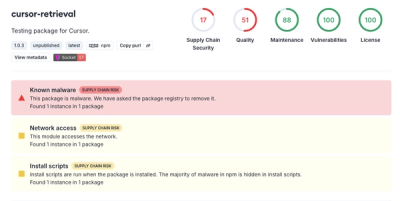
Security News
The Risks of Misguided Research in Supply Chain Security
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
cryptox is a node.js wrapper for REST API for multiple crypto currency exchanges.
cryptox manages API communication with different exchanges and provides common methods for all exchanges. Differences between the different API's are abstracted away.
npm install cryptox
var Cryptox = require("cryptox");
var account = new Cryptox("btce", {key: "your_key", secret: "your_secret"});
account.getOpenOrders({pair: "LTCUSD"}, function (err, openOrders) {
if (!err)
console.log(openOrders);
});
Example result:
{
"timestamp": "2015-02-03T00:03:27+00:00",
"error": "",
"data": [
{
"order_id": "563489985",
"pair": "LTCUSD",
"type": "buy",
"amount": 1,
"rate": 0.1,
"status": 0,
"created_at": "2015-02-01T19:23:15+00:00"
},
{
"order_id": "563612426",
"pair": "LTCUSD",
"type": "buy",
"amount": 2,
"rate": 0.5,
"status": 0,
"created_at": "2015-02-01T20:59:53+00:00"
}
]
}
if you are interested in extending cryptox for different exchange or a method not yet implemented, check out the document exchanges.md
|Bitfinex|Bitstamp |BitX|BTC-e|CEX.io|OXR<sup>1</sup>|
--- | :-: | :-: |:-: | :-: | :-: | :-: | getrate | | FI | FI | FI | | FI | getTicker | | FI | FI | FI | | — | getOrderBook | | FI | FI | FI | | — | getTrades | | | | | | — | getFee | | | | FI | | — | getTransactions| | | | | | — | getBalance | | | | | | — | getOpenOrders | | | | FI | | — | postSellOrder | | | | | | — | postBuyOrder | | | | | | — | cancelOrder | | | | | | — |
FI = Fully Implemented
FR = Fully Implemented, but restrictions apply (refer to notes below)
PI = Partially Implemented (refer to notes below)
— = Not Supported
1 OXR (Open Exchange Rates) is not a crypto exchange, however it provides exchange rates for world fiat currencies
cryptox module uses semver (http://semver.org/) for versioning: MAJOR.MINOR.PATCH.
- MAJOR version increments when non-backwards compatible API changes are introduced
- MINOR version increments when functionality in a backwards-compatible manner are introduced
- PATCH version increments when backwards-compatible bug fixes are made
IMPORTANT NOTE: Major version zero (0.y.z) is for initial development. Anything may change at any time. The public API should not be considered stable.
See detailed Changelog
Creates an instance of Cryptox
object type.
Cryptox(exchangeSlug [, options])
Depending on the parameters used, two types of objects can be created
var account = new Cryptox("btce", {key: "yourKey", secret: "yourSecret"});
var exchange = new Cryptox("btce");
exchangeSlug
is required and should have one values in table belowoptions
is used to pass parameters required for authentication, as indicated in table belowExchange name | exchangeSlug | Authentication |
---|---|---|
Bitstamp | "bitstamp" | key , secret , username |
BitX | "bitx" | key , secret |
BTC-e | "btce" | key , secret |
CEX.io | "cexio" | key , secret , username |
Open Exchange Rates | "oxr" | key |
options
should be used when calling methods that require authentication. Missing or incorrect key causes an error to be returned when calling a method that requires authentication (see Authentication).
getTicker(options, callback);
getTicker({pair: "BTCUSD"}, function (err, result) {
if (!err)
console.log(result);
});
options
is a JSON object containing parameters specific for each API callcallback
is executed inside the method once the API response is received from the exchangeThe arguments passed to the callback function for each method are:
err
is an error object or null
if no error occurred.
result
is JSON object containing the response.
Example result:
{
"timestamp": "2015-02-03T00:01:48+00:00",
"error": "",
"data": [
{
"pair": "BTCUSD",
"last": 272.064,
"bid": 272.064,
"ask": 273.395,
"volume": 7087.93047
}
]
}
| Type | Description
--- | --- | ---
timestamp
| string | ISO 8601 date & time
error
| string | error message or ""
if no error
data
| array | array of one or more JSON objects containig the API result
Following methods require authentication
Method | Requires authentication |
---|---|
getRate | 1 |
getTicker | |
getOrderBook | |
getTrades | |
getFee | x2 |
getTransactions | x |
getBalance | x |
getOpenOrders | x |
postSellOrder | x |
placeBuyOrder | x |
cancelOrder | x |
1 Open Exchange Rates (OXR) requires authentication
2 BTC-e does not require authentication forgetFee
(since the fee is fixed amount)
When calling a method that requires authentication, the object should have been constructed with parameters key
, secret
and (optional) userId
.
