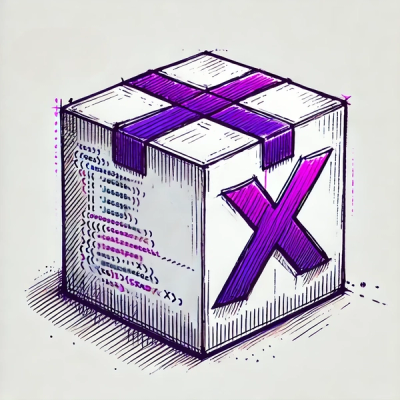
Security News
pnpm 10.0.0 Blocks Lifecycle Scripts by Default
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
The cyclist npm package is a utility for managing a fixed size cyclic list, also known as a ring buffer. It is particularly useful in scenarios where a fixed number of items need to be stored and managed efficiently, such as in systems or applications where memory management is critical and older entries are overwritten by new ones once the buffer is full.
Create and manage a cyclic list
This feature allows the creation of a cyclic list with a specified size. You can add items to the list at specific indices and retrieve them. When the index is reused, the old value is overwritten with the new value.
const Cyclist = require('cyclist');
const buffer = new Cyclist(3);
buffer.put(0, 'a');
buffer.put(1, 'b');
buffer.put(2, 'c');
console.log(buffer.get(1)); // Output: 'b'
buffer.put(1, 'd');
console.log(buffer.get(1)); // Output: 'd'
RingBufferJS is another npm package that provides similar functionality to cyclist. It allows for the creation and management of ring buffers. Compared to cyclist, RingBufferJS might offer different methods for interacting with the buffer, but the core concept of a fixed-size, overwritable buffer remains the same.
Circular-buffer is an npm package that also implements a circular buffer mechanism. It provides functionality similar to cyclist but may include additional features such as different methods for buffer manipulation and retrieval. It's useful for comparing how different packages implement similar data structures.
Cyclist is an efficient cyclic list implemention for Javascript. It is available through npm
npm install cyclist
Cyclist allows you to create a list of fixed size that is cyclic. In a cyclist list the element following the last one is the first one. This property can be really useful when for example trying to order data packets that can arrive out of order over a network stream.
var cyclist = require('cyclist')
var list = cyclist(4)
list.put(42, 'hello 42') // store something and index 42
list.put(43, 'hello 43') // store something and index 43
console.log(list.get(42)) // prints hello 42
console.log(list.get(46)) // prints hello 42 again since 46 - 42 == list.size
cyclist(size)
creates a new buffercyclist#get(index)
get an object stored in the buffercyclist#put(index,value)
insert an object into the buffercyclist#del(index)
delete an object from an indexcyclist#size
property containing current size of bufferMIT
FAQs
Cyclist is an efficient cyclic list implemention.
The npm package cyclist receives a total of 2,401,628 weekly downloads. As such, cyclist popularity was classified as popular.
We found that cyclist demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.