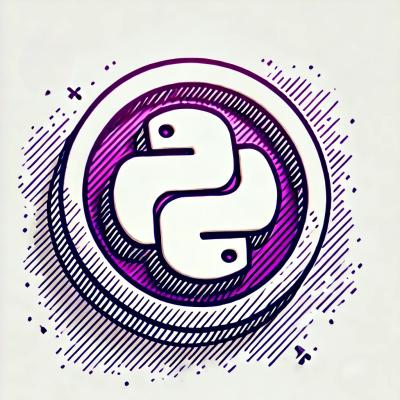
Security News
PyPI Now Supports iOS and Android Wheels for Mobile Python Development
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
component-hindawi-ui
Advanced tools
Utility HOCs
HOCs used for files drag and drop
Utility functions
Injects countries
and countryLabel
as props.
Name | Type | Description |
---|---|---|
countries | [{value: string, label: string}] | the list of countries |
countryLabel | (code: string) => string | get the name of the country with the specified code |
import { Menu } from '@pubsweet/ui'
import { withCountries } from 'component-hindawi-ui'
const Wrapped = ({ countries, countryLabel }) => (
<div>
<Menu options={countries} placeholder="Select a country" />
<span>Selected country: {countryLabel('RO')}</span>
</div>
)
export default withCountries(Wrapped)
Injects isFetching
, fetchingError
, setFetching
, toggleFetching
, setError
and clearError
as props.
Name | Type | Description |
---|---|---|
isFetching | bool | Pending async operation sattus |
fetchingError | fetchingError | Value representing the error |
setFetching | (value: bool) => any | Function for setting the isFetching value |
toggleFetching | (value: bool) => any | Function that toggles the current value of isFetching |
setError | (error: string) => any | Function that sets fetchingError |
clearError | () => any | Function that resets fetchingError to it's original value |
import { withFetching } from 'component-hindawi-ui'
const Wrapped = ({ isFetching, fetchingError, setFetching, toggleFetching }) => (
<div>
{isFetching && <span>I am fetching</span>}
<span>{`The error: ${fetchingError}`</span>
<button onClick={() => setFetching(true)}>Set fetching true</button>
<button onClick={() => setFetching(false)}>Set fetching false</button>
<button onClick={toggleFetching}>Toggle fetching</button>
</div>
)
export default withFetching(Wrapped)
Generate a securized file URL and preview it in a new tab. Injects previewFile
as a prop.
This HOC assumes the following props are present on the wrapped component:
Name | Type | Description |
---|---|---|
getSignedURL | (id: string) => Promise({signedURL: string}) | Async call that returns the securized S3 file url |
Name | Type | Description |
---|---|---|
previewFile | (file: {id: string, ...}) => any | Opens the file preview in a new tab (only possible for PDF files and images) |
import { withProps } from 'recompose'
import { FileItem, withFilePreview Wrapped} from 'component-hindawi-ui'
const file = {
id: 'myfile',
name: 'myfile.pdf',
size: 100231,
}
const Wrapped = ({ previewFile }) => (
<div>
<FileItem item={file} onPreview={previewFile} />
</div>
)
export default withFilePreview(Wrapped)
Injects page
, itemsPerPage
, toFirst
, nextPage
, toLast
, prevPage
, changeItemsPerPage
, hasMore
, maxItems
and paginatedItems
as props.
Name | Type | Description |
---|---|---|
page | number | Current page. |
itemsPerPage | number | Number of items to be shown per page. |
maxItems | number | Total number of items. |
maxItems | number | Total number of items. |
hasMore | bool | If we're not at the last page yet. |
paginatedItems | [any] | Slice of the original items. |
toFirst | () => { page: number } | Go to the first page. |
toLast | () => { page: number } | Go to the last page. |
nextPage | () => { page: number } | Move to the next page. |
prevPage | () => { page: number } | Move to the previous page. |
changeItemsPerPage | e: HTMLInputEvent => {page: number, itemsPerPage: number} | Change the number of items per page. |
import { withPagination } from 'component-hindawi-ui'
const Wrapped = ({ page, nextPage, prevPage, paginatedItems, changeItemsPerPage }) => (
<div>
<span>Page {page}</span>
<button onClick={prevPage}>Prev page</button>
<button onClick={nextPage}>Next page</button>
<input type="text" onChange={changeItemsPerPage} />
<div>
{
paginatedItems.map(p => <span>{p}<span>)
}
</div>
</div>
)
export default withPagination(Wrapped)
Injects the roles
array as a prop. The roles are parsed from the journal config files.
Name | Type | Description |
---|---|---|
roles | [{value: string, label: string}] | An array of user roles. |
import { Menu } from '@pubsweet/ui'
import { withRoles } from 'component-hindawi-ui'
const Wrapped = ({ roles }) => <Menu options={roles} />
export default withRoles(Wrapped)
HOC used to provide drop functionality to the FileSection
component. It's main purpose is to change a file from one list to another. This is usually done in a callback called changeList
that should be provided to the wrapped component.
This HOC assumes the wrapped component has the following props:
Name | Type | Description |
---|---|---|
files | [{id: string, ...}] | List of files passed to the wrapped component. |
setError | (error: string) => any | Error setting callback. |
listId | string | Current list id. |
allowedFileExtensions | [string] | Allowed file types. |
maxFiles | number | Maximum number of files allowed. |
changeList | (fromListId: string, toListId: string: fileId: string) => any | Callback fired when moving the file to a new list. |
import { compose, withHandler, withProps } from 'recompose'
import { FileSection, withFileSectionDrop } from 'component-hindawi-ui'
const Wrapped = compose(
withProps({
files: [...],
listId: 'CoverLetter',
maxFiles: 3,
allowedFileExtensions: ['pdf'],
}),
withHandlers({
changeList: () => (fromListId, toListId, fileId) => {
// do the actual change here
}
}),
withFileSectionDrop,
)(FileSection)
export default Wrapped
HOC used to provide native file drop functionality to the FileSection
component. It's purpose is to do something when dragging files from the computer's hard drive into the app. This HOC allows only single items! Dragging multiple items into the wrapped component will only handle the first item!
This HOC assumes the wrapped component has the following props:
Name | Type | Description |
---|---|---|
files | [{id: string, ...}] | List of files passed to the wrapped component. |
setError | (error: string) => any | Error setting callback. |
allowedFileExtensions | [string] | Allowed file types. |
maxFiles | number | Maximum number of files allowed. |
onFileDrop | (file: File) => any | Callback fired when a valid file is dropped. |
import { compose, withHandler, withProps } from 'recompose'
import { FileSection, withNativeFileDrop } from 'component-hindawi-ui'
const Wrapped = compose(
withProps({
files: [...],
listId: 'CoverLetter',
maxFiles: 3,
allowedFileExtensions: ['pdf'],
}),
withHandlers({
onFileDrop: () => file => {
// do something with the dropped file
}
}),
withNativeFileDrop,
)(FileSection)
export default Wrapped
Function that parses the server error. Calls the passed function with the parsed error.
Has the following signature:
(callbackFn: (parsedError) => any) => (e: Error) => any
const customErrorLogger = parsedError =>
console.error(`This is very handled: ${parsedError}`)
// point free notation
anAsyncOperation().catch(handleError(customErrorLogger))
// can be used besides other function calls
anAsyncOperation().catch(err => {
setFetching(false)
handleError(customErrorLogger)(err)
})
FAQs
Unknown package
The npm package component-hindawi-ui receives a total of 2 weekly downloads. As such, component-hindawi-ui popularity was classified as not popular.
We found that component-hindawi-ui demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.