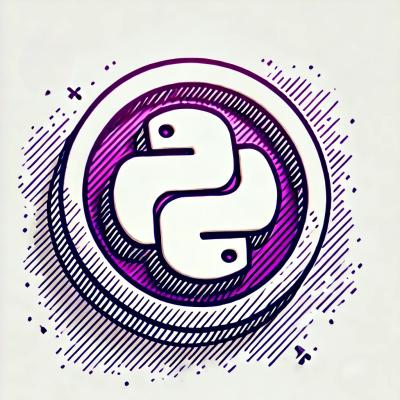
Security News
Python Overtakes JavaScript as Top Programming Language on GitHub
Python becomes GitHub's top language in 2024, driven by AI and data science projects, while AI-powered security tools are gaining adoption.
The croner npm package is a high-performance task scheduler for Node.js that allows you to run tasks at specific times or intervals. It is designed to be a drop-in replacement for the 'cron' package with additional features and improvements.
Simple cron job scheduling
This feature allows you to schedule a task to run at intervals defined by the cron syntax. In the provided code sample, a job is scheduled to run every 5 seconds.
const { Cron } = require('croner');
const job = Cron('*/5 * * * * *', () => {
console.log('This will run every 5 seconds');
});
One-time execution
This feature allows you to schedule a task to run once at a specific time in the future. The code sample schedules a job to run once after 10 seconds.
const { Cron } = require('croner');
const job = Cron(new Date(Date.now() + 10000), () => {
console.log('This will run once after 10 seconds');
});
Stopping a job
This feature allows you to stop a scheduled job. In the code sample, a job is scheduled to run every 5 seconds but is stopped after 15 seconds.
const { Cron } = require('croner');
const job = Cron('*/5 * * * * *', () => {
console.log('This job will be stopped.');
});
setTimeout(() => {
job.stop();
}, 15000);
Timezone support
This feature allows you to schedule jobs according to a specific timezone. The code sample schedules a job to run at 10:30 AM in the 'America/New_York' timezone.
const { Cron } = require('croner');
const job = Cron('0 30 10 * * *', () => {
console.log('This will run at 10:30 AM in the America/New_York timezone.');
}, { timezone: 'America/New_York' });
node-schedule is a flexible cron-like and not-cron-like job scheduler for Node.js. It allows you to schedule jobs using both cron-style and human-readable syntax. It is similar to croner but offers a different API and additional scheduling features like scheduling jobs with JavaScript Date objects.
agenda is a job scheduling library for Node.js that uses MongoDB for persisting job data. It is more suitable for distributed or long-running job tasks. Unlike croner, which focuses on in-memory cron job scheduling, agenda provides persistence and is better suited for applications that require job recovery and failover capabilities.
bull is a Redis-based queue system for Node.js. It is designed for handling distributed jobs and messages in Node.js applications. While croner is used for scheduling tasks, bull is more focused on job queuing, processing, and concurrency, making it suitable for more complex job handling scenarios.
Pure JavaScript minimal isomorphic cron parser and scheduler. Or simply speaking - setInterval on steroids.
Supports Node.js, requirejs, es-module and stand alone usage.
Documented with JSDoc for intellisense, and complete TypeScript typings for type checking.
<script src="https://cdn.jsdelivr.net/npm/croner@2/minified"></script>
// Run a function each second
Cron('* * * * * *', function () {
console.log('This will run every second');
});
npm install croner --save
// ESM Import
import Cron from "croner";
// ... or
// CommonJS Require
const Cron = require("croner");
To use as a UMD-module (stand alone, RequireJS etc.)
<script src="https://cdn.jsdelivr.net/npm/croner@2/minified"></script>
To use as a ES-module
<script type="module">
import Cron from "https://cdn.jsdelivr.net/npm/croner@2/minified";
// ... see usage section ...
</script>
... or a ES-module with import-map
<script type="importmap">
{
"imports": {
"croner": "https://cdn.jsdelivr.net/npm/croner@2/minified"
}
}
</script>
<script type="module">
import Cron from 'croner';
// ... see usage section ...
</script>
Cron('* * * * * *', () => {
console.log('This will run every second');
});
Include by CDN, or include croner.min.js in your preferred way, it will register itself as a require.js module.
define(["croner"], function(Cron) {
Cron('* * * * * *', function () {
console.log('This will run every second');
});
});
// Run a function each second
Cron('* * * * * *', function () {
console.log('This will run every second');
});
// Run a function every fifth second
Cron('*/5 * * * * *', function () {
console.log('This will run every fifth second');
});
// Run a function the first five seconds of a minute
Cron('0-4 * * * * *', function () {
console.log('This will run the first five seconds every minute');
});
// Run a function each second, limit to five runs
Cron('* * * * * *', { maxRuns: 5 }, function () {
console.log('This will run each second, but only five times.');
});
// Run a function each second, get reference to job
var job = Cron('* * * * * *', function () {
console.log('This will run each second.');
});
// Pause job
job.pause();
// Resume job
job.resume();
// Stop job
job.stop();
// Run every minute
var scheduler = Cron('0 * * * * *');
scheduler.schedule(function() {
console.log('This will run every minute');
});
// Run every minute
var scheduler = Cron('0 * * * * *');
// Schedule with options (all options are optional)
scheduler.schedule({ maxRuns: 5 }, function() {
console.log('This will run every minute.');
});
// Run every minute
var scheduler = Cron('0 * * * * *');
// Schedule with options (all options are optional)
var job = scheduler.schedule({ maxRuns: 5 }, function() {
console.log('This will run every minute.');
});
// Pause job
job.pause();
// Resume job
job.resume();
// Stop job
job.stop();
var o = Cron( <string pattern> [, <object options>] [, <function callback> ] );
// If Cron is initialized without a scheduled function, cron itself is returned
// and the following member functions is available.
o.next( [ <date previous> ] );
o.msToNext();
o.previous();
// If Cron is initialized _with_ a scheduled function, the job is retured instead.
// Otherwise you get a reference to the job when scheduling a new job.
var job = o.schedule( [ { startAt: <date>, stopAt: <date>, maxRuns: <integer> } ,] callback);
// These self-explanatory functions is available to control the job
job.pause();
job.resume();
job.stop();
┌──────────────── second (0 - 59)
│ ┌────────────── minute (0 - 59)
│ │ ┌──────────── hour (0 - 23)
│ │ │ ┌────────── day of month (1 - 31)
│ │ │ │ ┌──────── month (1 - 12)
│ │ │ │ │ ┌────── day of week (0 - 6)
│ │ │ │ │ │ (0 to 6 are Sunday to Saturday; 7 is Sunday, the same as 0)
│ │ │ │ │ │
* * * * * *
MIT
FAQs
Trigger functions and/or evaluate cron expressions in JavaScript. No dependencies. Most features. All environments.
The npm package croner receives a total of 1,570,922 weekly downloads. As such, croner popularity was classified as popular.
We found that croner demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Python becomes GitHub's top language in 2024, driven by AI and data science projects, while AI-powered security tools are gaining adoption.
Security News
Dutch National Police and FBI dismantle Redline and Meta infostealer malware-as-a-service operations in Operation Magnus, seizing servers and source code.
Research
Security News
Socket is tracking a new trend where malicious actors are now exploiting the popularity of LLM research to spread malware through seemingly useful open source packages.