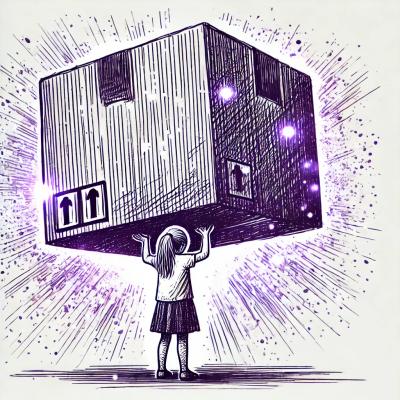
Security News
The Unpaid Backbone of Open Source: Solo Maintainers Face Increasing Security Demands
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
A curry function without anything too clever (... because hunger is the finest spice)
If you don't know currying, or aren't sold on it's awesomeness, perhaps a friendly blog post will help.
var curry = require('curry');
//-- creating a curried function is pretty
//-- straight forward:
var add = curry(function(a, b){ return a + b });
//-- it can be called like normal:
add(1, 2) //= 3
//-- or, if you miss off any arguments,
//-- a new funtion that expects all (or some) of
//-- the remaining arguments will be created:
var add1 = add(1);
add1(2) //= 3;
//-- curry knows how many arguments a function should take
//-- by the number of parameters in the parameter list
//-- in this case, a function and two arrays is expected
//-- (fn, a, b). zipWith will combine two arrays using a function:
var zipWith = curry(function(fn, a, b){
return a.map(function(val, i){ return fn(val, b[i]) });
});
//-- if there are still more arguments required, a curried function
//-- will always return a new curried function:
var zipAdd = zipWith(add);
var zipAddWith123 = zipAdd([1, 2, 3]);
//-- both functions are usable as you'd expect at any time:
zipAdd([1, 2, 3], [1, 2, 3]); //= [2, 4, 6]
zipAddWith123([5, 6, 7]); //= [6, 8, 10]
//-- the number of arguments a function is expected to provide
//-- can be discovered by the .length property
zipWith.length; //= 3
zipAdd.length; //= 2
zipAddWith123.length; //= 1
Sometimes it's necessary (especially when wrapping variadic functions) to explicitly provide an arity for your curried function:
var sum = function(){
var nums = [].slice.call(arguments);
return nums.reduce(function(a, b){ return a + b });
}
var sum3 = curry.to(3, sum);
var sum4 = curry.to(4, sum);
sum3(1, 2)(3) //= 6
sum4(1)(2)(3, 4) //= 10
It's a (sad?) fact that JavaScript functions are often written to take the 'context' object as the first argument.
With curried functions, of course, we want it to be the last object. curry.adapt
shifts the context to the last argument,
to give us a hand with this:
var delve = require('delve');
var delveC = curry.adapt(delve);
var getDataFromResponse = delveC('response.body.data');
getDataFromResponse({ response: { body: { data: { x: 2 }} } }); //= { x: 2 }
Like curry.adapt
, but the arity explicitly provided:
var _ = require('lodash');
var map = curry.adaptTo(2, _.map);
var mapInc = map(function(a){ return a + 1 })
mapInc([1, 2, 3]) //= [2, 3, 4]
npm install curry
define(['libs/curry.min'], function(curry){
//-- assuming libs/curry.min.js is the downloaded minified version from this repo,
//-- curry will be available here
});
If you're not using tools like browserify or require.js, you can load curry globally:
<script src="libs/curry.min.js"></script>
<script>
<!-- curry available here -->
</script>
∏∏
FAQs
flexible but simple curry function
The npm package curry receives a total of 7,986 weekly downloads. As such, curry popularity was classified as popular.
We found that curry demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
Security News
License exceptions modify the terms of open source licenses, impacting how software can be used, modified, and distributed. Developers should be aware of the legal implications of these exceptions.
Security News
A developer is accusing Tencent of violating the GPL by modifying a Python utility and changing its license to BSD, highlighting the importance of copyleft compliance.