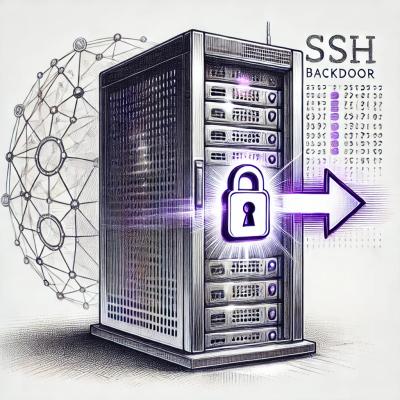
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
compute-anagram-hash
Advanced tools
Anagram hash table.
$ npm install compute-anagram-hash
For use in the browser, use browserify.
var createHash = require( 'compute-anagram-hash' );
Creates an anagram hash table.
var hash = createHash();
To initialize the hash table, provide a string array
.
var arr = [
'beep',
'boop',
'bop',
'bap',
'foo',
'bar',
'cat',
'bat',
'moot',
'woot',
'moto',
'tab',
'pad',
'Shakespeare is awesome!'
];
var hash = createHash( arr );
The hash table has the following methods...
Add strings
to the anagram hash table.
hash.push( 'dog', 'rad', 'super' );
Returns a list of anagrams. If provided an input string
, the method returns a list of corresponding anagrams from the hash table; otherwise, the method returns all anagram lists. If no anagrams exist, the method returns null
.
// Get all anagrams:
var lists = hash.get();
// returns [['bat','tab'],['moot','moto']]
// Get anagrams corresponding to a particular string:
var list = hash.get( 'moot' );
// returns ['moto']
list = hash.get( 'beep' );
// returns null
If the input string
is a hash key, set the key
flag to true
. When provided a hash key, the method returns all anagrams associated with a particular key (possibly including the key itself).
var list = hash.get( 'abt', true );
// returns ['bat','tab']
list = hash.get( 'beep', true );
// returns ['beep']
Note: when returning all anagram lists, the list order is not guaranteed.
Hashing function. Converts an input string
to an alphagram.
var key = hash.getKey( 'tab' );
// returns 'abt'
var list = hash.get( key, true );
// returns ['bat','tab']
Returns a list of hash keys.
var keys = hash.keys();
// returns ['abp','abr','abt','act',...]
The method accepts the following options
:
To return keys
having at least a min
number of anagrams, set the min
option.
var keys = hash.keys({ 'min': 2 });
// returns ['abt','moot']
To return keys
having at most a max
number of anagrams, set the max
option.
var keys = hash.keys({ 'max': 1 });
// returns ['abp','abr','act','adp',...]
Merges anagram hash tables into the current anagram hash instance.
var mhash1, mhash2;
mhash1 = createHash( ['yes','no'] );
mhash2 = createHash( ['beep','bepe'] );
hash.merge( mhash1, mhash2 );
var list = hash.get( 'beep', true );
// returns ['beep','bepe']
Copies an anagram hash table to a new hash table instance.
var copy = hash.copy();
var list = copy.get( 'abt', true );
// returns ['bat','tab']
To only copy specific keys to a new hash table, provide a keys array
.
var copy = hash.copy( ['beep'] );
var keys = copy.keys();
// returns ['beep']
var createHash = require( 'compute-anagram-hash' );
// Load a string array containing tokenized words:
var words = require( './words.json' );
// Create a new hash:
var hash = createHash( words );
// Get all anagram lists:
var lists = hash.get();
// Get a single anagram list:
var list = hash.get( 'rome' );
To run the example code from the top-level application directory,
$ node ./examples/index.js
Unit tests use the Mocha test framework with Chai assertions. To run the tests, execute the following command in the top-level application directory:
$ make test
All new feature development should have corresponding unit tests to validate correct functionality.
This repository uses Istanbul as its code coverage tool. To generate a test coverage report, execute the following command in the top-level application directory:
$ make test-cov
Istanbul creates a ./reports/coverage
directory. To access an HTML version of the report,
$ make view-cov
Copyright © 2015. Athan Reines.
FAQs
Anagram hash table.
The npm package compute-anagram-hash receives a total of 0 weekly downloads. As such, compute-anagram-hash popularity was classified as not popular.
We found that compute-anagram-hash demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.