console-gui-tools
A simple library to draw option menu or other popup inputs and layout on Node.js console.

console-gui-tools
A simple Node.js library to create Console Apps like wizard (or maybe if you like old style colored screen or something like "teletext" programs 😂)
Apart from jokes, it is a library that allows you to create a screen divided into a part with everything you want to see (such as variable values) and another in which the logs run.
Moreover in this way the application is managed by the input event "keypressed" to which each key corresponds to a bindable command.
For example, to change variables you can open popups with an option selector or with a textbox.
It's in embryonic phase, any suggestion will be constructive :D
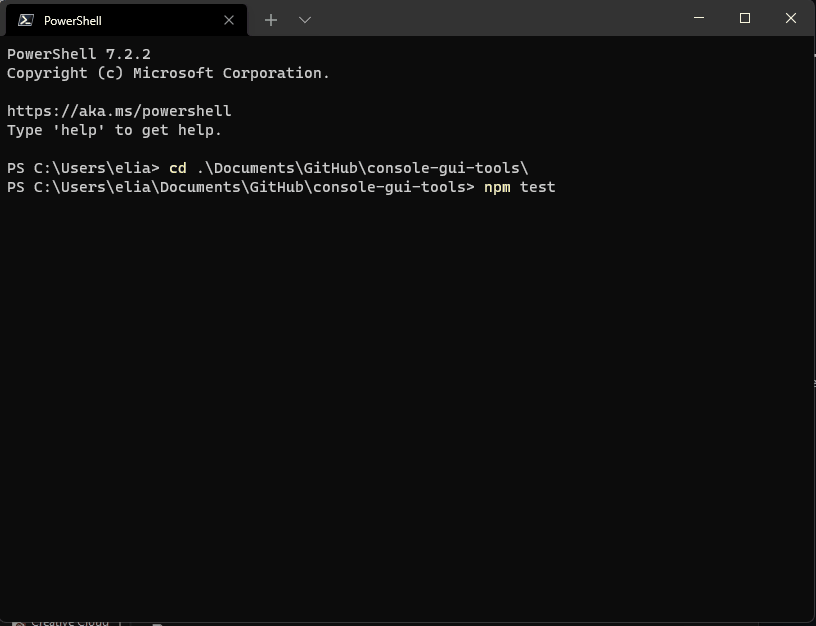

Installation
Install with:
npm i console-gui-tools
Options
The library has a few options that can be set in the constructor.
options.title
The title of the application. It will be displayed in the top of the screen.
options.logsPageSize
The number of lines that will be displayed in the logs page.
options.changeLayoutKey
The key that will be used to change the layout. (To switch between the two pages, logs and main page)
options.layoutBorder
To enable the border of the layout and the title.
Example of usage:
import { ConsoleManager, OptionPopup, InputPopup } from '../index.js'
const GUI = new ConsoleManager({
title: 'TCP Simulator',
logsPageSize: 12,
changeLayoutKey: 'ctrl+l',
})
const updateConsole = async() => {
let screen = ""
screen += chalk.yellow(`TCP server simulator app! Welcome...`) + `\n`
screen += chalk.green(`TCP Server listening on ${HOST}:${PORT}`) + `\n`
screen += chalk.green(`Connected clients: `) + chalk.white(`${connectedClients}\n`)
screen += chalk.magenta(`TCP Messages sent: `) + chalk.white(`${tcpCounter}`) + `\n\n`
if (!valueEmitter) {
screen += chalk.red(`Simulator is not running! `) + chalk.white(`press 'space' to start`) + `\n`
} else {
screen += chalk.green(`Simulator is running! `) + chalk.white(`press 'space' to stop`) + `\n`
}
screen += chalk.cyan(`Mode:`) + chalk.white(` ${mode}`) + `\n`;
screen += chalk.cyan(`Message period:`) + chalk.white(` ${period} ms`) + `\n`;
screen += chalk.cyan(`Min:`) + chalk.white(` ${min}`) + `\n`;
screen += chalk.cyan(`Max:`) + chalk.white(` ${max}`) + `\n`;
screen += chalk.cyan(`Values:`) + chalk.white(` ${values.map(v => v.toFixed(4)).join(' ')}`) + `\n`;
screen += `\n\n`;
