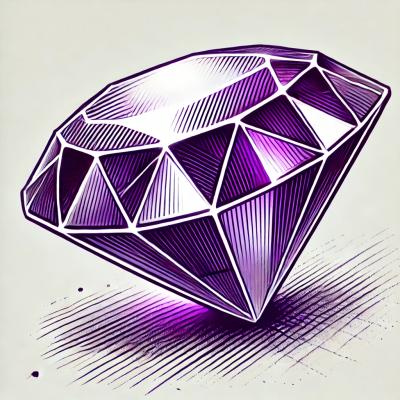
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
csv-writer
Advanced tools
The csv-writer npm package is a simple and powerful library for writing CSV files in Node.js. It provides an easy-to-use API for creating CSV files from arrays of objects or arrays of arrays, and it supports various customization options such as headers, field delimiters, and encoding.
Writing CSV from an array of objects
This feature allows you to write a CSV file from an array of objects. You can specify the path to the output file and define the headers for the CSV file. The records are written to the CSV file, and a promise is returned to indicate when the writing is complete.
const createCsvWriter = require('csv-writer').createObjectCsvWriter;
const csvWriter = createCsvWriter({
path: 'out.csv',
header: [
{id: 'name', title: 'NAME'},
{id: 'age', title: 'AGE'},
{id: 'address', title: 'ADDRESS'}
]
});
const records = [
{name: 'John Doe', age: 30, address: '123 Main St'},
{name: 'Jane Doe', age: 25, address: '456 Maple Ave'}
];
csvWriter.writeRecords(records) // returns a promise
.then(() => {
console.log('...Done');
});
Writing CSV from an array of arrays
This feature allows you to write a CSV file from an array of arrays. You can specify the path to the output file and define the headers for the CSV file. The records are written to the CSV file, and a promise is returned to indicate when the writing is complete.
const createCsvWriter = require('csv-writer').createArrayCsvWriter;
const csvWriter = createCsvWriter({
path: 'out.csv',
header: ['NAME', 'AGE', 'ADDRESS']
});
const records = [
['John Doe', 30, '123 Main St'],
['Jane Doe', 25, '456 Maple Ave']
];
csvWriter.writeRecords(records) // returns a promise
.then(() => {
console.log('...Done');
});
Customizing field delimiters
This feature allows you to customize the field delimiter used in the CSV file. In this example, the field delimiter is set to a semicolon (';'). The records are written to the CSV file with the specified delimiter, and a promise is returned to indicate when the writing is complete.
const createCsvWriter = require('csv-writer').createObjectCsvWriter;
const csvWriter = createCsvWriter({
path: 'out.csv',
header: [
{id: 'name', title: 'NAME'},
{id: 'age', title: 'AGE'},
{id: 'address', title: 'ADDRESS'}
],
fieldDelimiter: ';'
});
const records = [
{name: 'John Doe', age: 30, address: '123 Main St'},
{name: 'Jane Doe', age: 25, address: '456 Maple Ave'}
];
csvWriter.writeRecords(records) // returns a promise
.then(() => {
console.log('...Done');
});
fast-csv is a comprehensive library for parsing and formatting CSVs in Node.js. It offers a wide range of features including streaming, custom formatting, and support for various CSV dialects. Compared to csv-writer, fast-csv provides more advanced parsing capabilities and is suitable for handling large datasets efficiently.
csv-parser is a simple and efficient library for parsing CSV files in Node.js. It is designed to be fast and easy to use, with a focus on performance. While csv-parser is primarily focused on reading CSV files, it can be used in conjunction with other libraries to write CSV files. It is a good choice for applications that require high-speed CSV parsing.
papaparse is a powerful CSV parser that works in both Node.js and the browser. It supports a wide range of features including streaming, custom delimiters, and error handling. While papaparse is primarily known for its parsing capabilities, it also includes functionality for writing CSV files. It is a versatile library that can handle various CSV-related tasks.
Convert objects/arrays into a CSV string or write them into a file. It respects RFC 4180 for the output CSV format.
The example below shows how you can write records defined as the array of objects into a file.
const createCsvWriter = require('csv-writer').createObjectCsvWriter;
const csvWriter = createCsvWriter({
path: 'path/to/file.csv',
header: [
{id: 'name', title: 'NAME'},
{id: 'lang', title: 'LANGUAGE'}
]
});
const records = [
{name: 'Bob', lang: 'French, English'},
{name: 'Mary', lang: 'English'}
];
csvWriter.writeRecords(records) // returns a promise
.then(() => {
console.log('...Done');
});
// This will produce a file path/to/file.csv with following contents:
//
// NAME,LANGUAGE
// Bob,"French, English"
// Mary,English
You can keep writing records into the same file by calling writeRecords
multiple times
(but need to wait for the fulfillment of the promise
of the previous writeRecords
call).
