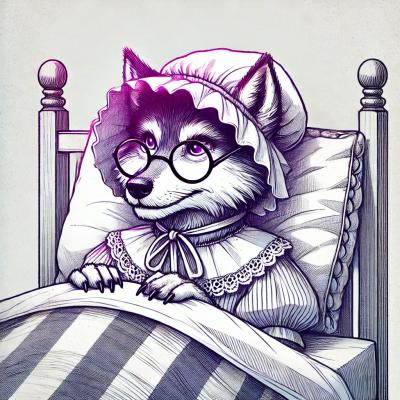
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
country-locale-map
Advanced tools
Provides mapping between country codes and provides default country locales. Includes optional fuzzy matching for country name.
Provides easy conversion between country codes and names as well as a default locale for each country. Includes fuzzy matching for country name lookups. Methods return undefined if no matches are found
const clm = require('country-locale-map');
clm.getAlpha3ByAlpha2('CA'); //returns 'CAN'
If you would like locales to be returned with - instead of_ you can set an environment variable
CLM_MODE='INTL' in a .env file using dotenv just make sure that dotenv is required before requiring the country local map library
clm.getAlpha3ByAlpha2('CA'); //returns 'CAN'
clm.getLocaleByAlpha2('CA'); //returns 'en_CA'
clm.getCountryNameByAlpha2('CA'); //returns 'Canada'
clm.getNumericByAlpha2('CA'); //returns '124'
clm.getCurrencyByAlpha2('CA'); //returns 'CAD'
clm.getCountryByAlpha2('CA');
/*returns
{
"name":"Canada",
"alpha2":"CA",
"alpha3":"CAN",
"numeric":"124",
"locales":["en_CA","fr_CA"],
"default_locale":"en_CA",
"currency":"CAD",
"languages":["en","fr"],
"capital":"Ottawa",
"emoji":"🇨🇦",
"emojiU":"U+1F1E8 U+1F1E6",
"fips":"CA",
"internet":"CA",
"continent":"Americas",
"region":"North America"
}
*/
clm.getAlpha3ByAlpha2('CAN'); //returns 'CA'
clm.getLocaleByAlpha3('CAN'); //returns 'en_CA'
clm.getCountryNameByAlpha3('CAN'); //returns 'Canada'
clm.getNumericByAlpha3('CAN'); //returns '124'
clm.getCurrencyByAlpha3('CAN'); //returns 'CAD'
clm.getCountryByAlpha3('CAN');
/*returns
{
"name":"Canada",
"alpha2":"CA",
"alpha3":"CAN",
"numeric":"124",
"locales":["en_CA","fr_CA"],
"default_locale":"en_CA",
"currency":"CAD",
"languages":["en","fr"],
"capital":"Ottawa",
"emoji":"🇨🇦",
"emojiU":"U+1F1E8 U+1F1E6",
"fips":"CA",
"internet":"CA",
"continent":"Americas",
"region":"North America"
}
*/
clm.getAlpha2ByNumeric('123'); //returns 'CA'
clm.getAlpha3ByNumeric('124'); //returns 'CAN'
clm.getLocaleByNumeric('124'); //returns 'en_CA'
clm.getCountryNameByNumeric('124'); //returns 'Canada'
clm.getCurrencyByNumeric('124'); //returns 'CAD'
clm.getCountryByNumeric('124');
/*returns
{
"name":"Canada",
"alpha2":"CA",
"alpha3":"CAN",
"numeric":"124",
"locales":["en_CA","fr_CA"],
"default_locale":"en_CA",
"currency":"CAD",
"languages":["en","fr"],
"capital":"Ottawa",
"emoji":"🇨🇦",
"emojiU":"U+1F1E8 U+1F1E6",
"fips":"CA",
"internet":"CA",
"continent":"Americas",
"region":"North America"
}
*/
clm.getAlpha2ByName('Canada'); //returns 'CA'
clm.getAlpha3ByName('Canada'); //returns 'CAN'
clm.getLocaleByName('Canada'); //returns 'en_CA'
clm.getNumericByName('Canada'); //returns '124'
clm.getCurrencyByName('Canada'); //returns 'CAD'
clm.getCountryByName('Canada');
/*returns
{
"name":"Canada",
"alpha2":"CA",
"alpha3":"CAN",
"numeric":"124",
"locales":["en_CA","fr_CA"],
"default_locale":"en_CA",
"currency":"CAD",
"languages":["en","fr"],
"capital":"Ottawa",
"emoji":"🇨🇦",
"emojiU":"U+1F1E8 U+1F1E6",
"fips":"CA",
"internet":"CA",
"continent":"Americas",
"region":"North America"
}
*/
if true is passed for fuzzy these functions will do a fuzzy match if it can't find an exact match eg:
clm.getCountryByName('Candaa', true);
/*returns
{
"name":"Canada",
"alpha2":"CA",
"alpha3":"CAN",
"numeric":"124",
"locales":["en_CA","fr_CA"],
"default_locale":"en_CA",
"currency":"CAD",
"languages":["en","fr"],
"capital":"Ottawa",
"emoji":"🇨🇦",
"emojiU":"U+1F1E8 U+1F1E6",
"fips":"CA",
"internet":"CA",
"continent":"Americas",
"region":"North America"
}
*/
clm.getAllCountries();
/*returns
the list of all countries
*/
FAQs
Provides mapping between country codes and provides default country locales. Includes optional fuzzy matching for country name.
The npm package country-locale-map receives a total of 0 weekly downloads. As such, country-locale-map popularity was classified as not popular.
We found that country-locale-map demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.