if (lastErr.length > 0) {
screen += lastErr + `\n\n`
}
screen += chalk.bgBlack(`Commands:`) + `\n`;
screen += ` ${chalk.bold('space')} - ${chalk.italic('Start/stop simulator')}\n`;
screen += ` ${chalk.bold('m')} - ${chalk.italic('Select simulation mode')}\n`;
screen += ` ${chalk.bold('s')} - ${chalk.italic('Select message period')}\n`;
screen += ` ${chalk.bold('h')} - ${chalk.italic('Set max value')}\n`;
screen += ` ${chalk.bold('l')} - ${chalk.italic('Set min value')}\n`;
screen += ` ${chalk.bold('q')} - ${chalk.italic('Quit')}\n`;
GUI.setHomePage(screen)
}
GUI.on("keypressed", (key) => {
switch (key.name) {
case 'space':
if (valueEmitter) {
clearInterval(valueEmitter)
valueEmitter = null
} else {
valueEmitter = setInterval(frame, period)
}
break
case 'm':
new OptionPopup("popupSelectMode", "Select simulation mode", modeList, mode).show().on("confirm", (_mode) => {
mode = _mode
GUI.warn(`NEW MODE: ${mode}`)
drawGui()
})
break
case 's':
new OptionPopup("popupSelectPeriod", "Select simulation period", periodList, period).show().on("confirm", (_period) => {
period = _period
GUI.warn(`NEW PERIOD: ${period}`)
drawGui()
})
break
case 'h':
new InputPopup("popupTypeMax", "Type max value", max, true).show().on("confirm", (_max) => {
max = _max
GUI.warn(`NEW MAX VALUE: ${max}`)
drawGui()
})
break
case 'l':
new InputPopup("popupTypeMin", "Type min value", min, true).show().on("confirm", (_min) => {
min = _min
GUI.warn(`NEW MIN VALUE: ${min}`)
drawGui()
})
break
case 'q':
closeApp()
break
default:
break
}
})
const drawGui = () => {
updateConsole()
}
new OptionPopup("popupSelectPeriod", "Select simulation period", periodList, period).show().on("confirm", (_period) => {
period = _period
GUI.warn(`NEW PERIOD: ${period}`)
drawGui()
})
constructor(id, title, options, selected)
- id: string
- title: string
- options: Array<string | number>
- selected: string | number
The response is triggered via EventEmitter using "on"
The result is this:
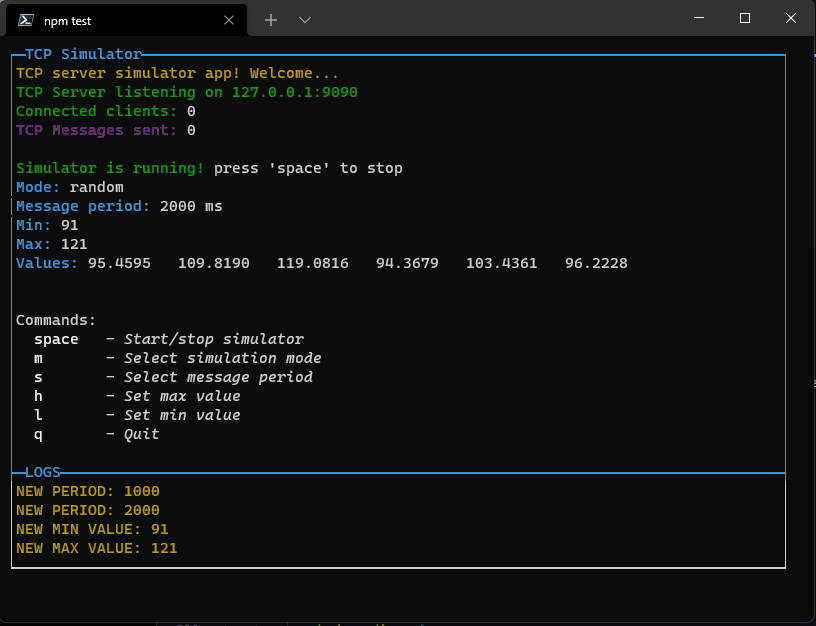
Pressing enter it will close the popup and set the new value
new InputPopup("popupTypeMax", "Type max value", max, true).show().on("confirm", (_max) => {
max = _max
GUI.warn(`NEW MAX VALUE: ${max}`)
drawGui()
})
constructor(id, title, value, isNumeric)
- id: string
- title: string
- value: string | number
- isNumeric: boolean
You can use it for example to set a numeric threshold:
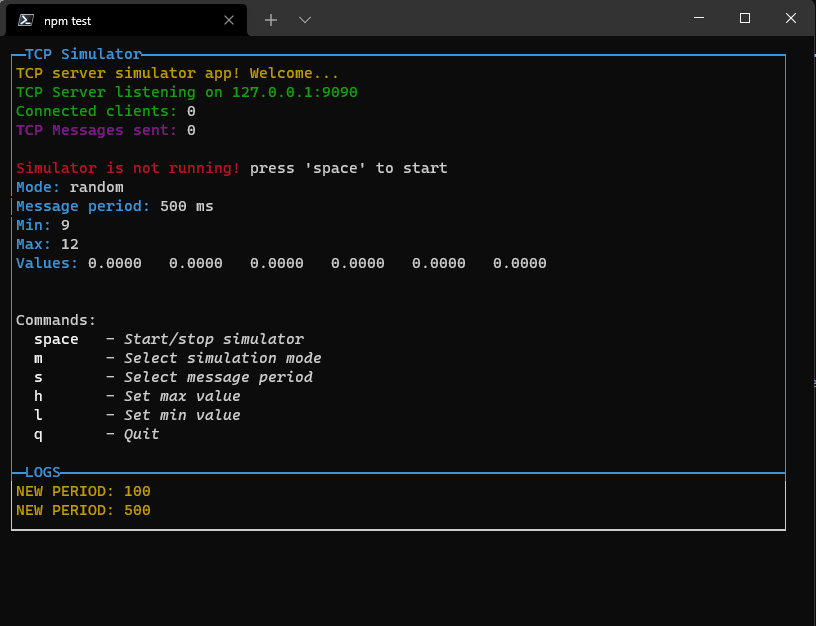
If you set isNumeric to true, only numbers are allowed.
