console-gui-tools
A simple library to draw option menu or other popup inputs and layout on Node.js console.

users-session-manager
A simple Node.js library to create Console Apps like wizard (or maybe if you like old style colored screen or something like "teletext" programs 😂)
Apart from jokes, it is a library that allows you to create a screen divided into a part with everything you want to see (such as variable values) and another in which the logs run.
Moreover in this way the application is managed by the input event "keypressed" to which each key corresponds to a bindable command.
For example, to change variables you can open popups with an option selector or with a textbox.
It's in embryonic phase, any suggestion will be constructive :D

Installation
Install with:
npm i console-gui-tools
Example of usage:
import { ConsoleManager, OptionPopup, InputPopup } from '../index.js'
const GUI = new ConsoleManager()
const updateConsole = async() => {
let screen = ""
screen += chalk.yellow(`TCP server simulator app! Welcome...`) + `\n`
screen += chalk.green(`TCP Server listening on ${HOST}:${PORT}`) + `\n`
screen += chalk.green(`Connected clients: `) + chalk.white(`${connectedClients}\n`)
screen += chalk.magenta(`TCP Messages sent: `) + chalk.white(`${tcpCounter}`) + `\n\n`
if (!valueEmitter) {
screen += chalk.red(`Simulator is not running! `) + chalk.white(`press 'space' to start`) + `\n`
} else {
screen += chalk.green(`Simulator is running! `) + chalk.white(`press 'space' to stop`) + `\n`
}
screen += chalk.cyan(`Mode:`) + chalk.white(` ${mode}`) + `\n`;
screen += chalk.cyan(`Message period:`) + chalk.white(` ${period} ms`) + `\n`;
screen += chalk.cyan(`Min:`) + chalk.white(` ${min}`) + `\n`;
screen += chalk.cyan(`Max:`) + chalk.white(` ${max}`) + `\n`;
screen += chalk.cyan(`Values:`) + chalk.white(` ${values.map(v => v.toFixed(4)).join(' ')}`) + `\n`;
screen += `\n\n`;
if (lastErr.length > 0) {
screen += lastErr + `\n\n`
}
screen += chalk.bgBlack(`Commands:`) + `\n`;
screen += ` ${chalk.bold('space')} - ${chalk.italic('Start/stop simulator')}\n`;
screen += ` ${chalk.bold('m')} - ${chalk.italic('Select simulation mode')}\n`;
screen += ` ${chalk.bold('s')} - ${chalk.italic('Select message period')}\n`;
