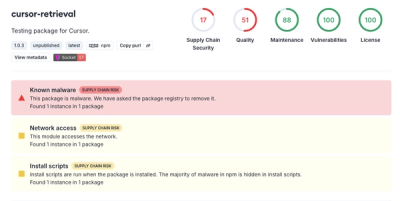
Security News
The Risks of Misguided Research in Supply Chain Security
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
countup.js
Advanced tools
countup.js is a lightweight JavaScript library that allows you to create animated counting numbers. It is useful for creating dynamic and engaging number displays in web applications, such as statistics, counters, and other numerical data visualizations.
Basic Count Up
This feature allows you to animate a number counting up from zero to a specified value. In this example, the number will count up to 1000 and display in the element with the ID 'targetElement'.
const countUp = new CountUp('targetElement', 1000);
if (!countUp.error) {
countUp.start();
} else {
console.error(countUp.error);
}
Custom Start and End Values
This feature allows you to specify custom start and end values for the count up animation. In this example, the number will count up from 500 to 2000.
const countUp = new CountUp('targetElement', 2000, { startVal: 500 });
if (!countUp.error) {
countUp.start();
} else {
console.error(countUp.error);
}
Formatting Options
This feature allows you to format the number with options such as decimal places, prefixes, and suffixes. In this example, the number will be formatted with two decimal places and display as currency (e.g., $1000.00 USD).
const countUp = new CountUp('targetElement', 1000, { decimalPlaces: 2, prefix: '$', suffix: ' USD' });
if (!countUp.error) {
countUp.start();
} else {
console.error(countUp.error);
}
Easing Functions
This feature allows you to apply custom easing functions to the count up animation. In this example, a quadratic easing function is used to create a smooth animation effect.
const countUp = new CountUp('targetElement', 1000, { easingFn: function (t, b, c, d) { return c * (t /= d) * t + b; } });
if (!countUp.error) {
countUp.start();
} else {
console.error(countUp.error);
}
Odometer is a smooth, themeable, and easy-to-use JavaScript library for transitioning numbers. It provides a more visually appealing way to animate numbers compared to countup.js, with various themes and styles that mimic the look of an odometer.
jquery.counterup is a jQuery plugin that animates a number from zero to a specified value. It is similar to countup.js but requires jQuery as a dependency, making it less lightweight. It is suitable for projects that already use jQuery.
CountUp.js is a dependency-free, lightweight Javascript class that can be used to quickly create animations that display numerical data in a more interesting way.
Despite its name, CountUp can count in either direction, depending on the start and end values that you pass.
CountUp.js supports all browsers. MIT license.
countUp.min.js
for modern browsers or countUp.withPolyfill.min.js
for IE9 and older, and Opera mini.CountUp is now distributed as a commonjs
module - see below for how to include it in your project.
On npm: countup.js
Params:
target: string | HTMLElement | HTMLInputElement
- id of html element, input, svg text element, or DOM element reference where counting occursendVal: number
- the value you want to arrive atoptions?: CountUpOptions
- optional configuration object for fine-grain controlOptions (defaults in parentheses):
interface CountUpOptions {
startVal?: number; // number to start at (0)
decimalPlaces?: number; // number of decimal places (0)
duration?: number; // animation duration in seconds (2)
useGrouping?: boolean; // example: 1,000 vs 1000 (true)
useEasing?: boolean; // ease animation (true)
smartEasingThreshold?: number; // smooth easing for large numbers above this if useEasing (999)
smartEasingAmount?: number; // amount to be eased for numbers above threshold (333)
separator?: string; // grouping separator (',')
decimal?: string; // decimal ('.')
// easingFn: easing function for animation (easeOutExpo)
easingFn?: (t: number, b: number, c: number, d: number) => number;
formattingFn?: (n: number) => string; // this function formats result
prefix?: string; // text prepended to result
suffix?: string; // text appended to result
numerals?: string[]; // numeral glyph substitution
}
const countUp = new CountUp('targetId', 5234);
if (!countUp.error) {
countUp.start();
} else {
console.error(countUp.error);
}
Pass options:
const countUp = new CountUp('targetId', 5234, options);
with optional callback:
countUp.start(someMethodToCallOnComplete);
// or an anonymous function
countUp.start(() => console.log('Complete!'));
Other methods:
Toggle pause/resume:
countUp.pauseResume();
Reset the animation:
countUp.reset();
Update the end value and animate:
countUp.update(989);
CountUp v2 is distributed as a commonjs
module which means you need to include CountUp in your project using a build tool such as Webpack or Browserify.
Example using Browserify
Install browserify and countup
npm i countup.js
npm i -g browserify
main.js:
var countUpModule = require('countup.js');
window.onload = function() {
var countUp = countUpModule.CountUp('target', 2000);
countUp.start();
}
Build your bundle
browserify main.js -o bundle.js
Include in your html:
<script src="bundle.js"></script>
🎉 Done!
Before you make a pull request, please be sure to follow these instructions:
src/countUp.ts
npm t
npm run build
, which copies and minifies the .js files to the dist
folder.npm run build:demo
then open index.html in a browser and make sure it works.FAQs
Animates a numerical value by counting to it
The npm package countup.js receives a total of 362,288 weekly downloads. As such, countup.js popularity was classified as popular.
We found that countup.js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.