All class of components will be destroyed when the popup is closed. The event listeners are removed from the store. Then the garbage collector will clean the memory.
Console.log and other logging tools
To log you have to use the following functions:
GUI.log(`NEW MIN VALUE: ${min}`)
GUI.warn(`NEW MIN VALUE: ${min}`)
GUI.error(`NEW MIN VALUE: ${min}`)
GUI.info(`NEW MIN VALUE: ${min}`)
And they written to the bottom of the page.
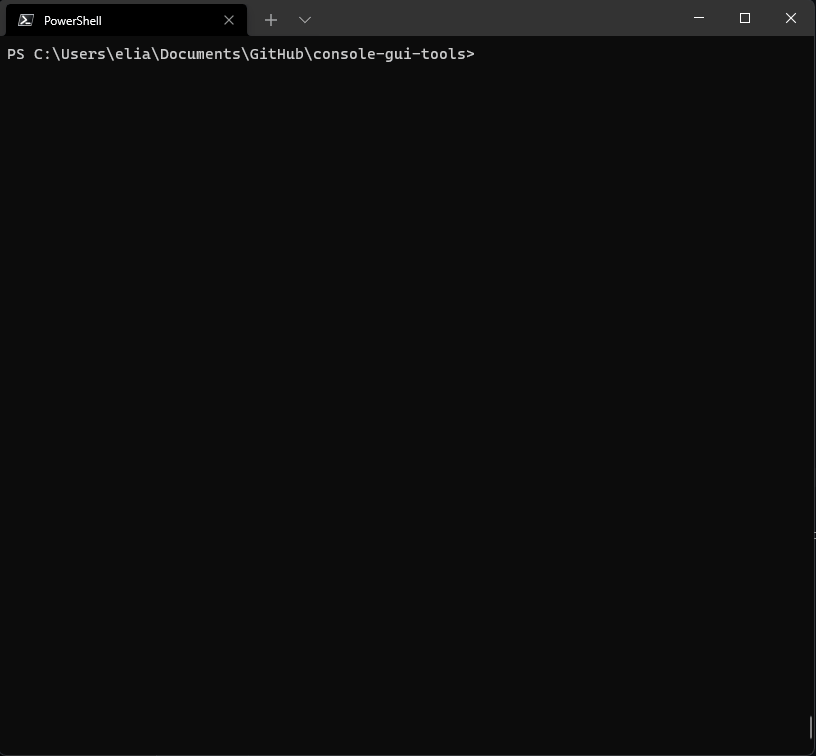
You can switch to the log view by pressing the "changeLayoutKey" key or combination:
The maximum number of lines is set to 10 by default but you can change it by setting the option "logsPageSize".
When the logs exceed the limit, you can scroll up and down with up and down arrows (if you are in the log view).
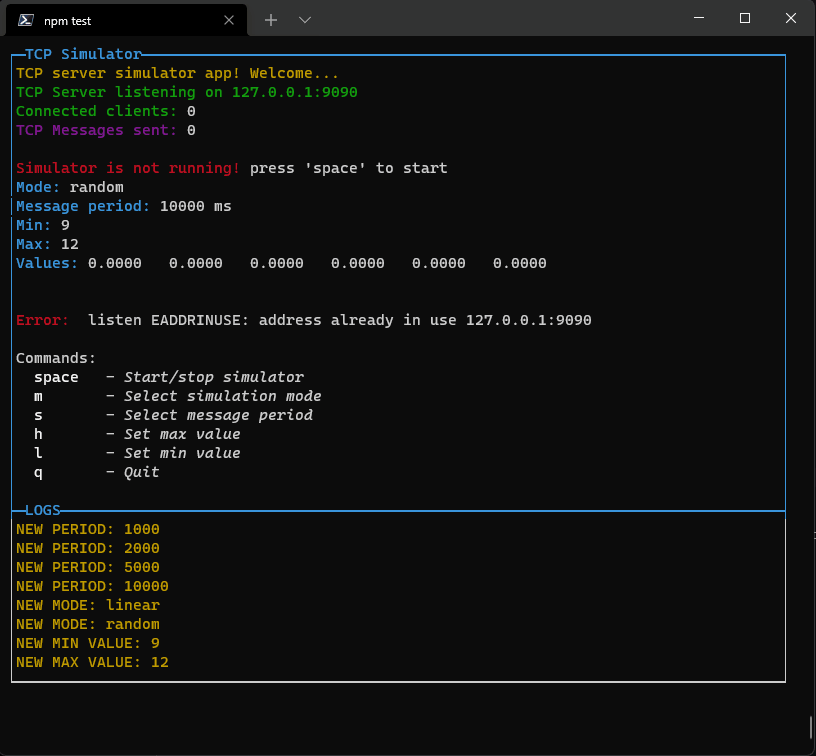
This library is in development now. New componets will come asap.