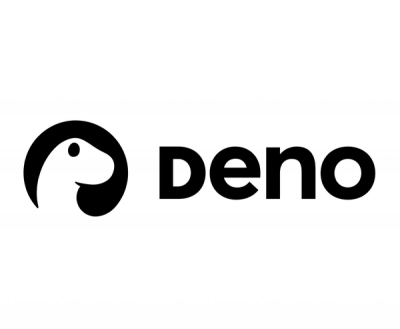
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
The d3-brush module is part of the D3.js library and provides tools for creating interactive brushing and linking in visualizations. Brushing allows users to select a region of a chart or graph, which can then be used to highlight or filter data.
Basic Brush
This code sets up a basic brush on an SVG element. When the user interacts with the brush, the 'brushed' function is called, logging the selected region.
const svg = d3.select('svg');
const brush = d3.brush().on('brush', brushed);
svg.append('g').attr('class', 'brush').call(brush);
function brushed(event) {
const selection = event.selection;
console.log(selection);
}
Brush with Custom Handle
This code demonstrates how to create a brush with a custom handle size. The handle size is set to 10 pixels.
const svg = d3.select('svg');
const brush = d3.brush().handleSize(10).on('brush', brushed);
svg.append('g').attr('class', 'brush').call(brush);
function brushed(event) {
const selection = event.selection;
console.log(selection);
}
Brush with Extent
This code sets up a brush with a specified extent, limiting the brushable area to a 400x400 pixel region.
const svg = d3.select('svg');
const brush = d3.brush().extent([[0, 0], [400, 400]]).on('brush', brushed);
svg.append('g').attr('class', 'brush').call(brush);
function brushed(event) {
const selection = event.selection;
console.log(selection);
}
The d3-selection module is used for selecting and manipulating DOM elements. While it does not provide brushing functionality, it is often used in conjunction with d3-brush to handle DOM selections and updates.
The d3-zoom module provides tools for enabling zooming and panning on visualizations. It can be used alongside d3-brush to create more interactive and dynamic visualizations.
Crossfilter is a JavaScript library for exploring large multivariate datasets in the browser. It provides filtering and grouping functionalities that can complement the brushing capabilities of d3-brush.
FAQs
Select a one- or two-dimensional region using the mouse or touch.
The npm package d3-brush receives a total of 1,952,514 weekly downloads. As such, d3-brush popularity was classified as popular.
We found that d3-brush demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.