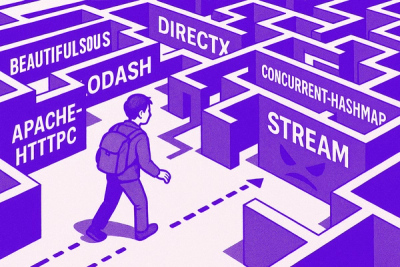
Research
NPM targeted by malware campaign mimicking familiar library names
Socket uncovered npm malware campaign mimicking popular Node.js libraries and packages from other ecosystems; packages steal data and execute remote code.
d3-interpolate-path
Advanced tools
Interpolates path `d` attribute smoothly when A and B have different number of points.
The d3-interpolate-path npm package is a utility for interpolating between two SVG path data strings. It is particularly useful for creating smooth transitions and animations between different shapes in data visualizations.
Basic Path Interpolation
This feature allows you to interpolate between two SVG path data strings. The code sample demonstrates how to create an interpolator and use it to find a path that is halfway between two given paths.
const interpolatePath = require('d3-interpolate-path').interpolatePath;
const path1 = 'M10,10L90,10L90,90L10,90Z';
const path2 = 'M20,20L80,20L80,80L20,80Z';
const interpolator = interpolatePath(path1, path2);
console.log(interpolator(0.5)); // Outputs a path halfway between path1 and path2
Animating Path Transitions
This feature allows you to animate transitions between two SVG paths. The code sample demonstrates how to use D3.js to create a smooth transition between two paths over a duration of 1000 milliseconds.
const d3 = require('d3');
const interpolatePath = require('d3-interpolate-path').interpolatePath;
const path1 = 'M10,10L90,10L90,90L10,90Z';
const path2 = 'M20,20L80,20L80,80L20,80Z';
const interpolator = interpolatePath(path1, path2);
d3.select('path')
.transition()
.duration(1000)
.attrTween('d', function() {
return function(t) {
return interpolator(t);
};
});
Flubber is a library for interpolating between shapes in a way that looks natural and smooth. It supports more complex shape morphing compared to d3-interpolate-path and provides additional utilities for handling shape transitions.
svg-path-interpolator is a package that provides utilities for interpolating between SVG path data. It is similar to d3-interpolate-path but offers a different API and additional customization options for path interpolation.
d3-interpolate-path is a zero-dependency that adds an interpolator optimized for SVG <path> elements. It can also work directly with object representations of path commands that can be later interpreted for use with canvas or WebGL.
Note this package no longer has a dependency on d3 or d3-interpolate, but in web usage adds itself to the d3 namespace like other d3 plugins do.
Blog: Improving D3 Path Animation
Demo: https://pbeshai.github.io/d3-interpolate-path/
var line = d3.line()
.curve(d3.curveLinear)
.x(function (d) { return x(d.x); })
.y(function (d) { return y(d.y); });
d3.select('path.my-path')
.transition()
.duration(2000)
.attrTween('d', function (d) {
var previous = d3.select(this).attr('d');
var current = line(d);
return d3.interpolatePath(previous, current);
});
If you're using it in a module environment, you can import it as follows:
import { interpolatePath } from 'd3-interpolate-path';
Otherwise, you can use it via a <script>
tag as follows:
<script src="https://unpkg.com/d3-interpolate-path/build/d3-interpolate-path.min.js"></script>
Get rollup watching for changes and rebuilding
npm run watch
Run a web server in the docs directory
cd docs
php -S localhost:8000
Go to http://localhost:8000
If you use NPM, npm install d3-interpolate-path
. Otherwise, download the latest release.
# interpolatePath(a, b, excludeSegment)
Returns an interpolator between two path attribute d
strings a and b. The interpolator extends a and b to have the same number of points before applying linear interpolation on the values. It uses De Castlejau's algorithm for handling bezier curves.
var pathInterpolator = interpolatePath('M0,0 L10,10', 'M10,10 L20,20 L30,30')
pathInterpolator(0) // 'M0,0 L10,10 L10,10'
pathInterpolator(0.5) // 'M5,5 L15,15 L20,20'
pathInterpolator(1) // 'M10,10 L20,20 L30,30'
You can optionally provide a function excludeSegment that takes two adjacent path commands and returns true if that segment should be excluded when splitting the line. A command object has form { type, x, y }
(with possibly more attributes depending on type). An example object:
// equivalent to M0,150 in a path `d` string
{
type: 'M',
x: 0,
y: 150
}
This is most useful when working with d3-area. Excluding the final segment (i.e. the vertical line at the end) from being split ensures a nice transition. If you know that highest x
value in the path, you can exclude the final segment by passing an excludeSegment function similar to:
function excludeSegment(a, b) {
return a.x === b.x && a.x === 300; // here 300 is the max X
}
# interpolatePathCommands(aCommands, bCommands, excludeSegment)
Returns an interpolator between two paths defined as arrays of command objects a and b. The interpolator extends a and b to have the same number of points if they differ. This can be useful if you want to work with paths in other formats besides SVG (e.g. canvas or WebGL).
Command objects take the following form:
| { type: 'M', x: number, y: number },
| { type: 'L', x, y }
| { type: 'H', x }
| { type: 'V', y }
| { type: 'C', x1, y1, x2, y2, x, y }
| { type: 'S', x2, y2, x, y }
| { type: 'Q', x1, y1, x, y }
| { type: 'T', x, y }
| { type: 'A', rx, ry, xAxisRotation, largeArcFlag, sweepFlag, x, y }
| { type: 'Z' }
Example usage:
const a = [
{ type: 'M', x: 0, y: 0 },
{ type: 'L', x: 10, y: 10 },
];
const b = [
{ type: 'M', x: 10, y: 10 },
{ type: 'L', x: 20, y: 20 },
{ type: 'L', x: 200, y: 200 },
];
const interpolator = interpolatePathCommands(a, b);
> interpolator(0);
[
{ type: 'M', x: 0, y: 0 },
{ type: 'L', x: 5, y: 5 },
{ type: 'L', x: 10, y: 10 },
]
> interpolator(0.5);
[
{ type: 'M', x: 5, y: 5 },
{ type: 'L', x: 12.5, y: 12.5 },
{ type: 'L', x: 105, y: 105 },
]
# pathCommandsFromString(pathDString)
Converts a path d
string into an array of path command objects to work with interpolatePathCommands.
Example usage:
const a = 'M0,0L10,10';
> pathCommandsFromString(a)
[
{ type: 'M', x: 0, y: 0 },
{ type: 'L', x: 10, y: 10 },
]
FAQs
Interpolates path `d` attribute smoothly when A and B have different number of points.
The npm package d3-interpolate-path receives a total of 291,859 weekly downloads. As such, d3-interpolate-path popularity was classified as popular.
We found that d3-interpolate-path demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Socket uncovered npm malware campaign mimicking popular Node.js libraries and packages from other ecosystems; packages steal data and execute remote code.
Research
Socket's research uncovers three dangerous Go modules that contain obfuscated disk-wiping malware, threatening complete data loss.
Research
Socket uncovers malicious packages on PyPI using Gmail's SMTP protocol for command and control (C2) to exfiltrate data and execute commands.