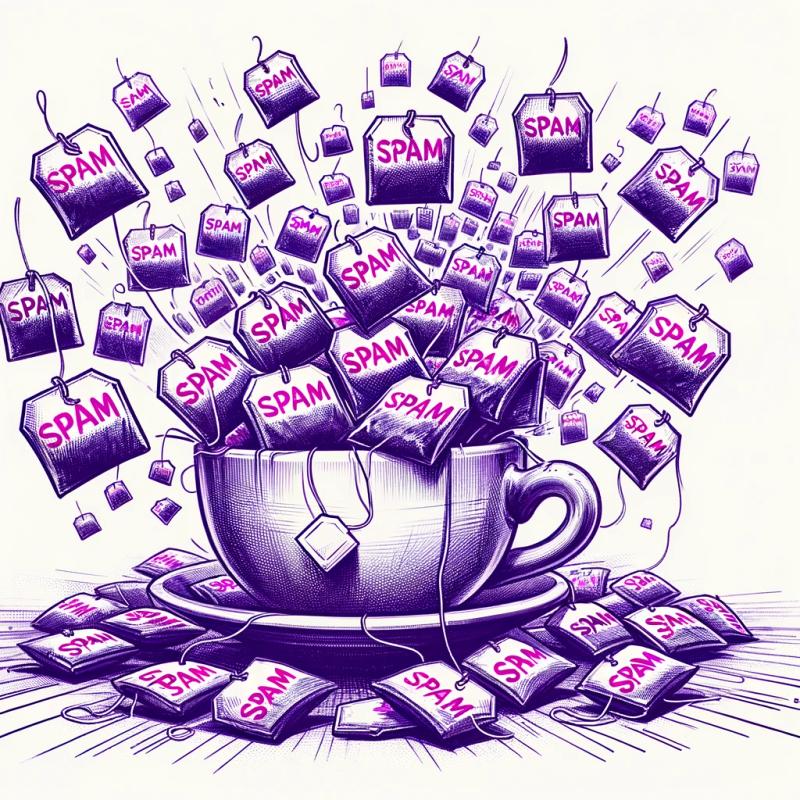
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
content-disposition
Advanced tools
Package description
The content-disposition npm package is used to create and parse HTTP Content-Disposition headers. It is commonly used to set the disposition type (inline or attachment) and parameters for a response in a web application, which instructs the browser how to handle the content, such as to display it inline or to download it as a file.
Creating Content-Disposition header for attachment
This code sample demonstrates how to create a Content-Disposition header for an attachment, suggesting the browser to download the file as 'filename.txt'.
const contentDisposition = require('content-disposition');
let header = contentDisposition('filename.txt');
Creating Content-Disposition header with Unicode filenames
This code sample shows how to create a Content-Disposition header for a file with a Unicode filename, ensuring proper encoding for non-ASCII characters.
const contentDisposition = require('content-disposition');
let header = contentDisposition('filename.txt', { type: 'attachment', filename: 'файл.txt' });
Parsing Content-Disposition header
This code sample illustrates how to parse a Content-Disposition header string to get an object representing the disposition type and parameters.
const contentDisposition = require('content-disposition');
let parsedHeader = contentDisposition.parse('attachment; filename="filename.txt"');
The 'mime' package can be used to determine a file's MIME type based on its extension, which is helpful when setting the Content-Type header. It does not directly handle Content-Disposition headers but is often used in conjunction with setting these headers.
The 'form-data' package allows for the creation and submission of FormData instances, which can include files with specific Content-Disposition. It is more focused on constructing multipart/form-data payloads than on creating or parsing Content-Disposition headers.
Readme
Create an attachment Content-Disposition header
$ npm install content-disposition
var contentDisposition = require('content-disposition')
Create an attachment Content-Disposition
header value using the given file name,
if supplied. The filename
is optional and if no file name is desired, but you
want to specify options
, set filename
to undefined
.
res.setHeader('Content-Disposition', contentDisposition('∫ maths.pdf'))
contentDisposition
accepts these properties in the options object.
If the filename
option is outside US-ASCII, then the file name is actually
stored in a supplemental field for clients that support Unicode file names and
a US-ASCII version of the file name is automatically generated.
This specifies the US-ASCII file name to override the automatic generation or
disables the generation all together, defaults to true
.
false
will disable including a US-ASCII file name and only include the
Unicode version (unless the file name is already US-ASCII).true
will enable automatic generation if the file name is outside US-ASCII.If the filename
option is US-ASCII and this option is specified and has a
different value, then the filename
option is encoded in the extended field
and this set as the fallback field, even though they are both US-ASCII.
Specifies the disposition type, defaults to "attachment"
. This can also be
"inline"
, or any other value (all values except inline are treated like
attachment
, but can convey additional information if both parties agree to
it). The type is normalized to lower-case.
var contentDisposition = require('content-disposition')
var destroy = require('destroy')
var http = require('http')
var onFinished = require('on-finished')
var filePath = '/path/to/public/plans.pdf'
http.createServer(function onRequest(req, res) {
// set headers
res.setHeader('Content-Type', 'application/pdf')
res.setHeader('Content-Disposition', contentDisposition(filePath))
// send file
var stream = fs.createReadStream(filePath)
stream.pipe(res)
onFinished(res, function (err) {
destroy(stream)
})
})
$ npm test
FAQs
Create and parse Content-Disposition header
The npm package content-disposition receives a total of 27,793,038 weekly downloads. As such, content-disposition popularity was classified as popular.
We found that content-disposition demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.