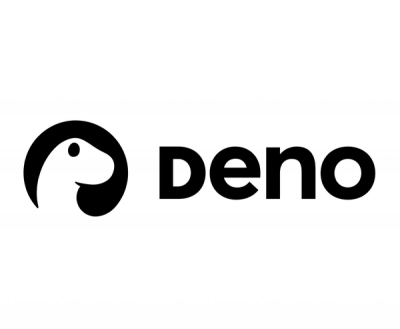
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
d3-geo-projection
Advanced tools
The d3-geo-projection npm package is an extension of the D3.js library that provides a variety of geographic projections. It allows you to transform geographic coordinates into a variety of different map projections, which can be used for data visualization, cartography, and geographic analysis.
Azimuthal Equidistant Projection
This feature allows you to create an Azimuthal Equidistant projection, which is useful for showing distances from a central point.
const d3 = require('d3-geo-projection');
const projection = d3.geoAzimuthalEquidistant();
const coordinates = projection([0, 0]);
console.log(coordinates);
Mercator Projection
This feature allows you to create a Mercator projection, which is commonly used for navigation maps.
const d3 = require('d3-geo-projection');
const projection = d3.geoMercator();
const coordinates = projection([0, 0]);
console.log(coordinates);
Orthographic Projection
This feature allows you to create an Orthographic projection, which is useful for creating a globe-like view of the Earth.
const d3 = require('d3-geo-projection');
const projection = d3.geoOrthographic();
const coordinates = projection([0, 0]);
console.log(coordinates);
Proj4 is a library for performing cartographic transformations. It supports a wide range of projections and is highly configurable. Compared to d3-geo-projection, proj4 is more focused on the mathematical transformations and less on the visualization aspects.
Leaflet is a popular open-source JavaScript library for mobile-friendly interactive maps. While it does not provide as many projection options as d3-geo-projection, it is highly effective for creating interactive maps with a variety of base layers and overlays.
Mapbox GL JS is a JavaScript library that uses WebGL to render interactive maps from vector tiles and Mapbox styles. It offers fewer projection options compared to d3-geo-projection but excels in rendering performance and interactive features.
Projections:
This plugin also provides d3.geo.interrupt, which can be used to create arbitrary interrupted projections from a given raw projection. For example, see Philbrick’s interrupted Sinu-Mollweide.
This plugin requires D3 3.0 or greater. To use the official hosted version, include the projection plugin after including D3:
<script src="http://d3js.org/d3.v3.min.js" charset="utf-8"></script>
<script src="http://d3js.org/d3.geo.projection.v0.min.js" charset="utf-8"></script>
Alternatively, you can clone this repo, download the zipball, or right-click d3.geo.projection.v0.min.js and save.
To use this plugin within a Node.js context, you can npm install d3 d3-geo-projection
and then say:
var d3 = require("d3");
require("d3-geo-projection")(d3);
Subsequently, d3.geo
will contain all the extended projections.
First define your raw projection function:
function cosinusoidal(λ, φ) {
return [λ * Math.sin(φ), φ];
}
cosinusoidal.invert = function(x, y) {
return [x / Math.sin(y), y];
};
Then create a constructor using d3.geo.projection:
d3.geo.cosinusoidal = function() {
return d3.geo.projection(cosinusoidal);
};
You can optionally expose the raw projection to facilitate composite projections:
(d3.geo.cosinusoidal = function() {
return d3.geo.projection(cosinusoidal);
}).raw = cosinusoidal;
FAQs
Extended geographic projections for d3-geo.
The npm package d3-geo-projection receives a total of 358,424 weekly downloads. As such, d3-geo-projection popularity was classified as popular.
We found that d3-geo-projection demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.