Promise.resolve()
.then(() => csvWriter.writeRecords(records1))
.then(() => csvWriter.writeRecords(records2))
...
However, if you need to keep writing large data to a certain file, you would want to create
node's transform stream and use CsvStringifier
, which is explained later, inside it
, and pipe the stream into a file write stream.
If you don't want to write a header line, don't give title
to header elements and just give field IDs as a string.
const createCsvWriter = require('csv-writer').createObjectCsvWriter;
const csvWriter = createCsvWriter({
path: 'path/to/file.csv',
header: ['name', 'lang']
});
If each record is defined as an array, use createArrayCsvWriter
to get an csvWriter
.
const createCsvWriter = require('csv-writer').createArrayCsvWriter;
const csvWriter = createCsvWriter({
header: ['NAME', 'LANGUAGE'],
path: 'path/to/file.csv'
});
const records = [
['Bob', 'French, English'],
['Mary', 'English']
];
csvWriter.writeRecords(records) // returns a promise
.then(() => {
console.log('...Done');
});
// This will produce a file path/to/file.csv with following contents:
//
// NAME,LANGUAGE
// Bob,"French, English"
// Mary,English
If you just want to get a CSV string but don't want to write into a file,
you can use createObjectCsvStringifier
(or createArrayCsvStringifier
)
to get an csvStringifier
.
const createCsvStringifier = require('csv-writer').createObjectCsvStringifier;
const csvStringifier = createCsvStringifier({
header: [
{id: 'name', title: 'NAME'},
{id: 'lang', title: 'LANGUAGE'}
]
});
const records = [
{name: 'Bob', lang: 'French, English'},
{name: 'Mary', lang: 'English'}
];
console.log(csvStringifier.getHeaderString());
// => 'NAME,LANGUAGE\n'
console.log(csvStringifier.stringifyRecords(records));
// => 'Bob,"French, English"\nMary,English\n'
<Object>
path <string>
Path to a write file
header <Array<{id, title}|string>>
Array of objects (id
and title
properties) or strings (field IDs).
A header line will be written to the file only if given as an array of objects.
fieldDelimiter <string>
(optional)
Default: ,
. Only either comma ,
or semicolon ;
is allowed.
recordDelimiter <string>
(optional)
Default: \n
. Only either LF (\n
) or CRLF (\r\n
) is allowed.
encoding <string>
(optional)
Default: utf8
.
append <boolean>
(optional)
Default: false
. When true
, it will append CSV records to the specified file.
If the file doesn't exist, it will create one.
NOTE: A header line will not be written to the file if true
is given.
<CsvWriter>
<Object>
path <string>
Path to a write file
header <Array<string>>
(optional)
Array of field titles
fieldDelimiter <string>
(optional)
Default: ,
. Only either comma ,
or semicolon ;
is allowed.
recordDelimiter <string>
(optional)
Default: \n
. Only either LF (\n
) or CRLF (\r\n
) is allowed.
encoding <string>
(optional)
Default: utf8
.
append <boolean>
(optional)
Default: false
. When true
, it will append CSV records to the specified file.
If the file doesn't exist, it will create one.
NOTE: A header line will not be written to the file if true
is given.
<CsvWriter>
records <Iterator<Object|Array>>
Depending on which function was used to create a csvWriter
(i.e. createObjectCsvWriter
or createArrayCsvWriter
),
records will be either a collection of objects or arrays. As long as the collection is iterable, it doesn't need to be an array.
<Promise>
<Object>
header <Array<{id, title}|string>>
Array of objects (id
and title
properties) or strings (field IDs)
fieldDelimiter <string>
(optional)
Default: ,
. Only either comma ,
or semicolon ;
is allowed.
recordDelimiter <string>
(optional)
Default: \n
. Only either LF (\n
) or CRLF (\r\n
) is allowed.
<ObjectCsvStringifier>
<string>
<Array<Object>>
<string>
<Object>
header <Array<string>>
(optional)
Array of field titles
fieldDelimiter <string>
(optional)
Default: ,
. Only either comma ,
or semicolon ;
is allowed.
recordDelimiter <string>
(optional)
Default: \n
. Only either LF (\n
) or CRLF (\r\n
) is allowed.
<ArrayCsvStringifier>
<string>
<Array<Array<string>>>
<string>
Feature requests and bug reports are very welcome: https://github.com/ryu1kn/csv-writer/issues
A couple of requests from me when you raise an issue on GitHub.
FAQs
Convert objects/arrays into a CSV string or write them into a CSV file
The npm package csv-writer receives a total of 370,897 weekly downloads. As such, csv-writer popularity was classified as popular.
We found that csv-writer demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.