Returns the exchange rate.
getRate(options, callback);
exchange.getRate({pair: "EURUSD"}, function (err, rate) {
if (!err)
console.log(rate);
});
Example result:
{
"timestamp": "2015-02-04T20:01:09+00:00",
"error": "",
"data": [
{
"pair": "USDEUR",
"rate": 1.149807,
}
]
}
options
Parameter | Type | Required for | Description |
---|---|---|---|
pair | string | All | trading pair |
callback
see CallbacksParameter | Type | Description |
---|---|---|
pair | String | trading pair |
rate | Number | rate |
Returns the latest ticker indicators.
getTicker(options, callback);
exchange.getTicker({pair: "BTCUSD"}, function (err, ticker) {
if (!err)
console.log(ticker);
});
Example result:
{
"timestamp": "2015-02-03T00:01:48+00:00",
"error": "",
"data": [
{
"pair": "BTCUSD",
"last": 272.064,
"bid": 272.064,
"ask": 273.395,
"volume": 7087.93047
}
]
}
options
Parameter | Type | Required for | Description |
---|---|---|---|
pair | string | BTC-e | trading pair |
callback
see CallbacksParameter | Type | Description |
---|---|---|
pair | String | trading pair |
last | Number | last price |
bid | Number | highest buy order |
ask | Number | lowest sell order |
volume | Number | last 24 hours volume |
Optional response parameters
Parameter / Availability | Type | Description |
---|---|---|
high 1 | Number | last 24 hours price high |
low 1 | Number | last 24 hours price low |
vwap 1 | Number | last 24 hours volume weighted average price |
1 Bitstamp
Returns a list of bids and asks in the order book. Ask orders are sorted by price ascending. Bid orders are sorted by price descending. Note that multiple orders at the same price are not necessarily conflated.
cryptox.getOrderBook(options, callback);
exchange.getOrderBook({pair: "BTCUSD"}, function (err, orderBook) {
if (!err)
console.log(orderBook);
});
Example result:
{
"timestamp": ""2015-02-03T00:01:48+00:00"",
"error": "",
"data": [
{
"pair": "BTCUSD",
"asks": [[212.962,0.014],[213,1.46820994],[213.226,3.78630967]],
"bids": [[212.885,0.014],[212.827,0.00414864],[212.74,6.685]]
}
]
}
options
Parameter | Type | Required | Description |
---|---|---|---|
pair | string | All exchanges | trading pair |
callback
see CallbacksParameter | Type | Required | Description |
---|---|---|---|
timestamp | String | Yes | server time, ISO 8601 string |
error | Boolean | Yes | error message or "" if no error |
pair | String | Yes | trading pair |
asks | Array | Yes | list of asks in the order book |
bids | Array | Yes | list of bids in the order book |
Returns a list of the most recent trades.
getFee(options, callback);
Returns a fee, which is a float that represents the amount the exchange takes out of the orders. If an exchange has a fee of 0.2% this would be 0.002
.
account.getFee({pair: "BTCUSD"}, function (err, fee) {
if (!err)
console.log(fee);
});
Example result:
{
timestamp: "2015-02-01T20:59:53+00:00",
data: [
{
pair: "BTCUSD",
fee: 0.002
}
]
}
options
parameter is not used at the moment and can have any valuecallback
see CallbacksgetOpenOrders(options, callback);
Returns the open orders
account.getOpenOrders({pair: "LTCUSD"}, function (err, openOrders) {
if (!err)
console.log(openOrders);
});
Example result:
{
"timestamp": "2015-02-03T00:03:27+00:00",
"error": "",
"data": [
{
"orderId": "563489985",
"pair": "LTCUSD",
"type": "buy",
"amount": 1,
"rate": 0.1,
"status": 0,
"timestamp": "2015-02-01T19:23:15+00:00"
},
{
"orderId": "563612426",
"pair": "LTCUSD",
"type": "buy",
"amount": 2,
"rate": 0.5,
"status": 0,
"timestamp": "2015-02-01T20:59:53+00:00"
}
]
}
options
Parameter | Type | Required | Description |
---|---|---|---|
pair | string | No | trading pair |
callback
see CallbacksThe MIT License (MIT)
Copyright (c) 2015 Adrian Clinciu adrian.clinciu@outlook.com
Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
FAQs
Common API wrapper for multiple crypto currency exchanges
The npm package cryptox receives a total of 7 weekly downloads. As such, cryptox popularity was classified as not popular.
We found that cryptox demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.