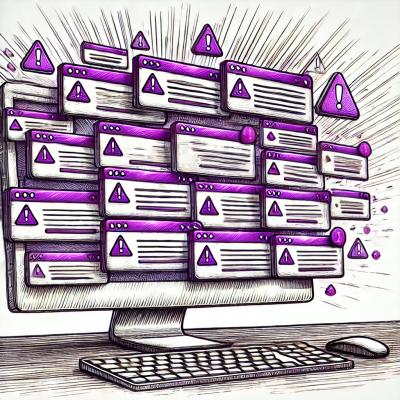
Security News
Combatting Alert Fatigue by Prioritizing Malicious Intent
In 2023, data breaches surged 78% from zero-day and supply chain attacks, but developers are still buried under alerts that are unable to prevent these threats.
The cwebp-bin npm package provides a Node.js wrapper for the cwebp command-line tool, which is used to convert images to the WebP format. This package allows developers to easily integrate WebP conversion into their Node.js applications.
Convert JPEG to WebP
This feature allows you to convert a JPEG image to WebP format using the cwebp command-line tool. The code sample demonstrates how to use the execFile function from the child_process module to execute the cwebp command with the input and output file paths.
const cwebp = require('cwebp-bin');
const { execFile } = require('child_process');
execFile(cwebp, ['input.jpg', '-o', 'output.webp'], err => {
if (err) {
throw err;
}
console.log('Image converted to WebP format');
});
Convert PNG to WebP
This feature allows you to convert a PNG image to WebP format using the cwebp command-line tool. The code sample demonstrates how to use the execFile function from the child_process module to execute the cwebp command with the input and output file paths.
const cwebp = require('cwebp-bin');
const { execFile } = require('child_process');
execFile(cwebp, ['input.png', '-o', 'output.webp'], err => {
if (err) {
throw err;
}
console.log('Image converted to WebP format');
});
Set WebP Quality
This feature allows you to set the quality of the output WebP image. The code sample demonstrates how to use the '-q' option with the cwebp command to specify the quality level (in this case, 80) for the output WebP image.
const cwebp = require('cwebp-bin');
const { execFile } = require('child_process');
execFile(cwebp, ['input.jpg', '-q', '80', '-o', 'output.webp'], err => {
if (err) {
throw err;
}
console.log('Image converted to WebP format with quality 80');
});
The imagemin-webp package is a plugin for the imagemin image optimization tool that allows you to convert images to the WebP format. It provides a higher-level API compared to cwebp-bin and integrates seamlessly with the imagemin ecosystem, making it easier to use in build processes and workflows.
The sharp package is a high-performance image processing library for Node.js that supports a wide range of image formats, including WebP. It provides a rich set of features for image manipulation, such as resizing, cropping, and format conversion. Compared to cwebp-bin, sharp offers more comprehensive image processing capabilities and a more user-friendly API.
The webp-converter package is a Node.js wrapper for the WebP conversion tools, similar to cwebp-bin. It provides functions to convert images to and from the WebP format. While it offers similar functionality to cwebp-bin, it also includes additional features such as batch conversion and support for animated WebP images.
![]() | ![]() |
---|---|
8bit converted png (14,225 bytes) | WebP converted (14,924 bytes) |
This case, 8-bit converted PNG image is smaller than WebP one,
Of course, WebP images are smaller than 24-bit PNG images.
But if PNG (event though 8-bit converted!) contains many colors, it depends.
![]() | ![]() |
---|---|
JPEGMini optimized jpg (39,335 bytes) | WebP converted (24,300 bytes) |
In most cases, WebP images are smaller than JPEG images.
WebP requires following libraries on Linux. See detail.
$ sudo apt-get install libjpeg-dev libpng-dev libtiff-dev libgif-dev
$ npm install --save cwebp-bin
$ cwebp input.png -o output.webp
var execFile = require('child_process').execFile;
var cwebp = require('cwebp-bin').path;
execFile(cwebp, ['input.png', '-o', 'output.webp'], function (error) {
if (error) {
throw error;
}
console.log('Image was converted');
});
Usage:
cwebp [-preset <...>] [options] in_file [-o out_file]
If input size (-s) for an image is not specified, it is assumed to be a PNG, JPEG or TIFF file.
options:
-h / -help ............ short help
-H / -longhelp ........ long help
-q <float> ............. quality factor (0:small..100:big)
-alpha_q <int> ......... Transparency-compression quality (0..100).
-preset <string> ....... Preset setting, one of:
default, photo, picture,
drawing, icon, text
-preset must come first, as it overwrites other parameters. -z <int> ............... Activates lossless preset with given level in [0:fast, ..., 9:slowest]
-m <int> ............... compression method (0=fast, 6=slowest)
-segments <int> ........ number of segments to use (1..4)
-size <int> ............ Target size (in bytes)
-psnr <float> .......... Target PSNR (in dB. typically: 42)
-s <int> <int> ......... Input size (width x height) for YUV
-sns <int> ............. Spatial Noise Shaping (0:off, 100:max)
-f <int> ............... filter strength (0=off..100)
-sharpness <int> ....... filter sharpness (0:most .. 7:least sharp)
-strong ................ use strong filter instead of simple (default).
-nostrong .............. use simple filter instead of strong.
-partition_limit <int> . limit quality to fit the 512k limit on
the first partition (0=no degradation ... 100=full)
-pass <int> ............ analysis pass number (1..10)
-crop <x> <y> <w> <h> .. crop picture with the given rectangle
-resize <w> <h> ........ resize picture (after any cropping)
-mt .................... use multi-threading if available
-low_memory ............ reduce memory usage (slower encoding)
-map <int> ............. print map of extra info.
-print_psnr ............ prints averaged PSNR distortion.
-print_ssim ............ prints averaged SSIM distortion.
-print_lsim ............ prints local-similarity distortion.
-d <file.pgm> .......... dump the compressed output (PGM file).
-alpha_method <int> .... Transparency-compression method (0..1)
-alpha_filter <string> . predictive filtering for alpha plane.
One of: none, fast (default) or best.
-alpha_cleanup ......... Clean RGB values in transparent area.
-blend_alpha <hex> ..... Blend colors against background color
expressed as RGB values written in
hexadecimal, e.g. 0xc0e0d0 for red=0xc0
green=0xe0 and blue=0xd0.
-noalpha ............... discard any transparency information.
-lossless .............. Encode image losslessly.
-hint <string> ......... Specify image characteristics hint.
One of: photo, picture or graph
-metadata <string> ..... comma separated list of metadata to
copy from the input to the output if present.
Valid values: all, none (default), exif, icc, xmp
-short ................. condense printed message
-quiet ................. don't print anything.
-version ............... print version number and exit.
-noasm ................. disable all assembly optimizations.
-v ..................... verbose, e.g. print encoding/decoding times
-progress .............. report encoding progress
Experimental Options:
-jpeg_like ............. Roughly match expected JPEG size.
-af .................... auto-adjust filter strength.
-pre <int> ............. pre-processing filter
This is licensed under BSD.
WebP is licensed under Creative Commons Attribution 3.0 License.
FAQs
cwebp wrapper that makes it seamlessly available as a local dependency
The npm package cwebp-bin receives a total of 130,089 weekly downloads. As such, cwebp-bin popularity was classified as popular.
We found that cwebp-bin demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
In 2023, data breaches surged 78% from zero-day and supply chain attacks, but developers are still buried under alerts that are unable to prevent these threats.
Security News
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
Security News
License exceptions modify the terms of open source licenses, impacting how software can be used, modified, and distributed. Developers should be aware of the legal implications of these exceptions.