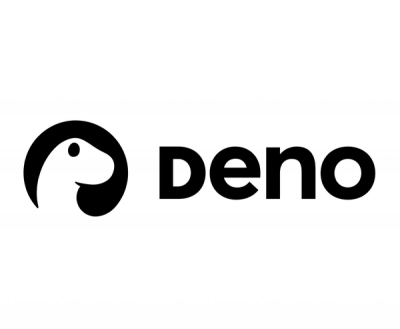
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Run and compose async tasks. Easily define groups of tasks to run in series or parallel.
Run and compose async tasks. Easily define groups of tasks to run in series or parallel.
Please consider following this project's author, Brian Woodward, and consider starring the project to show your :heart: and support.
(TOC generated by verb using markdown-toc)
Install with npm:
$ npm install --save composer
const Composer = require('composer');
const composer = new Composer();
composer.task('default', cb => {
console.log('Task: ', this.name);
cb();
});
composer.build('default')
.then(() => console.log('done!'))
.catch(console.error);
Factory for creating a custom Tasks
class that extends the given Emitter
. Or, simply call the factory function to use the built-in emitter.
Params
Emitter
{function}: Event emitter.returns
{Class}: Returns a custom Tasks
class.Example
// custom emitter
const Emitter = require('events');
const Tasks = require('composer/lib/tasks')(Emitter);
// built-in emitter
const Tasks = require('composer/lib/tasks')();
const composer = new Tasks();
Create an instance of Tasks
with the given options
.
Params
options
{object}Example
const Tasks = require('composer').Tasks;
const composer = new Tasks();
Define a task. Tasks run asynchronously, either in series (by default) or parallel (when options.parallel
is true). In order for the build to determine when a task is complete, one of the following things must happen: 1) the callback must be called, 2) a promise must be returned, or 3) a stream must be returned.
Params
name
{String}: The task name.deps
{Object|Array|String|Function}: Any of the following: task dependencies, callback(s), or options object, defined in any order.callback
{Function}: (optional) If the last argument is a function, it will be called after all of the task's dependencies have been run.returns
{undefined}Example
// 1. callback
app.task('default', cb => {
// do stuff
cb();
});
// 2. promise
app.task('default', () => {
return Promise.resolve(null);
});
// 3. stream (using vinyl-fs or your stream of choice)
app.task('default', function() {
return vfs.src('foo/*.js');
});
Run one or more tasks.
Params
tasks
{object|array|string|function}: One or more tasks to run, options, or callback function. If no tasks are defined, the default task is automatically run.callback
{function}: (optional)returns
{undefined}Example
const build = app.series(['foo', 'bar', 'baz']);
// promise
build().then(console.log).catch(console.error);
// or callback
build(function() {
if (err) return console.error(err);
});
Compose a function to run the given tasks in series.
Params
tasks
{object|array|string|function}: Tasks to run, options, or callback function. If no tasks are defined, the default
task is automatically run, if one exists.callback
{function}: (optional)returns
{promise|undefined}: Returns a promise if no callback is passed.Example
const build = app.series(['foo', 'bar', 'baz']);
// promise
build().then(console.log).catch(console.error);
// or callback
build(function() {
if (err) return console.error(err);
});
Compose a function to run the given tasks in parallel.
Params
tasks
{object|array|string|function}: Tasks to run, options, or callback function. If no tasks are defined, the default
task is automatically run, if one exists.callback
{function}: (optional)returns
{promise|undefined}: Returns a promise if no callback is passed.Example
// call the returned function to start the build
const build = app.parallel(['foo', 'bar', 'baz']);
// promise
build().then(console.log).catch(console.error);
// callback
build(function() {
if (err) return console.error(err);
});
// example task usage
app.task('default', build);
Static factory method for creating a custom Composer
class that extends the given Emitter
.
Params
Emitter
{function}: Event emitter.returns
{Class}: Returns a custom Composer
class.Example
const Emitter = require('events');
const Composer = require('composer').create(Emitter);
const composer = new Composer();
Create a wrapped generator function with the given name
, config
, and fn
.
Params
name
{string}config
{object}: (optional)fn
{function}returns
{function}Returns true if the given value is a Composer generator object.
Params
val
{object}returns
{boolean}Alias to .setGenerator
.
Params
name
{string}: The generator's nameoptions
{object|Function|String}: or generatorgenerator
{object|Function|String}: Generator function, instance or filepath.returns
{object}: Returns the generator instance.Example
app.register('foo', function(app, base) {
// "app" is a private instance created for the generator
// "base" is a shared instance
});
Get and invoke generator name
, or register generator name
with the given val
and options
, then invoke and return the generator instance. This method differs from .register
, which lazily invokes generator functions when .generate
is called.
