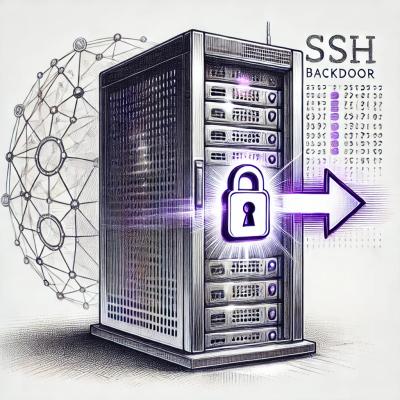
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
connective-tissue
Advanced tools
[](https://travis-ci.com/MatthewZito/connective-tissue) [](https://badge.fury.io/js/connective-tissue) [](https://coveralls.io/github/MatthewZito/connective-tissue?branch=master) [![Continuous Deployment](https://github.com/MatthewZito/connective-tiss
The npm package connective-tissue receives a total of 0 weekly downloads. As such, connective-tissue popularity was classified as not popular.
We found that connective-tissue demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.