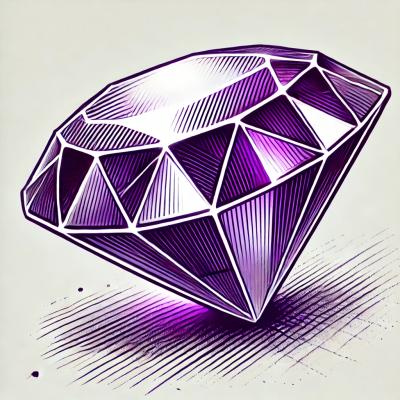
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
cspell is a spell checker for code that helps developers identify and correct spelling errors in their codebase, comments, strings, and documentation. It is highly configurable and can be integrated into various development workflows.
Basic Spell Checking
This command runs cspell to check for spelling errors in all TypeScript files within the 'src' directory and its subdirectories.
npx cspell 'src/**/*.ts'
Custom Dictionaries
You can add custom dictionaries to cspell by specifying them in the configuration file. This allows you to include domain-specific terms that are not in the default dictionary.
{
"dictionaries": ["custom-words"]
}
Ignoring Files and Patterns
cspell allows you to ignore specific files or patterns by adding them to the 'ignorePaths' array in the configuration file. This is useful for excluding generated files or dependencies.
{
"ignorePaths": ["node_modules/**", "dist/**"]
}
Configurable Language Settings
You can configure the language and locale settings in cspell to match the language used in your codebase. This ensures that the spell checker uses the correct dictionary and rules.
{
"language": "en",
"local": "en-US"
}
Integration with Code Editors
cspell can be integrated with popular code editors like VSCode. By enabling the cspell extension, you can get real-time spell checking as you write code.
/* Example for VSCode */
{
"cSpell.enabled": true
}
eslint-plugin-spellcheck is an ESLint plugin that checks for spelling errors in comments and string literals. It is less comprehensive than cspell but integrates directly with ESLint, making it a good choice for projects already using ESLint.
spellchecker is a simple spell checking library that can be used in Node.js applications. It provides basic spell checking functionality but lacks the extensive configuration options and integrations that cspell offers.
hunspell-spellchecker is a Node.js wrapper for the Hunspell spell checker. It provides powerful spell checking capabilities but requires more setup and configuration compared to cspell.
A Spell Checker for Code!
cspell
is a command line tool and library for spell checking code.
cspell was initially built as the spell checking service for the spell checker extension for Visual Studio Code.
npm install -g cspell
Example: recursively spell check all javascript files in src
cspell "src/**/*.js"
cspell --help
cspell needs Node 6 and above.
The concept is simple, split camelCase and snake_case words before checking them against a list of known words.
camelCase
-> camel case
HTMLInput
-> html input
srcCode
-> src code
snake_case_words
-> snake case words
camel2snake
-> camel snake
-- (the 2 is ignored)function parseJson(text: string)
-> function parse json text string
\n
, \t
are removed if the word does not match:
\narrow
-> narrow
- because narrow
is a word\ncode
-> code
- because ncode
is not a word.\network
-> network
- but it might be hiding a spelling error, if \n
was an escape character.english
which should be English
.It is possible to add spell check settings into your source code. This is to help with file specific issues that may not be applicable to the entire project.
All settings are prefixed with cSpell:
or spell-checker:
.
disable
-- turn off the spell checker for a section of code.enable
-- turn the spell checker back on after it has been turned off.ignore
-- specify a list of words to be ignored.words
-- specify a list of words to be considered correct and will appear in the suggestions list.ignoreRegExp
-- Any text matching the regular expression will NOT be checked for spelling.includeRegExp
-- Only text matching the collection of includeRegExp will be checked.enableCompoundWords
/ disableCompoundWords
-- Allow / disallow words like: "stringlength".It is possible to disable / enable the spell checker by adding comments to your code.
/* cSpell:disable */
/* spell-checker: disable */
/* spellchecker: disable */
/* cSpell:enable */
/* spell-checker: enable */
/* spellchecker: enable */
// cSpell:disable
const wackyWord = ['zaallano', 'wooorrdd', 'zzooommmmmmmm'];
/* cSpell:enable */
// Nest disable / enable is not Supported
// spell-checker:disable
// It is now disabled.
var liep = 1;
/* cspell:disable */
// It is still disabled
// cSpell:enable
// It is now enabled
const str = "goededag"; // <- will be flagged as an error.
// spell-checker:enable <- doesn't do anything
// cSPELL:DISABLE <-- also works.
// if there isn't an enable, spelling is disabled till the end of the file.
const str = "goedemorgen"; // <- will NOT be flagged as an error.
Ignore allows you the specify a list of words you want to ignore within the document.
// cSpell:ignore zaallano, wooorrdd
// cSpell:ignore zzooommmmmmmm
const wackyWord = ['zaallano', 'wooorrdd', 'zzooommmmmmmm'];
Note: words defined with ignore
will be ignored for the entire file.
The words list allows you to add words that will be considered correct and will be used as suggestions.
// cSpell:words woorxs sweeetbeat
const companyName = 'woorxs sweeetbeat';
Note: words defined with words
will be used for the entire file.
In some programing language it is common to glue words together.
// cSpell:enableCompoundWords
char * errormessage; // Is ok with cSpell:enableCompoundWords
int errornumber; // Is also ok.
Note: Compound word checking cannot be turned on / off in the same file. The last setting in the file determines the value for the entire file.
