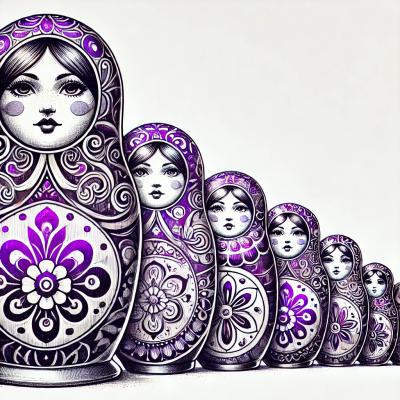
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
The config npm package is designed to simplify the management of configuration settings for Node.js applications. It allows developers to organize configuration variables for different deployment environments, such as development, testing, and production, in a structured and accessible manner. This package supports configuration file formats like JSON, YAML, and JavaScript, enabling easy integration into various projects.
Environment-Specific Configurations
This feature allows you to load different configurations based on the current environment (e.g., development, production). The code sample demonstrates how to access a database configuration specific to the current environment.
const config = require('config');
let dbConfig = config.get('Customer.dbConfig');
console.log(dbConfig.host);
Custom Environment Variables
Leverage custom environment variables within your configuration files. The example shows how to access a nested configuration property, such as a database password.
const config = require('config');
let dbPassword = config.get('Customer.dbConfig.password');
console.log(dbPassword);
Configuration File Formats
Supports multiple configuration file formats including JSON, YAML, and JavaScript. This example demonstrates accessing a server port setting from a JSON configuration file.
// Assuming you have a JSON config file named 'default.json' in your config directory
const config = require('config');
let serverPort = config.get('server.port');
console.log(serverPort);
Dotenv is a module that loads environment variables from a .env file into process.env. While dotenv is focused on loading environment variables, config deals with organizing and accessing hierarchical configurations.
nconf is a hierarchical node.js configuration with files, environment variables, command-line arguments, and atomic object merging. It provides a similar functionality to config but with a different approach to organizing and prioritizing configuration sources.
Configuration control for production node deployments
Node-config is a configuration system for Node.js application server deployments. It lets you define a default set of application parameters, and tune them for different runtime environments (development, qa, staging, production, etc.).
Parameters defined by node-config can be monitored and tuned at runtime without bouncing your production servers.
Online documentation is available at http://lorenwest.github.com/node-config/latest
In your project directory, install and verify using npm:
my-project$ npm install config
my-project$ npm test config
Edit the default configuration file (.js, .json, or .yaml):
my-project$ mkdir config
my-project$ vi config/default.yaml
(example default.yaml file):
Customer:
dbHost: localhost
dbPort: 5984
dbName: customers
Edit the production configuration file:
my-project$ vi config/production.yaml
(example production.yaml file):
Customer:
dbHost: prod-db-server
Use the configuration in your code:
var CONFIG = require('config').Customer;
...
db.connect(CONFIG.dbHost, CONFIG.dbPort, CONFIG.dbName);
Start your application server:
my-project$ export NODE_ENV=production
my-project$ node app.js
Running in this configuration, CONFIG.dbPort and CONFIG.dbName
will come from the default.yaml
file, and CONFIG.dbHost will
come from the production.yaml
file.
node-config - Online documentation
node-monitor - Monitor your running node applications
Released under the Apache License 2.0
See LICENSE
file.
Copyright (c) 2011 Loren West
FAQs
Configuration control for production node deployments
The npm package config receives a total of 1,156,477 weekly downloads. As such, config popularity was classified as popular.
We found that config demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.