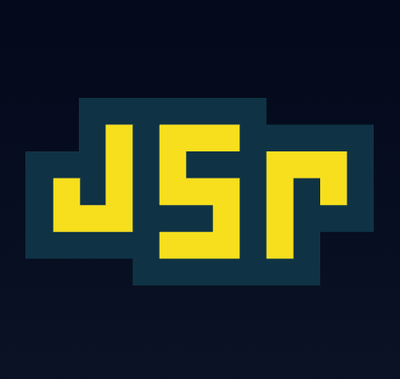
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
The cp-file npm package is a utility for copying files with support for progress reporting and other advanced features. It is designed to be a simple and efficient way to copy files in Node.js applications.
Basic File Copy
This feature allows you to copy a file from a source path to a destination path. The promise resolves when the file has been successfully copied.
const cpFile = require('cp-file');
cpFile('source.txt', 'destination.txt').then(() => {
console.log('File copied');
});
Progress Reporting
This feature provides progress reporting during the file copy process. You can listen to the 'progress' event to get updates on the copy progress.
const cpFile = require('cp-file');
cpFile('source.txt', 'destination.txt').on('progress', data => {
console.log(`Progress: ${data.percent * 100}%`);
}).then(() => {
console.log('File copied');
});
Preserve Timestamps
This feature allows you to preserve the original file timestamps when copying a file. This can be useful for maintaining file metadata.
const cpFile = require('cp-file');
cpFile('source.txt', 'destination.txt', { preserveTimestamps: true }).then(() => {
console.log('File copied with timestamps preserved');
});
Overwrite Option
This feature allows you to specify whether to overwrite the destination file if it already exists. By setting the 'overwrite' option to false, you can prevent accidental overwrites.
const cpFile = require('cp-file');
cpFile('source.txt', 'destination.txt', { overwrite: false }).then(() => {
console.log('File copied without overwriting existing files');
}).catch(error => {
console.error('File already exists');
});
fs-extra is a popular package that extends the native Node.js fs module with additional methods, including file copying. It provides similar functionality to cp-file but also includes many other file system utilities, making it a more comprehensive solution.
ncp (Node Copy) is another package for copying files and directories. It offers similar functionality to cp-file but is more focused on copying entire directories, making it a good choice for more complex copy operations.
cpy is a package for copying files with a focus on simplicity and performance. It offers similar features to cp-file, such as progress reporting and the ability to preserve timestamps, but is designed to be more lightweight and efficient.
Copy a file
fs.copyFileSync()
(when available) in the synchronous version.$ npm install cp-file
const cpFile = require('cp-file');
(async () => {
await cpFile('source/unicorn.png', 'destination/unicorn.png');
console.log('File copied');
})();
Returns a Promise
that resolves when the file is copied.
Type: string
File you want to copy.
Type: string
Where you want the file copied.
Type: Object
Type: boolean
Default: true
Overwrite existing file.
Progress reporting. Only available when using the async method.
Type: Function
{
src: string,
dest: string,
size: number,
written: number,
percent: number
}
src
and dest
are absolute paths.size
and written
are in bytes.percent
is a value between 0
and 1
.progress
event is emitted only once..on()
method is available only right after the initial cpFile()
call. So make sure
you add a handler
before .then()
:(async () => {
await cpFile(source, destination).on('progress', data => {
// …
});
})();
MIT © Sindre Sorhus
FAQs
Copy a file
The npm package cp-file receives a total of 1,729,741 weekly downloads. As such, cp-file popularity was classified as popular.
We found that cp-file demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.