Params
name
{string}fn
{function|Object}: Generator function, instance or filepath.returns
{object}: Returns the generator instance or undefined if not resolved.Example
app.generator('foo', function(app, options) {
// "app" - private instance created for generator "foo"
// "options" - options passed to the generator
});
Store a generator by file path or instance with the given name
and options
.
Params
name
{string}: The generator's nameoptions
{object|Function|String}: or generatorgenerator
{object|Function|String}: Generator function, instance or filepath.returns
{object}: Returns the generator instance.Example
app.setGenerator('foo', function(app, options) {
// "app" - new instance of Generator created for generator "foo"
// "options" - options passed to the generator
});
Get generator name
from app.generators
, same as [findGenerator], but also invokes the returned generator with the current instance. Dot-notation may be used for getting sub-generators.
Params
name
{string}: Generator name.returns
{object|undefined}: Returns the generator instance or undefined.Example
const foo = app.getGenerator('foo');
// get a sub-generator
const baz = app.getGenerator('foo.bar.baz');
Find generator name
, by first searching the cache, then searching the cache of the base
generator. Use this to get a generator without invoking it.
Params
name
{string}options
{function}: Optionally supply a rename function on options.toAlias
returns
{object|undefined}: Returns the generator instance if found, or undefined.Example
// search by "alias"
const foo = app.findGenerator('foo');
// search by "full name"
const foo = app.findGenerator('generate-foo');
Params
name
{string}returns
{boolean}Example
console.log(app.hasGenerator('foo'));
console.log(app.hasGenerator('foo.bar'));
Run one or more tasks or sub-generators and returns a promise.
Params
name
{string}tasks
{string|Array}returns
{promise}Events
emits
: generate
with the generator name
and the array of tasks
that are queued to run.Example
// run tasks `bar` and `baz` on generator `foo`
app.generate('foo', ['bar', 'baz']);
// or use shorthand
app.generate('foo:bar,baz');
// run the `default` task on generator `foo`
app.generate('foo');
// run the `default` task on the `default` generator, if defined
app.generate();
Create a generator alias from the given name
. By default, generate-
is stripped from beginning of the generator name.
Params
name
{string}options
{object}returns
{string}: Returns the alias.Example
// customize the alias
const app = new Generate({ toAlias: require('camel-case') });
Returns true if every name in the given array is a registered generator.
Params
names
{array}returns
{boolean}Format task and generator errors.
Params
name
{string}returns
{error}Get the first ancestor instance when generator.parent
is defined on nested instances.
Get or set the generator name.
Params
{string}
returns
{string}
Get or set the generator alias
. By default, the generator alias is created
by passing the generator name to the .toAlias method.
Params
{string}
returns
{string}
Get the generator namespace. The namespace is created by joining the generator's alias
to the alias of each ancestor generator.
Params
{string}
returns
{string}
Get the depth of a generator - useful for debugging. The root generator
has a depth of 0
, sub-generators add 1
for each level of nesting.
returns
{number}Static method that returns true if the given val
is an instance of Generate.
Params
val
{object}returns
{boolean}app.on('task', function(task) {
switch (task.status) {
case 'pending':
// Task was registered
break;
case 'preparing':
// Task is preparing to run, emitted right before "starting"
// (hint: you can use this event to dynamically skip tasks
// by updating "task.skip" to "true" or a function)
break;
case 'starting':
// Task is running
break;
case 'finished':
// Task is finished running
break;
}
});
See the changelog.
Pull requests and stars are always welcome. For bugs and feature requests, please create an issue.
Running and reviewing unit tests is a great way to get familiarized with a library and its API. You can install dependencies and run tests with the following command:
$ npm install && npm test
(This project's readme.md is generated by verb, please don't edit the readme directly. Any changes to the readme must be made in the .verb.md readme template.)
To generate the readme, run the following command:
$ npm install -g verbose/verb#dev verb-generate-readme && verb
You might also be interested in these projects:
Commits | Contributor |
---|---|
226 | doowb |
59 | jonschlinkert |
Brian Woodward
Copyright © 2018, Brian Woodward. Released under the MIT License.
This file was generated by verb-generate-readme, v0.6.0, on April 28, 2018.
FAQs
Run and compose async tasks. Easily define groups of tasks to run in series or parallel.
The npm package composer receives a total of 26,117 weekly downloads. As such, composer popularity was classified as popular.
We found that composer demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.