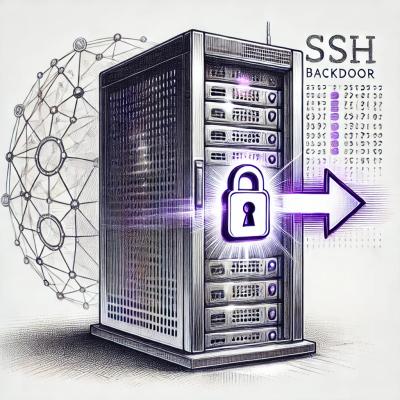
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
controller
Advanced tools
a small structural aid for creating express routes.
This code sets up an app with 3 handlers, 4 routes, and some middleware which applies to different handler groups.
var express = require('express');
var controller = require('controller');
var app = express();
var users = controller();
// Define handlers
users.define('secret-stuff', ['sensitive'], function(req, res) {});
users.define('edit-account', ['sensitive'], function(req, res) {});
users.define('view-account', function(req, res) {});
// Define middleware for all 'sensitive' grouped handlers
users.middleware('sensitive', function(req, res, next) {});
// Define middleware for all handlers on this controller
users.middleware('all', function(req, res, next) {});
// Define routes
users.route('get', '/secret-stuff/:id', 'secret-stuff');
users.route('put', '/user/edit/:id', 'edit-account');
users.route('get', '/user/:id', 'view-account');
users.route('get', '/view-user/:id', 'view-account');
// Attach to the app
users.attach(app);
Create a new controller by requiring controller and calling it as a function, like this:
var controller = require('controller');
var users = controller();
The Controller
function can also take an options
parameter. Available
options are:
prefix
a path to prefix all routes by. For example, you could set this to
'/user/'
, resulting in users.route('get', 'login', 'do-login');
routing to
/user/login
.Example with options:
var users = controller({ prefix: '/user/' });
users.direct('get', '/:id', function(req,res) {
res.send(Users.read(req.params.id));
})
Define a handler. A handler is a function that is called as the result of a route being visited. This does not route the handler, it only creates it, ready for routing.
Parameters
name
- the name of the handlergroups
(optional) - the groups to add this handler to, for the purpose of
applying middleware to groups of handlers.handler
- the function that is called when the route is visited.Example
users.define('view', function(req, res) {
res.send(Users.read(req.params.id));
});
users.define('edit', ['require-login'], function(req, res) {
Users.update(req.params.id, req.body);
res.send(200);
});
Define some middleware for a group. If middleware
is not defined, an array of
middleware for the group is returned instead. If group
is not defined,
the 'all'
group is returned - this is a group of middleware which applies to
all handlers.
The array that is returned can also be used to add more middleware.
The order that middleware is added is as follows:
Paramaters
group
optional - defaults to 'all'
middleware
optional - middleware to add to group
.Example
users.middleware('require-login', function checkLoggedIn(req, res, next) {
// -> check if the user is logged in
});
users.middleware('require-login'); // -> [ [Function checkLoggedIn] ]
users.middleware('require-login').push(function(req,res,next) {});
// Define some middleware for all routes
users.middleware(function(res, req, next) {});
Route a handler. Handlers can be routed at more than one location.
Parameters
method
. The http method, for example 'get'
, 'post'
, 'put'
, etc.path
. The path to route the handler to, in exactly the same format you would
pass to express. You can use a regex, but it will ignore options.prefix
.handlerName
. The name of the handler to route.Example
users.route('get', '/user/:id', 'view');
users.route('post', '/user/:id', 'create');
users.route('put', '/user/:id', 'edit');
Directly route a function optionally with some middleware. This is essentially
the same as adding a route directly to express. The difference is that handlers
defined with direct
can be included in the controller's middleware groups, and
will be included in the all
group.
Paramaters
method
. The http method, for example 'get'
, 'post'
, 'put'
, etc.path
. The path to route the handler to, in exactly the same format you would
pass to express. You can use a regex, but it will ignore options.prefix
.middleware/groups
. A bunch of middlewares or groups to add the route to.
These can be mixed and matched, Controller will figure it out.handlerfn
. The handler function to call when the route is visited.Example
var uselessMiddleware = function(req,res,next) { next(); };
users.direct('delete', '/user/:id', uselessMiddleware, 'require-login', function(req, res) {
Users.delete(req.params.id);
res.end();
});
users.direct('get', '/user/do-something', function(req, res) {});
Attach the routes to an express app. Note that after calling this function, making changes to the routes on the controller will do nothing.
FAQs
an action controller for express
The npm package controller receives a total of 296 weekly downloads. As such, controller popularity was classified as not popular.
We found that controller demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.