screen += ` ${chalk.bold('h')} - ${chalk.italic('Set max value')}\n`;
screen += ` ${chalk.bold('l')} - ${chalk.italic('Set min value')}\n`;
screen += ` ${chalk.bold('q')} - ${chalk.italic('Quit')}\n`;
GUI.setHomePage(screen)
}
GUI.on("keypressed", (key) => {
if (key.ctrl && key.name === 'c') {
closeApp()
}
switch (key.name) {
case 'space':
if (valueEmitter) {
clearInterval(valueEmitter)
valueEmitter = null
} else {
valueEmitter = setInterval(frame, period)
}
break
case 'm':
new OptionPopup("popupSelectMode", "Select simulation mode", modeList, mode).show().once("confirm", (_mode) => {
mode = _mode
GUI.warn(`NEW MODE: ${mode}`)
drawGui()
})
break
case 's':
new OptionPopup("popupSelectPeriod", "Select simulation period", periodList, period).show().once("confirm", (_period) => {
period = _period
GUI.warn(`NEW PERIOD: ${period}`)
drawGui()
})
break
case 'h':
new InputPopup("popupTypeMax", "Type max value", max, true).show().once("confirm", (_max) => {
max = _max
GUI.warn(`NEW MAX VALUE: ${max}`)
drawGui()
})
break
case 'l':
new InputPopup("popupTypeMin", "Type min value", min, true).show().once("confirm", (_min) => {
min = _min
GUI.warn(`NEW MIN VALUE: ${min}`)
drawGui()
})
break
case 'q':
closeApp()
break
default:
break
}
drawGui()
})
const drawGui = () => {
updateConsole()
}
new OptionPopup("popupSelectPeriod", "Select simulation period", periodList, period).show().once("confirm", (_period) => {
period = _period
GUI.warn(`NEW PERIOD: ${period}`)
drawGui()
})
The response is triggered via EventEmitter using "once" (not "on")
The result is this:
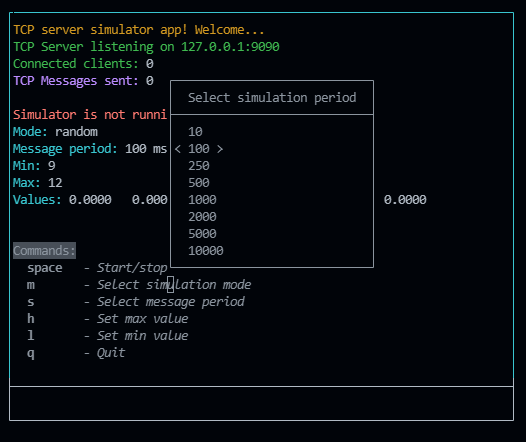
Pressing enter it will close the popup and set the new value:
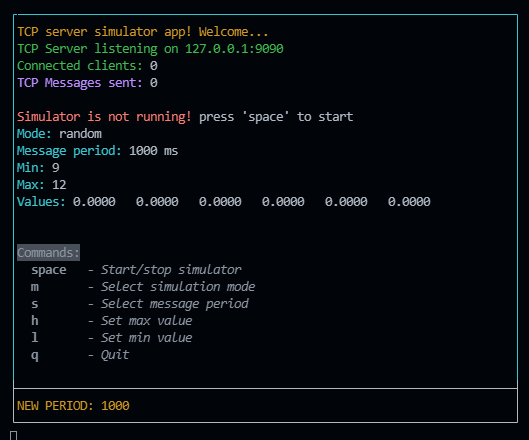
You can also use it to set a numeric threshold:
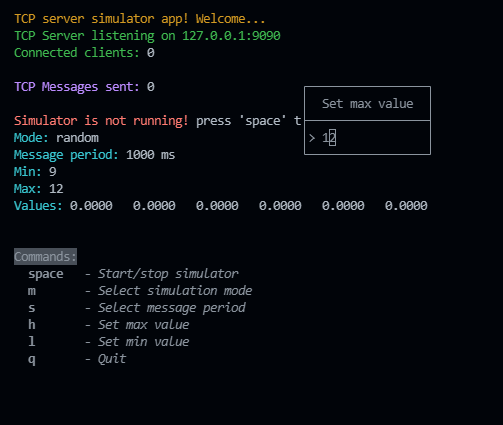
Only numbers are allowed.
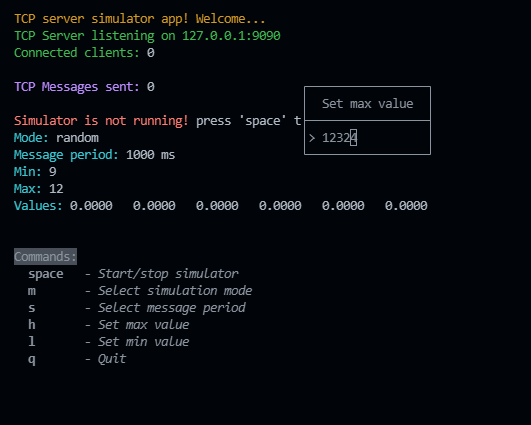
Console.log and other logging tools
To log you have to use the following functions:
GUI.log(`NEW MIN VALUE: ${min}`)
GUI.warn(`NEW MIN VALUE: ${min}`)
GUI.error(`NEW MIN VALUE: ${min}`)
GUI.info(`NEW MIN VALUE: ${min}`)
And they written to the bottom of the page.
This library is in development now. New componets will come asap.