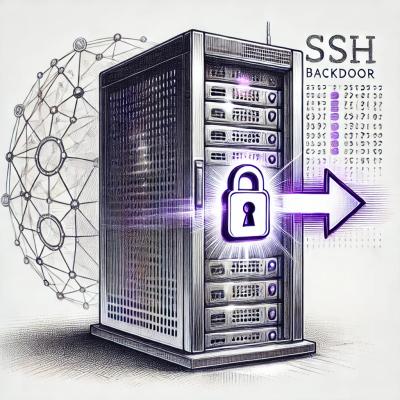
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
contact-form-hexipi
Advanced tools
//As a Class Component
import React from 'react';
import ContactForm, { FormRes } from './components/ContactForm';
import './App.css';
class App extends React.Component {
state = {
formSubmitResult: FormRes.NONE,
};
onSubmit = formData => {
//Use to call your backend/API functions (or anything else you need)
//to send your form data.
alert(`Form Data: ${JSON.stringify(formData)}`);
this.setState({
formSubmitResult: FormRes.OK,
})
};
//Set the state of the 'formSubmitResult' to the default so that
//the form could be displayed again
formSubmitResultReset = () => this.setState({ formSubmitResult: FormRes.NONE });
render() {
return (
<div className="App">
<header className="App-header">
<ContactForm
submitMethod="post"
email="info@example.com"
tel="+18005555555"
socialMediaLinks={[
//Facebook link
"https://www.facebook.com/HexiPi.Web.Dev",
//Instagram Link
"https://instagram.com/hexipi",
//YouTube link
"https://www.youtube.com/channel/UCxJUbbqJ_3hpaL53vn2EFVA",
//Website Link
"https://hexipi.com/"
//...Or anything you can think of!
]}
onSubmitCallback={this.onSubmit}
formSubmitResult={this.state.formSubmitResult}
formSubmitResultReset={this.formSubmitResultReset}
/>
</header>
</div>
);
}
}
export default App;
//As a Funcational Component Using Hooks
import React, { useState } from 'react';
import ContactForm, { FormRes } from './components/ContactForm';
import './App.css';
const App = () => {
const [formSubmitResult, setFormSubmitResult] = useState(FormRes.NONE);
const onSubmit = formData => {
//Use to call your backend/API functions (or anything else you need)
//to send your form data.
alert(`Form Data: ${JSON.stringify(formData)}`);
setFormSubmitResult(FormRes.OK);
};
//Sets the state of the 'formSubmitResult' to the default so that
//the form could be displayed again
const formSubmitResultReset = () => setFormSubmitResult(FormRes.NONE);
return (
<div className="App">
<header className="App-header">
<ContactForm
submitMethod="post"
email="info@example.com"
tel="+18005555555"
socialMediaLinks={[
//Facebook link
"https://www.facebook.com/HexiPi.Web.Dev",
//Instagram Link
"https://instagram.com/hexipi",
//YouTube link
"https://www.youtube.com/channel/UCxJUbbqJ_3hpaL53vn2EFVA",
//Website Link
"https://hexipi.com/"
//...Or anything you can think of!
]}
onSubmitCallback={this.onSubmit}
formSubmitResult={this.state.formSubmitResult}
formSubmitResultReset={this.formSubmitResultReset}
/>
</header>
</div>
);
}
export default App;
interface ContactFormProps {
//The form submission method (either 'get' or 'post')
submitMethod: 'get' | 'post',
//The email address that would be displayed
email: string,
//The phone number that would be displayed
tel: string,
//The optional fax number that would be displayed
fax?: string,
//The array of social media links/web links that would be displayed
socialMediaLinks: string[],
//The optional custom main heading that would be displayed
mainHeading?: string,
//The optional custom subheading that would be displayed
subHeading?: string,
//The result of the form submission (one of the options of the FormRes enum)
formSubmitResult: FormRes,
//The callback that is executed after the form is submitted
//The 'formData' parameter holds the data that was submitted on the form
//of the type 'ContactFormSubmissionData'
onSubmitCallback: (formData: ContactFormSubmissionData) => void,
//The callback that is executed after the form is reset
formSubmitResultReset: () => void
};
static defaultProps = {
submitMethod: "get",
email: "info@example.com",
tel: "+15555555555",
fax: undefined,
socialMediaLinks: [
"https://www.facebook.com/HexiPi.Web.Dev",
"https://instagram.com/hexipi",
"https://www.youtube.com/channel/UCxJUbbqJ_3hpaL53vn2EFVA",
"https://hexipi.com/"
],
onSubmitCallback:
(formData: ContactFormSubmissionData) => alert(JSON.stringify(formData)),
mainHeading: "Need More Information?",
subHeading: "Send Us a Message!",
formSubmitResult: FormRes.NONE,
};
enum FormRes {
//The form submitted successfully; a success message is displayed
OK,
//An error occurred during form submission; an error message is displayed
ERROR,
//The form is displayed and is ready to be filled out
NONE
}
interface ContactFormSubmissionData {
name: string,
email: string,
phone_number?: string, //Optional and may have a value of 'undefined'
message: string
}
FAQs
This is a React.JS contact form module.
We found that contact-form-hexipi demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.