By default, the entire document is checked for spelling.
cSpell:disable
/cSpell:enable
above allows you to block off sections of the document.
ignoreRegExp
and includeRegExp
give you the ability to ignore or include patterns of text.
By default the flags gim
are added if no flags are given.
The spell checker works in the following way:
includeRegExp
excludeRegExp
// cSpell:ignoreRegExp 0x[0-9a-f]+ -- will ignore c style hex numbers
// cSpell:ignoreRegExp /0x[0-9A-F]+/g -- will ignore upper case c style hex numbers.
// cSpell:ignoreRegExp g{5} h{5} -- will only match ggggg, but not hhhhh or 'ggggg hhhhh'
// cSpell:ignoreRegExp g{5}|h{5} -- will match both ggggg and hhhhh
// cSpell:ignoreRegExp /g{5} h{5}/ -- will match 'ggggg hhhhh'
/* cSpell:ignoreRegExp /n{5}/ -- will NOT work as expected because of the ending comment -> */
/*
cSpell:ignoreRegExp /q{5}/ -- will match qqqqq just fine but NOT QQQQQ
*/
// cSpell:ignoreRegExp /[^\s]{40,}/ -- will ignore long strings with no spaces.
// cSpell:ignoreRegExp Email -- this will ignore email like patterns -- see Predefined RegExp expressions
var encodedImage = 'HR+cPzr7XGAOJNurPL0G8I2kU0UhKcqFssoKvFTR7z0T3VJfK37vS025uKroHfJ9nA6WWbHZ/ASn...';
var email1 = 'emailaddress@myfancynewcompany.com';
var email2 = '<emailaddress@myfancynewcompany.com>';
Note: ignoreRegExp and includeRegExp are applied to the entire file. They do not start and stop.
In general you should not need to use includeRegExp
. But if you are mixing languages then it could come in helpful.
# cSpell:includeRegExp #.*
# cSpell:includeRegExp ("""|''')[^\1]*\1
# only comments and block strings will be checked for spelling.
def sum_it(self, seq):
"""This is checked for spelling"""
variabele = 0
alinea = 'this is not checked'
for num in seq:
# The local state of 'value' will be retained between iterations
variabele += num
yield variabele
Urls
1 -- Matches urlsHexDigits
-- Matches hex digits: /^x?[0-1a-f]+$/i
HexValues
-- Matches common hex format like #aaa, 0xfeef, \u0134Base64
1 -- matches base64 blocks of text longer than 40 characters.Email
-- matches most email addresses.Everything
1 -- By default we match an entire document and remove the excludes.string
-- This matches common string formats like '...', "...", and `...`CStyleComment
-- These are C Style comments /* */ and //PhpHereDoc
-- This matches PHPHereDoc strings.1. These patterns are part of the default include/exclude list for every file.
cspell's behavior can be controlled through a config file. By default it looks for any of the following files:
cspell.json
.cspell.json
cSpell.json
Or you can specify a path to a config file with the --config <path>
argument on the command line.
// cSpell Settings
{
// Version of the setting file. Always 0.1
"version": "0.1",
// language - current active spelling language
"language": "en",
// words - list of words to be always considered correct
"words": [
"mkdirp",
"tsmerge",
"githubusercontent",
"streetsidesoftware",
"vsmarketplacebadge",
"visualstudio"
],
// flagWords - list of words to be always considered incorrect
// This is useful for offensive words and common spelling errors.
// For example "hte" should be "the"
"flagWords": [
"hte"
]
}
version
- currently always 0.1
language
- this specifies the language local to use in choosing the general dictionary.
For example: "language": "en-GB"
tells cspell to use British English instead of US English.
words
- a list of words to be considered correct.
flagWords
- a list of words to be allways considered incorrect
ignoreWords
- a list of words to be ignored (even if they are in the flagWords).
ignorePaths
- a list of globs to specify which files are to be ignored.
Example
"ignorePaths": ["node_modules/**"]
will cause cspell to ignore anything in the node_modules
directory.
maxNumberOfProblems
- defaults to 100 per file.
minWordLength
- defaults to 4 - the minimum length of a word before it is checked.
allowCompoundWords
- defaults to false; set to true to allow compound words by default.
dictionaries
- list of the names of the dictionaries to use. See Dictionaries below.
dictionaryDefinitions
- this list defines any custom dictionaries to use. This is how you can include other langauges like Spanish.
Example
"language": "en",
// Dictionaries "spanish", "ruby", and "corp-term" will always be checked.
// Including "spanish" in the list of dictionaries means both Spanish and English
// words will be considered correct.
"dictionaries": ["spanish", "ruby", "corp-terms", "fonts"],
// Define each dictionary. Relative paths are relative to the config file.
"dictionaryDefinitions": [
{ "name": "spanish", "path": "./spanish-words.txt"},
{ "name": "ruby", "path": "./ruby.txt"},
{ "name": "company-terms", "path": "./corp-terms.txt"}
],
ignoreRegExpList
- list of patterns to be ignored
includeRegExpList
- (Advanced) limits the text checked to be only that matching the expressions in the list.
patterns
- this allows you to define named patterns to be used with
ignoreRegExpList
and includeRegExpList
.
languageSettings
- this allow for per programming language configuration settings. See LanguageSettings
The spell checker includes a set of default dictionaries.
FAQs
A Spelling Checker for Code!
The npm package cspell receives a total of 412,953 weekly downloads. As such, cspell popularity was classified as popular.
We found that cspell demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.